Do you want to check file size in PowerShell? In this PowerShell tutorial, I will explain everything about PowerShell get file size with various methods and examples.
To check the file size using PowerShell, you can use the Get-Item command followed by the .Length property. For instance, $fileSize = (Get-Item “C:\path\to\your\file.txt”).Length will retrieve the size of file.txt in bytes. This method is straightforward and efficient for quickly obtaining the file size in PowerShell.
PowerShell get file size
Now, let us see various methods to get file size in PowerShell.
In PowerShell, you can use the Get-Item PowerShell cmdlets to get file size. The Get-Item command in PowerShell is a basic and straightforward way to get the properties of a file, including its size. Get-Item is primarily used to obtain details about files and directories.
Get-Item is most commonly used to get properties of file system objects. When applied to a file, it returns an object that includes various properties such as the file’s name, directory, size (Length), creation time, last access time, and more.
Get-Item supports the use of wildcards, making it useful for retrieving items that match a certain pattern. For example, Get-Item C:\Documents*.txt would retrieve all .txt files in the specified directory.
The basic syntax of the Get-Item command is
Get-Item -Path <path-to-item>
The -Path parameter specifies the path to the item you want to retrieve. This path can be an absolute path or a relative path.
Here is an example of “PowerShell file size”.
$file = Get-Item "C:\Bijay\Dummy\spguides.pdf"
$fileSize = $file.Length
Write-Host "File Size: $fileSize bytes"
- $file = Get-Item “C:\Bijay\Dummy\spguides.pdf”: This command fetches the file properties of the specified file.
- $fileSize = $file.Length: Here, we extract the Length property from the file object, which represents the file size in bytes.
- Write-Host “File Size: $fileSize bytes”: Finally, we print the file size to the console.
You can see the output in the screenshot below once you run the code using Windows PowerShell ISE.
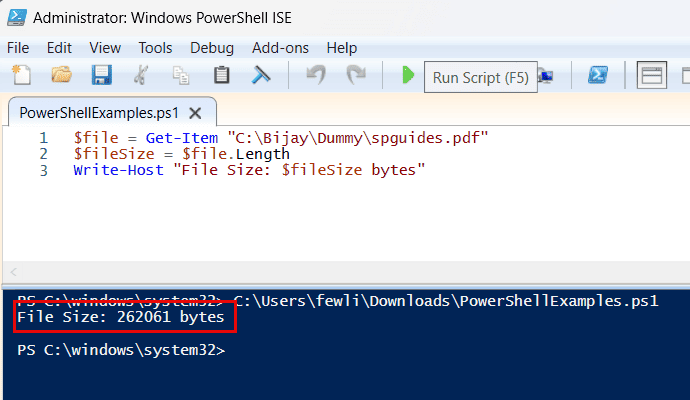
PowerShell check file size using Get-ChildItem for Multiple Files
If you want to check the size of multiple files in a directory, then you can use the Get-ChildItem PowerShell command.
Here is a complete example of how to get file size in PowerShell (multiple files).
$files = Get-ChildItem "C:\Bijay\Dummy\*"
foreach ($file in $files) {
$fileSize = $file.Length
Write-Host "$($file.Name) - Size: $fileSize bytes"
}
- $files = Get-ChildItem “C:\Bijay\Dummy\*”: This retrieves all files in the specified directory.
- foreach ($file in $files): We loop through each file.
- Inside the loop, we again use $file.Length to get each file’s size and print it alongside the file name.
Once you run the code using PowerShell ISE, you can see the output below in the screenshot:
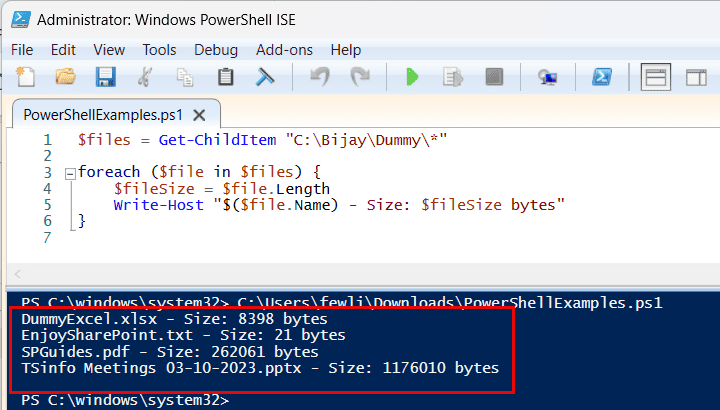
get file size in PowerShell in KB, MB, GB
Now, let us see how to get file size in PowerShell in KB, MB, and GB format. Working with file sizes in bytes might not be very user-friendly. Let’s convert the file size to a more readable format (KB, MB, GB).
Here is the complete PowerShell script.
function Format-FileSize {
param([long]$size)
if ($size -gt 1GB) {
"{0:N2} GB" -f ($size / 1GB)
} elseif ($size -gt 1MB) {
"{0:N2} MB" -f ($size / 1MB)
} elseif ($size -gt 1KB) {
"{0:N2} KB" -f ($size / 1KB)
} else {
"$size bytes"
}
}
$file = Get-Item "C:\Bijay\Dummy\SPGuidesMeetings.pptx"
$fileSize = Format-FileSize -size $file.Length
Write-Host "File Size: $fileSize"
- Format-FileSize is a custom function that takes a size in bytes and converts it to a more readable format.
- It checks if the size is greater than 1 GB, MB, or KB and formats the output accordingly.
- $fileSize = Format-FileSize -size $file.Length: We use this function to format the size of our file.
Once you run the PowerShell script, you can see the output in the screenshot below:
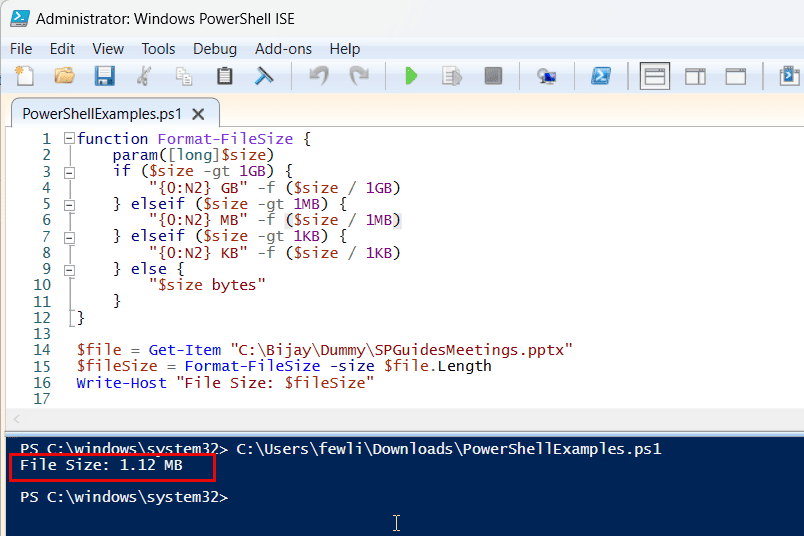
Conclusion
PowerShell offers powerful ways to work with files and their properties, including file size. The examples above provide different methods to retrieve and display file sizes, from simple byte counts to more readable formats. In this PowerShell tutorial, I explained how to get file size in PowerShell using the Get-Item and the Get-ChildItem PowerShell cmdlets for multiple files.
You may also like:
- SharePoint download multiple files
- PowerShell Copy Item examples
- PowerShell Create Log File
- How to loop through a PowerShell array
- How to create an array in PowerShell from CSV file
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com