Are you looking for a few examples of how to use PowerShell Copy Item? This tutorial explains how to use Copy-Item in PowerShell. Also, we will discuss the following points:
- PowerShell copy-item
- PowerShell Copy Item recurse subfolders
- PowerShell Copy Item create folder/PowerShell copy item to new directory
- PowerShell copy item without folder
- PowerShell copy specific file type
- PowerShell copy item exclude
- PowerShell copy item exclude folder
- PowerShell copy-item exclude files
- PowerShell copy-item exclude file types
- PowerShell copy-item exclude empty folders
- PowerShell copy-item exclude hidden files
- PowerShell copy item excludes existing files
- PowerShell copy item overwrite
- PowerShell copy-item overwrite directory
- PowerShell copy-item progress
- PowerShell copy item from network share
- PowerShell copy item Merge multiple folders
- PowerShell copy item and rename the file
PowerShell Copy-Item
We can use Copy-Item to copy an item from one location to another. The command only copies the item, it will not cut or delete the item after being copied. But Copy-Item can copy and rename the item also. We just need to pass the new name in the Destination parameter in the PowerShell copy-item command.
According to the MSDN, the syntax for copy-item is below:
Copy-Item
[-Path] <String[]>
[[-Destination] <String>]
[-Container]
[-Force]
[-Filter <String>]
[-Include <String[]>]
[-Exclude <String[]>]
[-Recurse]
[-PassThru]
[-Credential <PSCredential>]
[-WhatIf]
[-Confirm]
[-FromSession <PSSession>]
[-ToSession <PSSession>]
[<CommonParameters>]
Now, let us see a few examples of PowerShell copy-item. To write, execute, and debug the PowerShell script, I will use Windows PowerShell ISE.
Example-1:
In the first example, we will see how to copy a file from one location to another location using Copy-Item.
Copy-Item "D:\Source\Test.docx" -Destination "D:\Bijay\Destination"
You can see the file has been copied to the destination location.
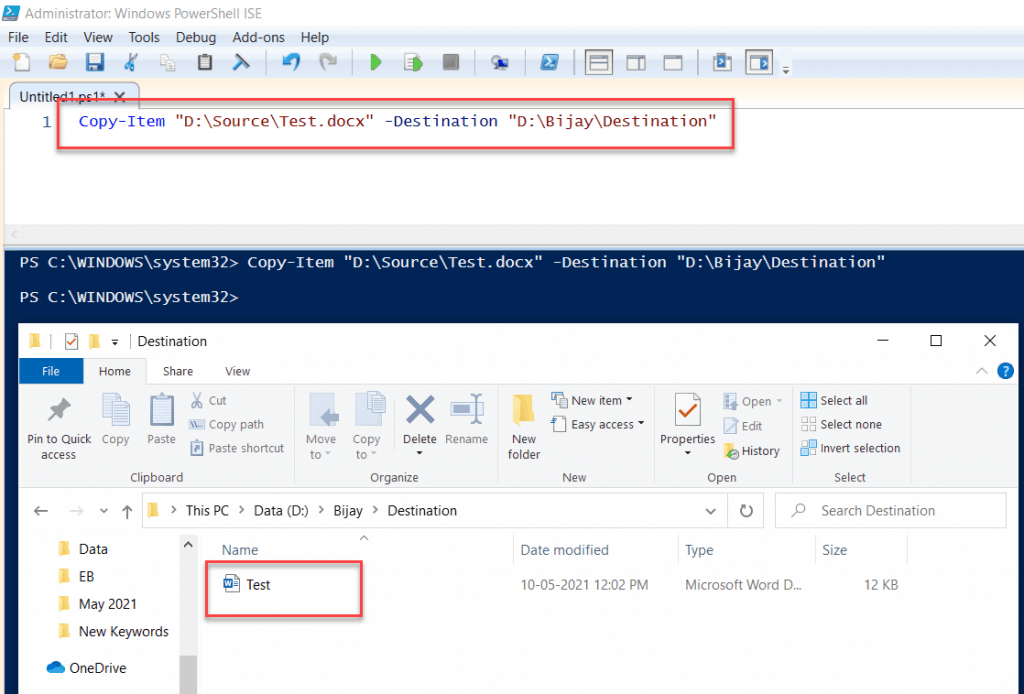
Example-2:
In the next example, we will see how to copy all files from one location to another location. Here I have a few different types of files in the Source folder. By using the PowerShell Copy-Item cmdlet, we will copy all the files from the source folder to the destination folder.
