Do you want to know about the Typescript typeof keyword? In this Typescript tutorial, I have explained how to use the typeof keyword in Typescript, its syntax, and Typescript typeof keyword examples.
The typeof keyword in TypeScript is used primarily in two ways: as a type query and as a type guard. As a type query, it captures the type of a variable or property at compile time, allowing for type reuse and refactoring. As a type guard, it checks the type of a variable at runtime, ensuring type safety in functions and conditional statements. This dual functionality makes typeof an essential tool for maintaining type integrity in TypeScript code.
What is typeof in TypeScript?
In TypeScript, typeof is used in two primary contexts:
- Type Query: It captures the type of a variable or property at compile time.
- Type Guard: It checks the type of a variable at runtime.
typeof is most effective with primitive types (e.g., string, number). It is less useful with complex objects.
Using typeof for Type Queries
Type queries are useful for creating new types based on existing variable types. Let’s dive into an example:
let sampleVar = "Hello, TypeScript!";
type SampleVarType = typeof sampleVar;
// SampleVarType is now 'string'
let anotherVar: SampleVarType;
anotherVar = "Another string"; // OK
anotherVar = 123; // Error: Type 'number' is not assignable to type 'string'
In this example, SampleVarType becomes a type alias for the type of sampleVar, which is string.
Using typeof for Type Guards
Type guards are a runtime feature of TypeScript, where typeof is used in conditional checks to ensure a certain type of variable is being used. This is particularly useful in functions:
function process(input: string | number) {
if (typeof input === "string") {
console.log("It's a string:", input);
} else {
console.log("It's a number:", input);
}
}
process("Hello");
process(42);
Here, typeof checks whether input is a string or a number, allowing the function to handle each type appropriately.
Advanced typeof Typescript Example: Combining typeof with Custom Types
You can combine typeof with custom types for more complex scenarios in Typescript. For instance:
interface Person {
name: string;
age: number;
}
const person: Person = { name: "John Doe", age: 30 };
type PersonType = typeof person;
function greet(person: PersonType) {
console.log(`Hello, ${person.name}!`);
}
greet(person);
This example demonstrates how to use typeof to capture the type of a custom object and reuse it in a function in Typescript. You can see the output in the screenshot below.
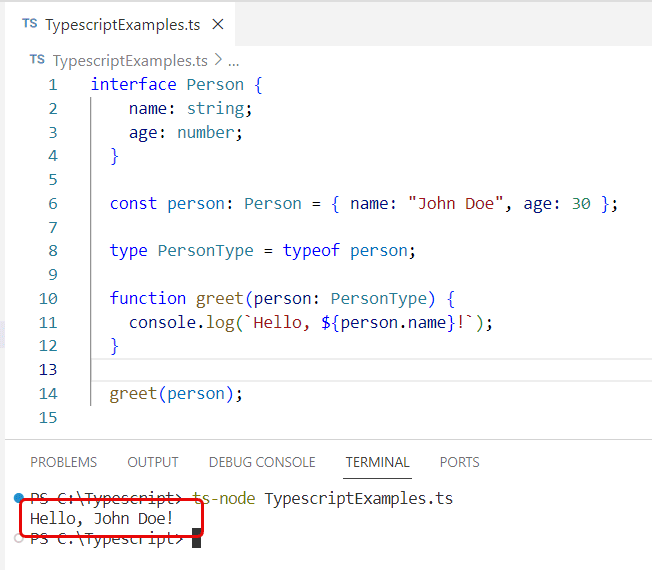
Conclusion
In this Typescript tutorial, I have clearly explained how to use the typeof Keyword in Typescript.
You may also like:
- throw Keyword in Typescript
- operator Keyword in Typescript
- async Keyword in Typescript
- await Keyword in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com