Do you want to know about the Typescript const keyword? In this Typescript tutorial, I will explain to you how to use the const Keyword in Typescript with various examples.
The const keyword in TypeScript is used to declare variables that cannot be reassigned after their initial assignment. These variables are block-scoped and must be initialized at declaration. While the binding to the variable is immutable, the contents of objects or arrays assigned to const variables can still be modified.
What is the const keyword in Typescript?
In TypeScript, const is a keyword used to declare a variable. However, it’s not just any variable declaration; it cannot be reassigned after its initial assignment. Here are a few things you should know about the const keyword:
- Block Scope: const variables are block-scoped, much like let. They are only accessible within the block where they are defined.
- Immutable Binding: The const declaration creates an immutable binding with the variable, meaning you cannot reassign a new value to it. However, it’s crucial to note that if the variable holds an object or array, the contents of the object or array can still be altered.
- Initialization Requirement: Variables declared with const must be initialized at the time of declaration. Failure to do so results in a syntax error.
Syntax of const Typescript keyword
Here is the syntax for the const keyword in Typescript.
const variableName = value;
How to use the const Keyword in Typescript?
Now, let us understand how to use the const keyword in Typescript with a few examples.
1. Here is a basic and simple usage of the const keyword in Typescript.
const pi = 3.14;
console.log(pi); // Output: 3.14
// Trying to reassign the value of pi
pi = 3.14159; // This will throw an error
In this example, pi is a constant whose value cannot be changed once set. You can see in the screenshot below that when I tried to assign a new value; it was throwing an error as “error TS2588: Cannot assign to ‘pi’ because it is a constant.“
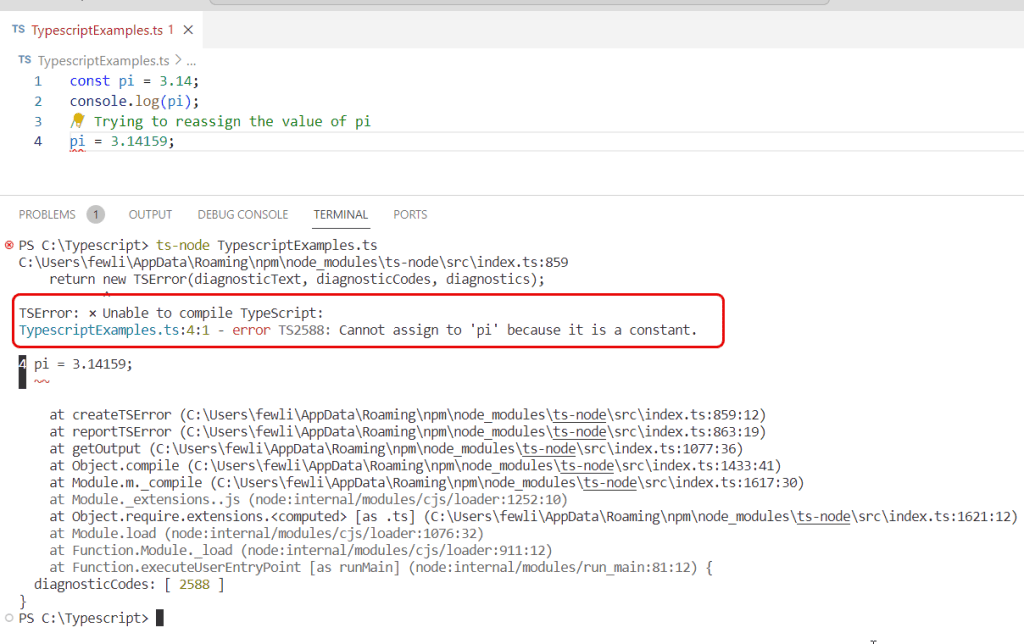
2. In the next example, you will see how to use the const keyword in block scope in Typescript. Check out the below code:
if (true) {
const a = "Inside block";
console.log(a); // Output: Inside block
}
console.log(a); // Error: a is not defined
You can see that after I ran the code using VS code, it is showing me an error as “error TS2304: Cannot find name ‘a’.“. Here, a is accessible only within the if-block, not outside of the if-block.
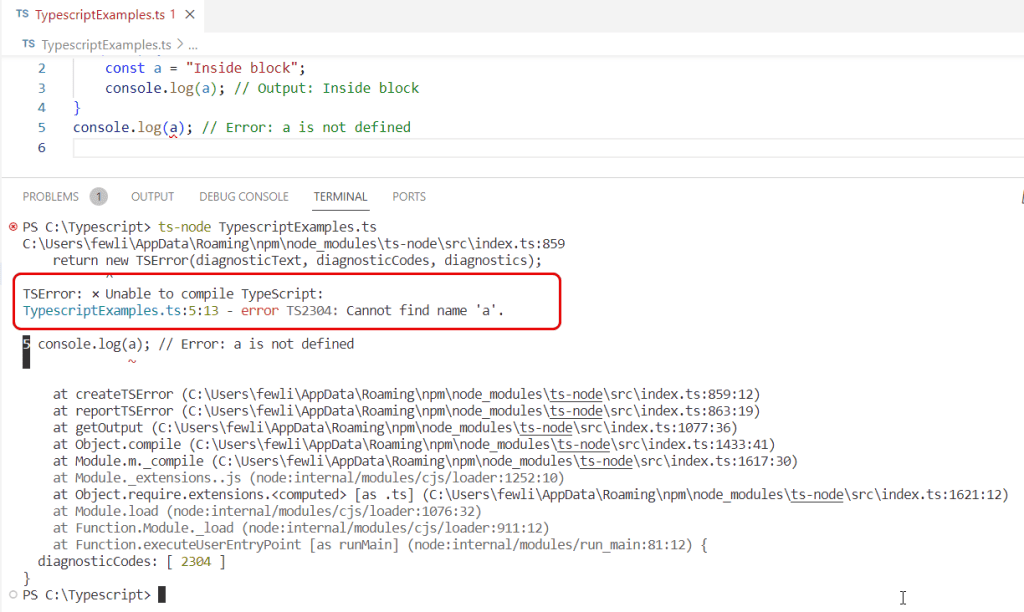
3. We can also use the const keyword in the Typescript array. Here is an example:
const person = { name: "John", age: 30 };
// Modifying the object's property is allowed
person.age = 31;
console.log(person); // Output: { name: "John", age: 31 }
// Reassigning the object is not allowed
person = { name: "Jane", age: 25 }; // Error
You can see in the screenshot below Reassigning the object is not allowed, which is why it is showing the error “error TS2588: Cannot assign to ‘person’ because it is a constant.” when I ran the code using VS code.
