In this Typescript tutorial, I have explained how to convert date to string format dd/mm/yyyy in Typescript using various methods with complete code.
To convert date to string format dd/mm/yyyy in Typescript, you can use various methods. One of the popular methods is by using toLocaleDateString like: function formatDateToDDMMYYYY(date: Date): string {return date.toLocaleDateString(‘en-GB’);}
Typescript convert date to string format dd/mm/yyyy
Let us discuss three different methods to convert date to string format dd/mm/yyyy in Typescript.
Convert date to string format dd/mm/yyyy using toLocaleDateString method
The toLocaleDateString method is a straightforward way to format a date in TypeScript. It converts a date to a string using the locale and options we specify. To format a date as DD/MM/YYYY, we can pass the appropriate options to this method. Here is the complete code.
function formatDateToDDMMYYYY(date: Date): string {
return date.toLocaleDateString('en-GB');
}
const currentDate = new Date();
console.log(formatDateToDDMMYYYY(currentDate));
Once you run the code using any Typescript code editor, you can see the output below:
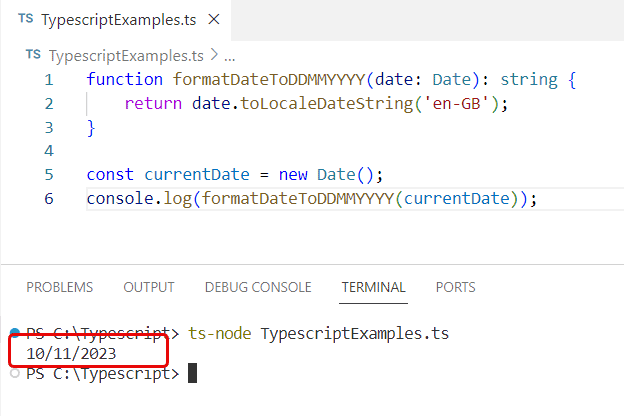
Convert date to string format dd/mm/yyyy using manual formatting
In Typescript, you can also extract the day, month, and year from the date, and then you can format it according to your requirements.
function formatDateManually(date: Date): string {
const day = date.getDate().toString().padStart(2, '0');
const month = (date.getMonth() + 1).toString().padStart(2, '0'); // Month is 0-indexed
const year = date.getFullYear();
return `${day}/${month}/${year}`;
}
const currentDate = new Date();
console.log(formatDateManually(currentDate));
You can see the output in the screenshot below after I ran the code using Visual Studio Code.
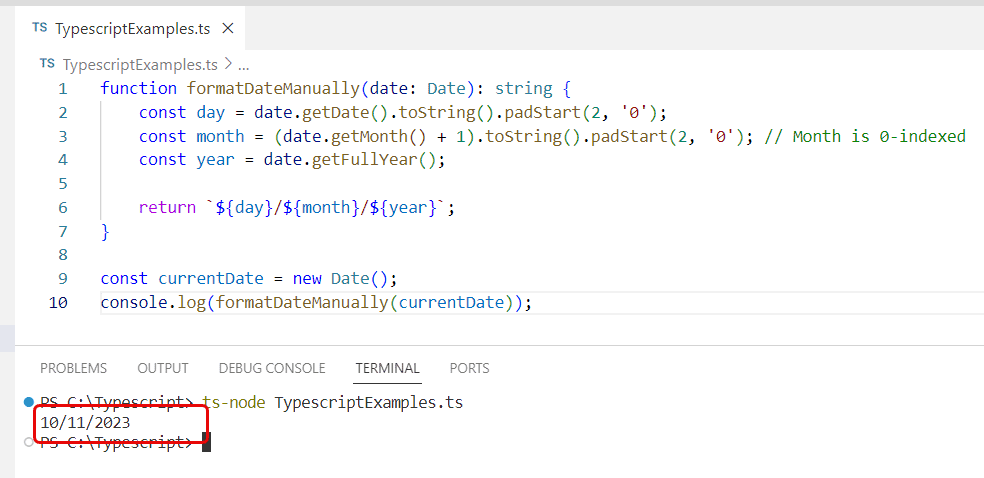
Convert date to string format dd/mm/yyyy using Intl.DateTimeFormat
Now, let us see how to convert the date to string format dd/mm/yyyy in Typescript using the Intl.DateTimeFormat.
The Intl.DateTimeFormat object is a constructor for objects that enable language-sensitive date and time formatting. We can use it to format our date in the DD/MM/YYYY format.
Here is the complete code:
function formatDateWithIntl(date: Date): string {
return new Intl.DateTimeFormat('en-GB').format(date);
}
const currentDate = new Date();
console.log(formatDateWithIntl(currentDate));
You can see the complete code to convert date to string format dd/mm/yyyy in Typescript using Intl.DateTimeFormat.
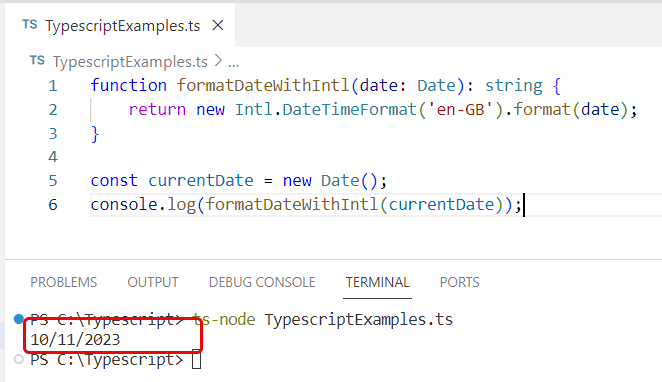
Conclusion
Converting dates to a specific string format in TypeScript is a common requirement for Typescript developers. In Typescript, you can use various methods like toLocaleDateString, manual formatting, or Intl.DateTimeFormat, etc. I hope you have an idea now of how to convert date to string format dd/mm/yyyy in Typescript.
You may also like:
- Get month day year from date in Typescript
- How to Format Date in Typescript
- TypeScript Date Add Days
- How to Calculate Date Difference in Days in Typescript?
- How to Remove Time from Date in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com