Do you want to know about the Typescript “is” keyword? In this Typescript tutorial, I will explain how to use the “is” keyword in Typescript.
The “is” keyword in TypeScript is used for type guarding, allowing developers to narrow down the type of an object within a conditional block. By using this keyword, you can specify that a certain variable is of a specific type in a given scope, enhancing type safety and code reliability in TypeScript applications. This feature is particularly useful for validating object types and ensuring that the correct methods or properties are accessible on those objects.
What is the “is” Keyword in TypeScript?
TypeScript, known for its strong typing capabilities, includes the “is” keyword as a type guard. This keyword helps in narrowing down the type of an object within a conditional block. Essentially, it tells the TypeScript compiler that a certain variable is of a specific type within a certain scope.
Why Use the “is” Keyword in Typescript?
The “is” keyword in Typescript is beneficial in scenarios where you need to confirm the type of a variable before proceeding with operations that are specific to that type. It improves code reliability and maintainability by ensuring type safety at compile time.
is Keyword in Typescript examples
Let us check out a few examples of the “is” keyword in Typescript to know how the “is” keyword works in TypeScript.
Here is a basic example:
interface Bird {
fly(): void;
}
interface Fish {
swim(): void;
}
function isFish(pet: Bird | Fish): pet is Fish {
return (pet as Fish).swim !== undefined;
}
let pet: Bird | Fish;
// Using the 'is' keyword
if (isFish(pet)) {
pet.swim();
} else {
pet.fly();
}
In this example, isFish is a type guard function that checks if pet is of type Fish. If pet is a Fish, it will have a swim method, and the code within the if block will execute. Otherwise, it falls back to the else block where pet is treated as a Bird.
Here is another advanced example of how to use the “is” keyword in Typescript.
class Circle {
constructor(public radius: number) {}
draw(): void {
console.log("Drawing a circle");
}
}
class Square {
constructor(public side: number) {}
draw(): void {
console.log("Drawing a square");
}
}
function isCircle(shape: any): shape is Circle {
return shape instanceof Circle;
}
let shape: Circle | Square = new Circle(10);
if (isCircle(shape)) {
console.log("Radius:", shape.radius);
} else {
// Type assertion to treat shape as Square in this block
let square = shape as Square;
console.log("Side:", square.side);
}
shape.draw();
You can see the output in the screenshot below after I ran the code using Visual Studio code.
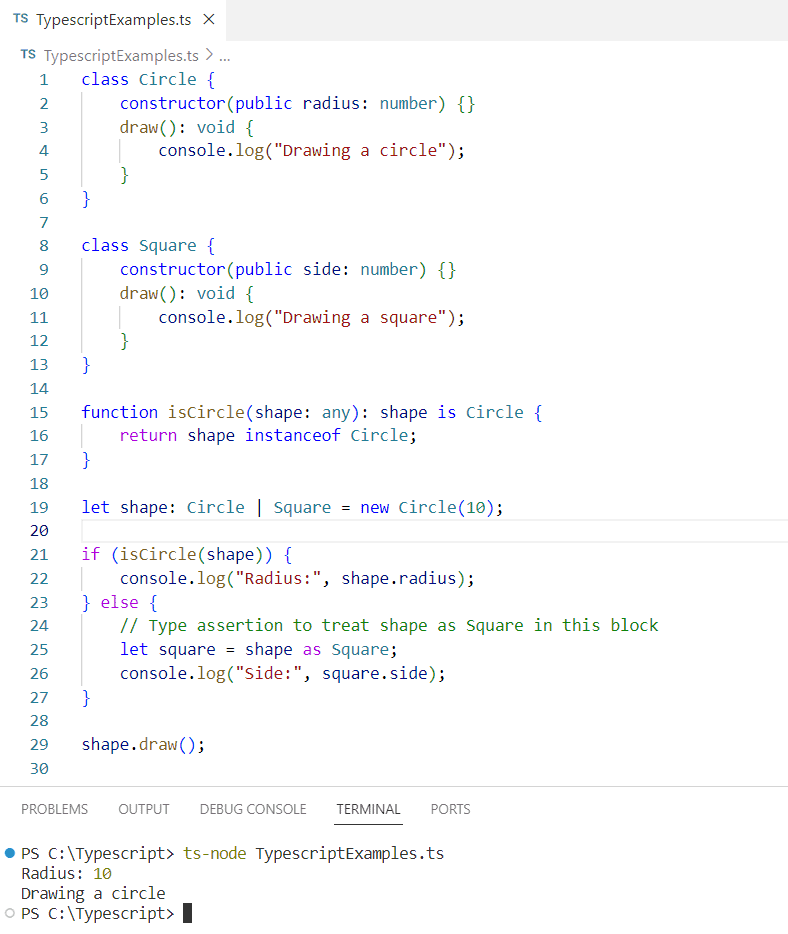
Conclusion
The “is” keyword in TypeScript is a powerful feature for ensuring type safety and clarity in your code. In this Typescript tutorial, I have explained two examples of how to use the “is” keyword effectively in your TypeScript projects.
You may also like:
- as Keyword in Typescript
- this Keyword in Typescript
- type keyword in Typescript
- override Keyword in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com