Do you want to know how you subtract days from current date in Typescript? In this tutorial, I have explained various methods to subtract days from current date in Typescript with examples and complete code.
To subtract days from the current date in TypeScript, you can use the Date object’s setDate() and getDate() methods. For instance, to subtract 10 days from the current date, create a new Date object and set its day to 10 days less than today: let currentDate = new Date(); currentDate.setDate(currentDate.getDate() – 10);. This approach is straightforward and utilizes TypeScript’s built-in Date functionalities.
Subtract Days from Current Date in Typescript
TypeScript, a strongly typed superset of JavaScript, provides robust ways to handle date operations, including subtracting days from the current date.
Now let us check the below 3 methods:
Using the Date Object
The Date object in TypeScript is inherited from JavaScript and is used to work with dates and times. To subtract days from the current date in Typescript, we first need to create a Date object representing the current date and time.
To subtract days, we manipulate the Date object by setting the day to the current day minus the number of days we wish to subtract.
Here is a complete example.
function subtractDays(days: number): Date {
let date = new Date();
date.setDate(date.getDate() - days);
return date;
}
let tenDaysAgo = subtractDays(10);
console.log(tenDaysAgo);
Output:
2023-11-02T07:21:47.318Z
You can check the screenshot below after I ran the code using Visual Studio Code:
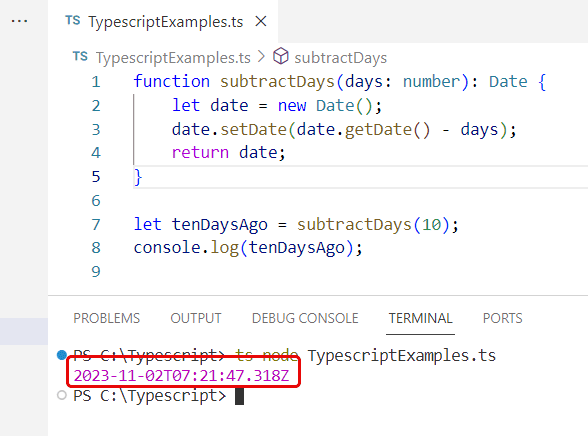
Using Time Arithmetic
Another way to subtract days in Typescript is by using time arithmetic. In TypeScript, dates are internally represented as the number of milliseconds since January 1, 1970 (Unix Epoch). Therefore, we can subtract days by converting them into milliseconds.
function subtractDaysTimeArithmetic(days: number): Date {
const millisecondsPerDay = 24 * 60 * 60 * 1000;
let date = new Date();
date.setTime(date.getTime() - days * millisecondsPerDay);
return date;
}
let fiveDaysAgo = subtractDaysTimeArithmetic(5);
console.log(fiveDaysAgo);
You can see the screenshot below:
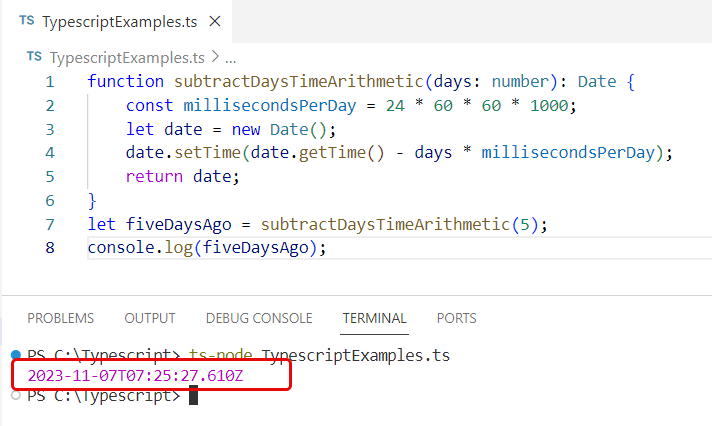
Using Third-Party Libraries
For more complex date manipulations, third-party libraries like date-fns or moment.js can be extremely useful. These libraries offer a wide range of date operations with minimal effort. You can use libraries like date-fns or moment.js to subtract days from the current date in Typescript.
Using date-fns
import { subDays } from 'date-fns';
let sevenDaysAgo = subDays(new Date(), 7);
console.log(sevenDaysAgo);
Using moment.js
import * as moment from 'moment';
let threeDaysAgo = moment().subtract(3, 'days').toDate();
console.log(threeDaysAgo);
Conclusion
In TypeScript, subtracting days from the current date can be done in several ways, including using the native Date object, time arithmetic, or third-party libraries. Each method has its own advantages and can be chosen based on the complexity of the task and the requirements of your project. In this tutorial, I have explained three different methods to subtract days from the current date in Typescript.
You may also like:
- How to Check If Date is Valid in Typescript?
- How to Check Date Less Than Today in Typescript?
- How to create date from year month day in Typescript?
- How to Get First and Last Day of Week in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com