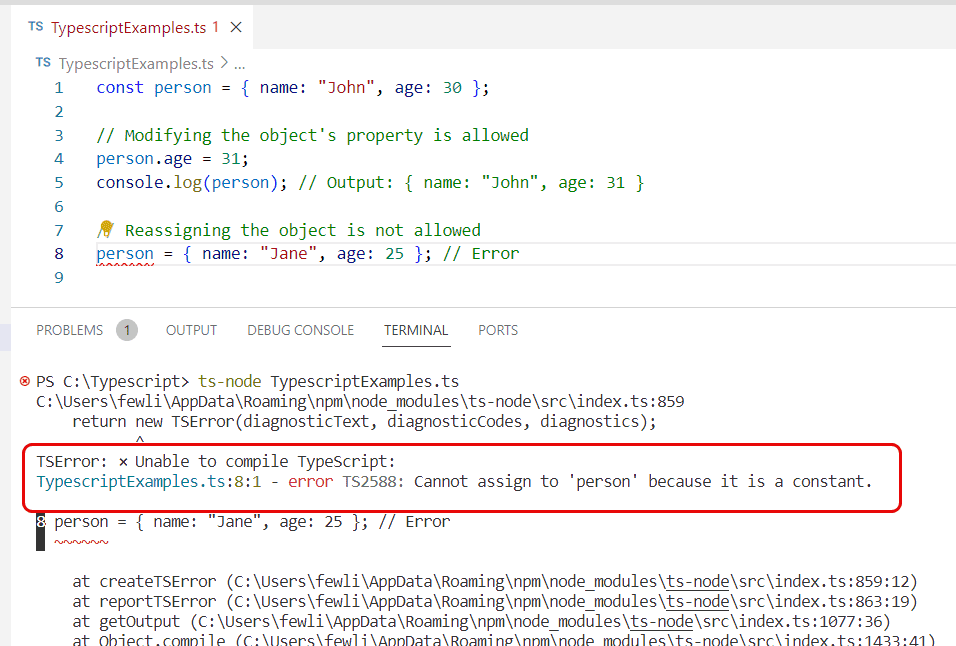
Here is another example of how to work with const in Typescript arrays.
const numbers = [1, 2, 3];
numbers.push(4);
console.log(numbers); // Output: [1, 2, 3, 4]
// Reassigning the array is not allowed
numbers = [5, 6, 7]; // Error
Here, you can see you can modify the array’s elements, but reassigning a new array to numbers is forbidden. Check out the screenshot below.
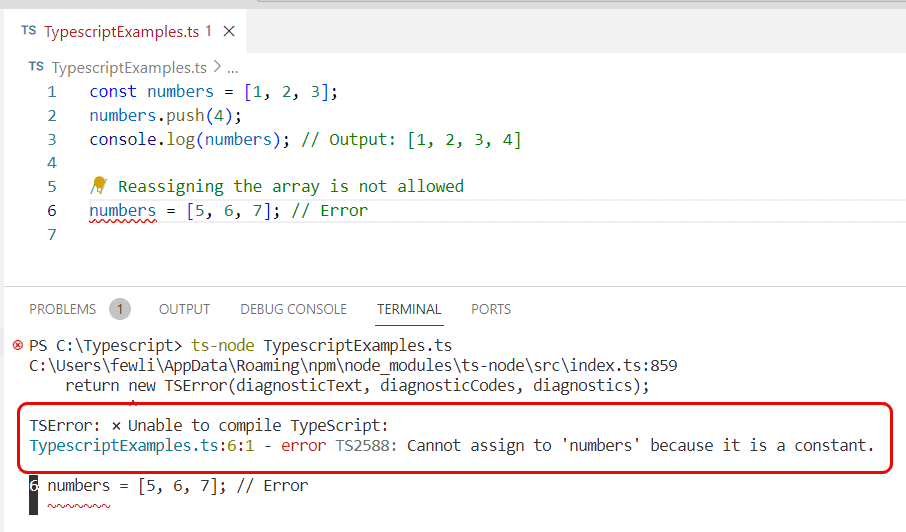
Conclusion
The const keyword in TypeScript is a powerful feature that allows you to declare variables with immutable bindings. This immutability applies to the variable’s binding and not necessarily to its value, especially in the case of objects and arrays. In this tutorial, I have explained everything about the const keyword in Typescript and how to use the const keyword in Typescript using various examples.
You may also like:
- type keyword in Typescript
- as Keyword in Typescript
- this Keyword in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com