In TypeScript, the Date type is a powerful tool for managing dates and times. The Date type in TypeScript is an object that represents a moment in time, typically expressed as a date and time of day. Here, we will go through the Typescript date and also the new date in Typescript.
Typescript Date
You can use the Date object to work with date manipulation in TypeScript. This built-in object to do all date and time-related things. To initialize a new Date object, you can either use the constructor without arguments to capture the current date and time or pass specific arguments to tailor the date to your requirements.
Here is the complete code:
// Current date and time
const dateNow = new Date();
console.log(dateNow);
// Specific date: Nov 10, 2023
const dateCustom = new Date(2023, 10, 10);
console.log(dateCustom);
Once you run the code using Visual Studio code, you can see the output in the screenshot below.
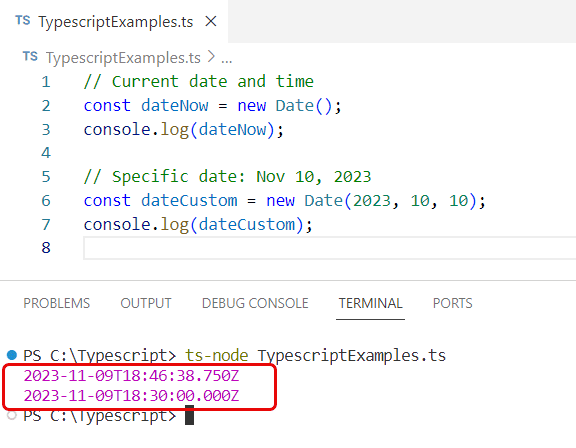
Once you have your Date object in Typescript, you can access and modify its properties. TypeScript provides a set of methods to retrieve and adjust the year, month, day, and other components.
Here is an example.
const date = new Date();
// Get year and month
const year = date.getFullYear();
const month = date.getMonth();
console.log(`Current Year: ${year}, Current Month: ${month}`);
// Set year and month
date.setFullYear(2023);
date.setMonth(11);
You can see the output in the screenshot below.
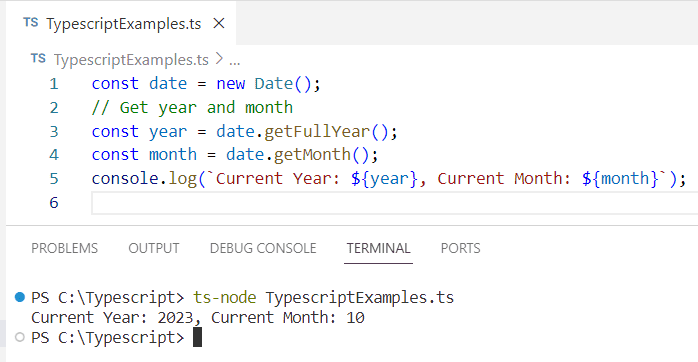
Typescript new date
The new Date() constructor is your gateway to creating date objects representing a single moment in time in TypeScript. These date objects are based on a time value that is the number of milliseconds since January 1, 1970, UTC.
Here is how to instantiate a new date object in Typescript.
let currentDate = new Date();
console.log(currentDate); // Outputs the current date and time
The above Typescript code prints the current date and time to the console.
In Typescript, you can also create a date object for a specific date and time like the below code:
let specificDate = new Date('2023-01-01T00:00:00');
console.log(specificDate);
And, if you want to construct a date from individual components like year, month, and day, you can write the below Typescript code:
let dateFromComponents = new Date(2023, 0, 1); // Note: Month is 0-indexed
console.log(dateFromComponents);
Typescript also provides various methods for getting and setting the various components of a date.
Here are some of the get method examples in Typescript date.
let date = new Date();
// Get full year
let year = date.getFullYear();
console.log(year); // Outputs the current year
// Get month (0-indexed)
let month = date.getMonth();
console.log(month); // Outputs the current month - 1
// Get day of the month
let day = date.getDate();
console.log(day); // Outputs the current day of the month
In the same way, you can see there are some setting methods in Typescript date.
let date = new Date();
// Set full year
date.setFullYear(2024);
console.log(date); // Outputs the date set to the year 2024
// Set month (0-indexed)
date.setMonth(11);
console.log(date); // Outputs the date set to December of the current year
// Set day of the month
date.setDate(25);
console.log(date); // Outputs the date set to the 25th of the current month
Conclusion
Now, I hope you understand how to work with the Typescript date object, and also understand the new Date() Typescript constructor and associated methods in TypeScript. TypeScript’s Date object is always helpful in working with the current date and time in Typescript.
You may like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com