Do you want to know about the Typescript override keyword? In this Typescript tutorial, I will show you how to use the override Keyword in Typescript with a complete example.
To use the ‘override’ keyword in TypeScript, declare it in a subclass method that intentionally overrides a method in its superclass. This keyword enhances code clarity and helps prevent errors by ensuring the overridden method exists and matches in the base class. For example, in a Dog class extending an Animal class, use override makeSound() in Dog to explicitly override the makeSound method in Animal.
What is the override Keyword in Typescript?
The ‘override’ keyword in TypeScript is important for developers working with class inheritance. It explicitly denotes that a method in a subclass is intended to override a method in its superclass. This explicit declaration serves two primary purposes:
- Enhanced Code Clarity: It becomes immediately clear to anyone reading the code that the method is overriding another.
- Error Prevention: The TypeScript compiler checks for the existence of the method in the base class. If the base class method is missing, renamed, or the signatures don’t match, it throws an error, thus preventing potential runtime issues.
Typescript override keyword example
Now, let us see, how to use the override keyword in Typescript with an example.
Base Class: Vehicle
First, we have a base class named Vehicle with a method startEngine.
class Vehicle {
startEngine() {
console.log("Engine started");
}
}
Derived Class: Car
Next, we create a subclass Car that extends Vehicle. In Car, we override the startEngine method using the override keyword.
class Car extends Vehicle {
override startEngine() {
console.log("Car engine started with a roar!");
}
}
In this example, the Car class overrides the startEngine method from the Vehicle class. The use of the override keyword explicitly indicates that the method in Car is intentionally overriding the method in Vehicle.
To test this code, you can create an instance of Car and call the startEngine method:
const myCar = new Car();
myCar.startEngine();
Here is the complete code:
class Vehicle {
startEngine() {
console.log("Engine started");
}
}
class Car extends Vehicle {
override startEngine() {
console.log("Car engine started with a roar!");
}
}
const myCar = new Car();
myCar.startEngine();
You can see the output in the screenshot below once I ran the code using Visual Studio Code.
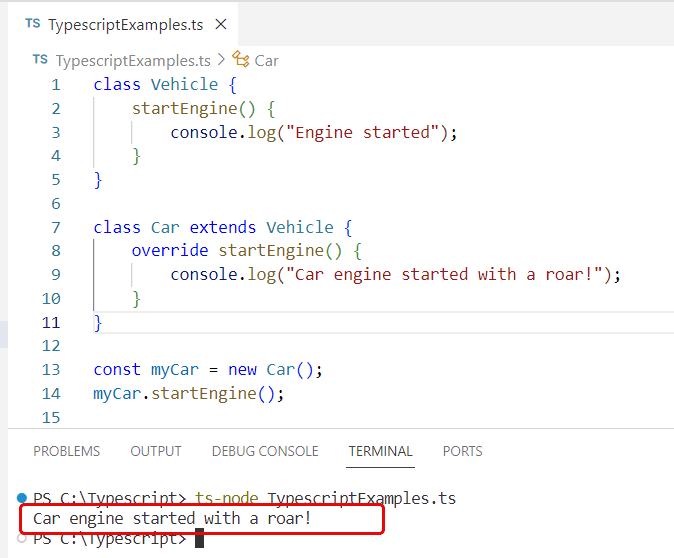
Here are a few points to remember while using the Typescript override keyword.
- Always Use ‘override’ When Overriding Methods: This clarifies your intentions and leverages TypeScript’s error-checking capabilities.
- Keep Method Signatures Consistent: Ensure that the overriding method has the same signature as the method in the base class to avoid errors.
- Refactor Carefully: When changing method names or signatures in base classes, remember to update all derived classes. TypeScript’s error messages can guide you in this process.
Conclusion
I hope you got an idea of the Typescript override keyword. I have explained the override keyword in Typescript with a complete example.
You may also like:
- as Keyword in Typescript
- this Keyword in Typescript
- unique Keyword in TypeScript
- is Keyword in Typescript
- super Keyword in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com