Do you want to know how to use the Typescript this keyword? In this Typescript tutorial, I will explain to you how to use the type keyword in Typescript with a few real examples.
To use the ‘type’ keyword in TypeScript, you define a type alias that can represent a simple type, a union or intersection of types, or an object shape. This enhances code readability and maintainability. For example, type StringOrNumber = string | number; creates a type alias that can be either a string or a number, simplifying the usage of complex type annotations in your TypeScript code.
What is the “type” Keyword in TypeScript?
The ‘type’ keyword in TypeScript is used to declare a custom type alias. A type alias is essentially a name given to a type or a combination of types. It simplifies complex type annotations, making your code more readable and maintainable. The ‘type’ keyword can be used with primitives, arrays, objects, and even complex structures.
How to use the Typescript “type” keyword?
Here is a simple example of how to use the “type” keyword in Typescript.
type StringOrNumber = string | number;
let myVariable: StringOrNumber;
myVariable = 'Hello'; // Valid
myVariable = 123; // Valid
In this example, StringOrNumber is a type alias for a union type that can be either a string or a number in the Typescript code.
You can also use ‘type’ to define more complex structures, like objects in Typescript.
type User = {
name: string;
age: number;
isActive: boolean;
};
const user: User = {
name: 'John Doe',
age: 30,
isActive: true
};
This example shows how ‘type’ can be used to define the shape of an object, making it easier to manage and understand object structures in your codebase.
Here is a complete example of how to use the type keyword in Typescript.
Let’s consider an example where we use the ‘type’ keyword in TypeScript to define a custom type and then use it in a function to see the output.
First, we’ll define a type alias named StringOrNumber which can be either a string or a number. Then, we’ll create a function named displayInfo that takes a parameter of this type and logs it to the console.
// Define a type alias
type StringOrNumber = string | number;
// Function that uses the StringOrNumber type
function displayInfo(input: StringOrNumber) {
console.log(`The input is: ${input}`);
}
// Test the function with different types of inputs
displayInfo("Hello, TypeScript!"); // Pass a string
displayInfo(2023); // Pass a number
In this example, when you call displayInfo with different types of arguments (once with a string and once with a number), the function will log these values to the console, demonstrating how the StringOrNumber type alias works in practice.
You can see the output after I ran the code using Visual Studio Code:
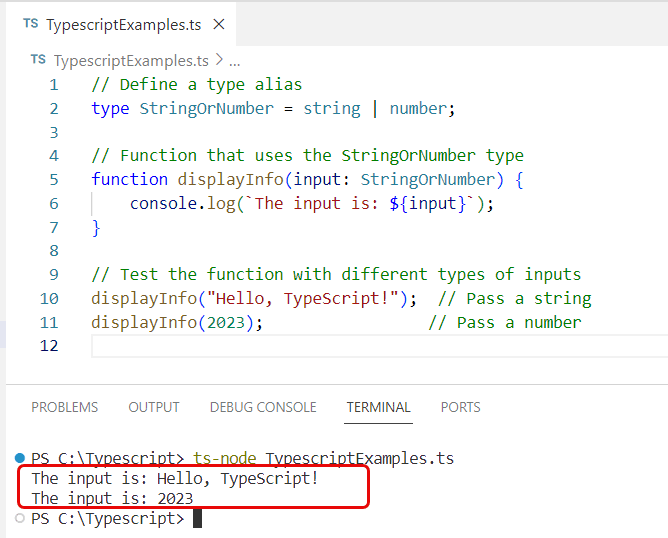
When to Use ‘type’ Over ‘interface’ in Typescript
The choice between ‘type’ and ‘interface’ can sometimes be confusing in Typescript. As a rule of thumb, use ‘type’ to define a union or intersection type or a type that cannot be extended or implemented. Use ‘interface’ to define the shape of an object that can be extended or implemented.
Example of Union Type with ‘type’ in Typescript
type Coordinates = [number, number];
type NamedLocation = { name: string; coordinates: Coordinates };
type GeoLocation = Coordinates | NamedLocation;
const location1: GeoLocation = [45.0, 75.0]; // Valid
const location2: GeoLocation = { name: "Central Park", coordinates: [40.785091, -73.968285] }; // Valid
Best Practices for Using ‘type’ in TypeScript
- Consistency: Be consistent in your use of ‘type’ and ‘interface’. Mixing them unnecessarily can lead to confusion.
- Readability: Choose clear and descriptive names for your type aliases.
- Modularity: Break down complex types into smaller, reusable types to keep your codebase clean and manageable.
Conclusion
The ‘type’ keyword in TypeScript is a powerful tool for creating custom types and enhancing the type safety of your code. Understanding its usage, especially when compared to ‘interface’, is key to writing effective and maintainable TypeScript code. I hope you got an idea of how to use the “type keyword in Typescript“.
You may also like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com