Do you want to know about the “this keyword in typescript“? In this Typescript tutorial, I will explain everything about this keyword in Typescript.
this keyword in Typescript refers to the context in which a function is executed. Its value varies: within a class method, it represents the class instance; in regular functions, it depends on the invocation method; and in arrow functions, it inherits the context from the enclosing scope. Understanding this is crucial for managing scope and object references effectively in TypeScript.
What is the “this” Keyword in TypeScript?
The this keyword in TypeScript is a special variable that represents the context in which a function is executed. It’s a reference to the object that owns the function at the time it is called. Understanding this is essential for effective TypeScript programming, as it influences how methods and functions interact with object properties.
Contextual Usage of “this” in Typescript
The value of this in TypeScript varies depending on the context in which a function is invoked:
- In a Method: When used inside a method of a class, this refers to the instance of the class.
- In a Function: In a regular function, the value of this depends on how the function is called.
- In an Arrow Function: Arrow functions don’t have their own this. Instead, they inherit this from the parent scope.
Examples of “this” Keyword in TypeScript
To better understand this, let’s look at some examples of this keyword in Typescript.
class Car {
constructor(public model: string) {}
displayThis() {
console.log(this); // Here, 'this' refers to the instance of Car
}
}
let myCar = new Car("Tesla Model S");
myCar.displayThis();
In the above example, this within displayThis() method refers to the instance of the Car class.
You can see the screenshot below for the output after I ran the code using Visual Studio Code:
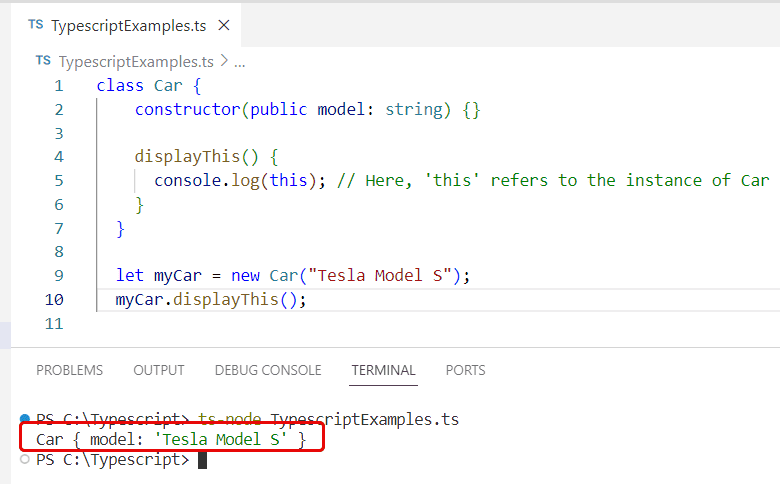
Handling “this” in Callbacks and Events
A common issue with this in TypeScript arises in callbacks and event handlers. In such cases, this might not refer to what you expect. To handle this, you can:
- Use an arrow function.
- Bind the function to the desired this context.
Best Practices for Using “this” Keyword
- Consistent Context: Ensure that the context of this remains consistent within your class or function.
- Arrow Functions: Utilize arrow functions for callbacks to maintain the context of this.
- Avoid using this in static methods: In static methods, this refers to the class itself, not an instance.
Conclusion
I hope now you have an idea of how to use the “this” keyword in Typescript.
You may also like:
- type keyword in Typescript
- as Keyword in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com