Do you want to know about the Typescript super keyword? In this Typescript tutorial, I will show you how to work with the super keyword in Typescript with real examples and complete code.
In TypeScript, the super keyword is used within a subclass to access the parent class’s methods and constructors. It enables subclasses to inherit and extend the functionalities of their parent classes. Specifically, super is essential for calling the parent class’s constructor and overriding subclass methods.
What is the super Keyword in Typescript?
In TypeScript, the super keyword is used within a derived class (subclass) to refer to its parent class. It serves two main purposes:
- To call the constructor of the parent class: This is essential when extending a class and adding new properties.
- To access methods on a parent class: This allows subclasses to leverage or modify functionalities of the parent class.
Typescript super keyword examples
Let us check a few examples of Typescript super keyword.
Example 1: Using super in Constructors
Let’s consider a real-world application with a generic User class, and a specialized AdminUser class that extends it. Here is the complete code:
class User {
name: string;
constructor(name: string) {
this.name = name;
}
greet() {
return `Hello, my name is ${this.name}`;
}
}
class AdminUser extends User {
adminLevel: string;
constructor(name: string, adminLevel: string) {
super(name); // Call the constructor of User
this.adminLevel = adminLevel;
}
adminGreet() {
return `${super.greet()} and I am an ${this.adminLevel} admin.`;
}
}
// Usage
const admin = new AdminUser("Alice", "Expert");
console.log(admin.adminGreet());
Once you run the code using VS code, you can see the output in the screenshot below.
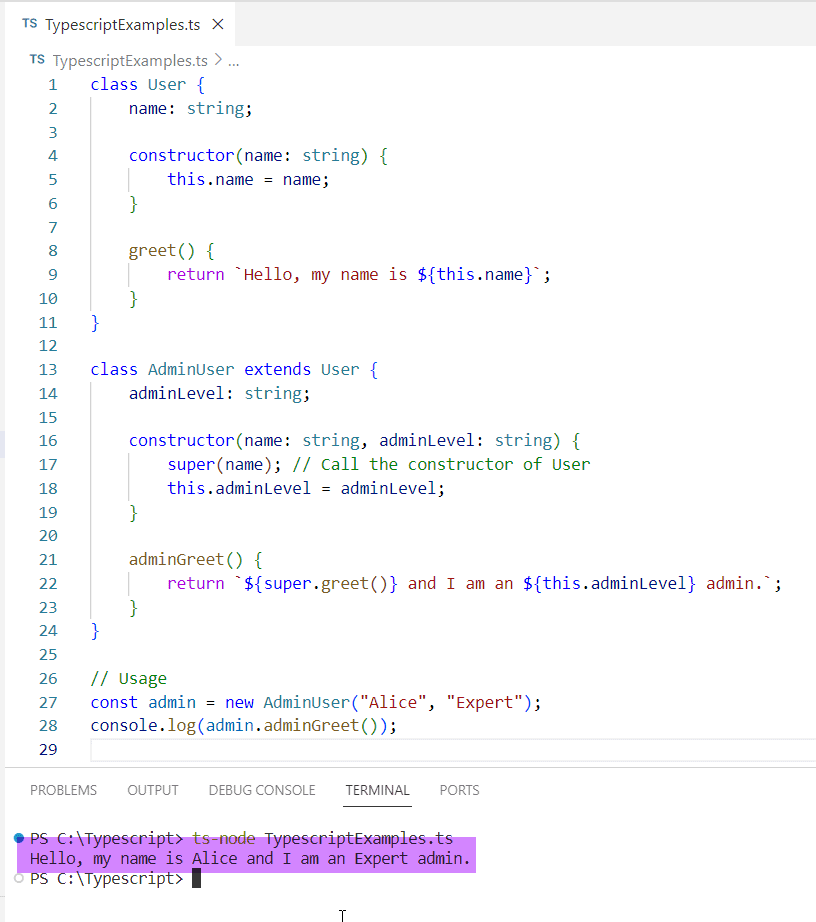
- AdminUser extends User.
- super(name) in AdminUser’s constructor calls the constructor of User, setting the name.
- super.greet() accesses the greet method from the parent User class.
Example 2: Overriding Methods and Using super
Now, let’s say we want to override the greet method in AdminUser but also use part of the functionality from the User class. Here is the complete code:
class User {
name: string;
constructor(name: string) {
this.name = name;
}
greet() {
return `Hello, my name is ${this.name}`;
}
}
class AdminUser extends User {
adminLevel: string;
constructor(name: string, adminLevel: string) {
super(name); // Call the constructor of User
this.adminLevel = adminLevel;
}
// Overriding the greet method in the subclass
greet() {
return `${super.greet()} and I am an admin.`; // Using super.greet() to access the greet method from User
}
}
// Usage
const admin = new AdminUser("Bob", "Master");
console.log(admin.greet()); // Outputs: Hello, my name is Bob and I am an admin.
Here is the code explanation:
- The
AdminUser
class extends theUser
class. - In
AdminUser
, we override thegreet
method. Inside this method, we usesuper.greet()
to call thegreet
() method from the parentUser
class, and then we add additional text to customize it for theAdminUser
. - When we create an instance of
AdminUser
and callgreet()
, it now uses the overridden method inAdminUser
, which includes the original greeting fromUser
plus the additional admin-specific message.
Once you run the code using Visual Studio code, you can see the output in the screenshot below:
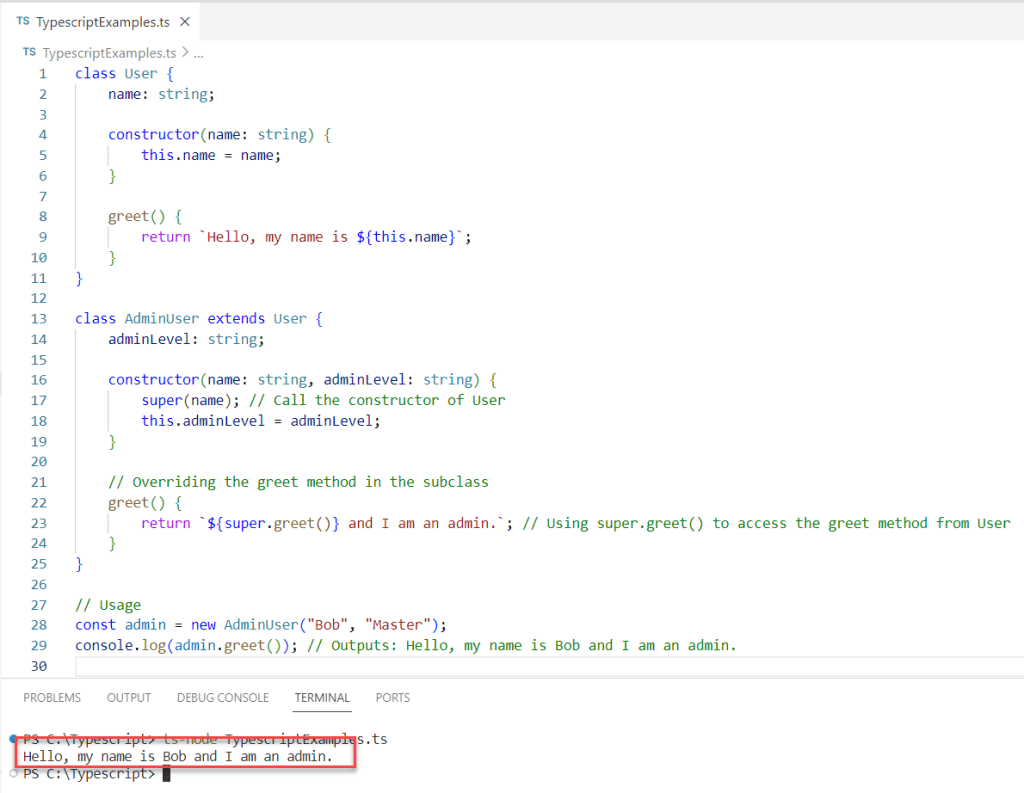
Conclusion
The super keyword in TypeScript is a powerful feature for object-oriented programming. In this Typescript tutorial, I have explained how to work with the super keyword in Typescript with two useful examples.
You may also like:
- declare Keyword in Typescript
- const Keyword in Typescript
- extend Keyword in Typescript
- readonly Keyword in Typescript
- arguments keyword in Typescript
- typeof Keyword in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com