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination"
You can see the output:
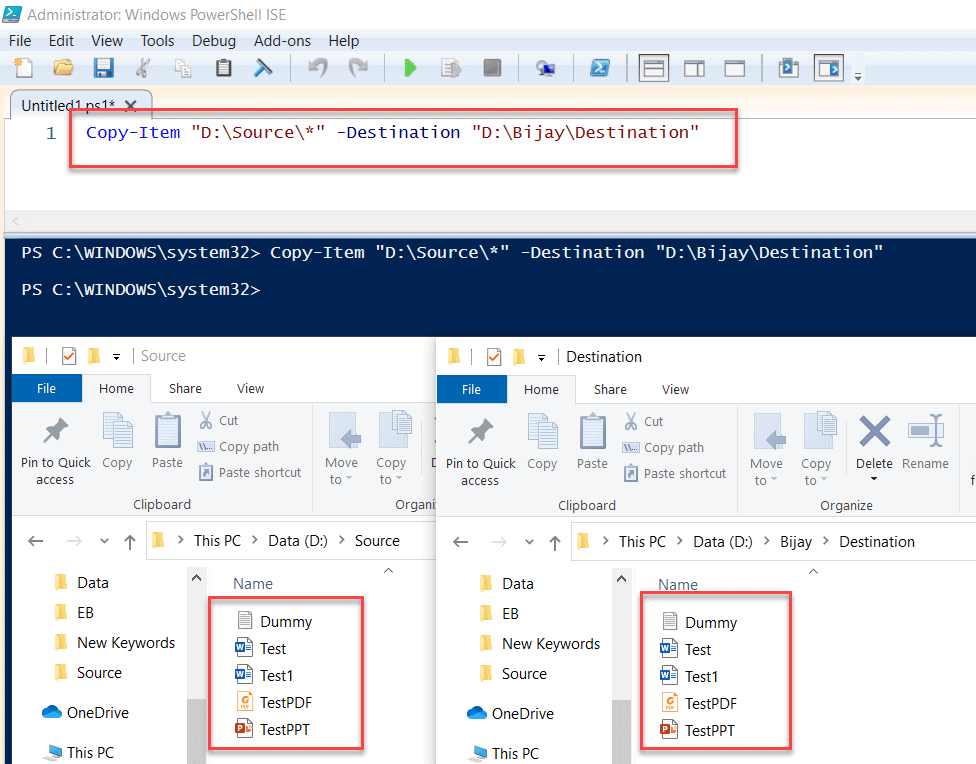
This is how we can copy all files from one location to another location using Copy-Item PowerShell command.
PowerShell Copy Item recurse subfolders
Now, let us see how to copy item recurse subfolders in PowerShell.
In this case, in the Source folder, I have a subfolder that has a few files. When I run the below command, it will just create the folder, but it did not copy the files that are presented in the sub folder.
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination"
In fact, if the subfolder has additional nested subfolder, it will not create the nested subfolder when you run the Copy-Item PowerShell cmdlet.
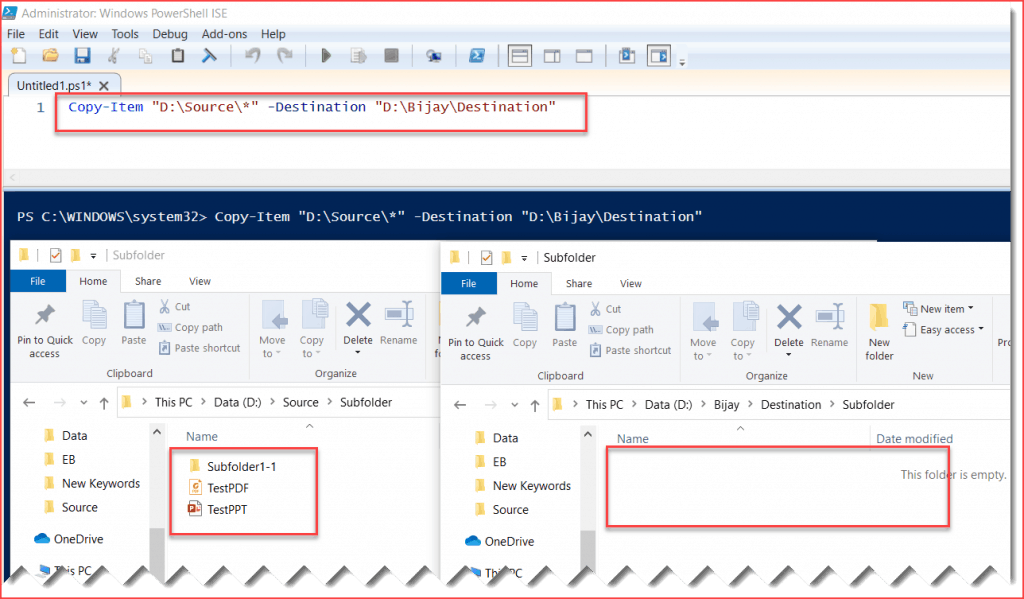
To copy items recursively, we need to use the -Recurse parameter with the Copy-Item PowerShell cmdlet. The complete PowerShell cmdlets look like below:
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination" -Recurse
Once you run the above command, you can see it creates the subfolder, including nested subfolders and files to the destination folder.
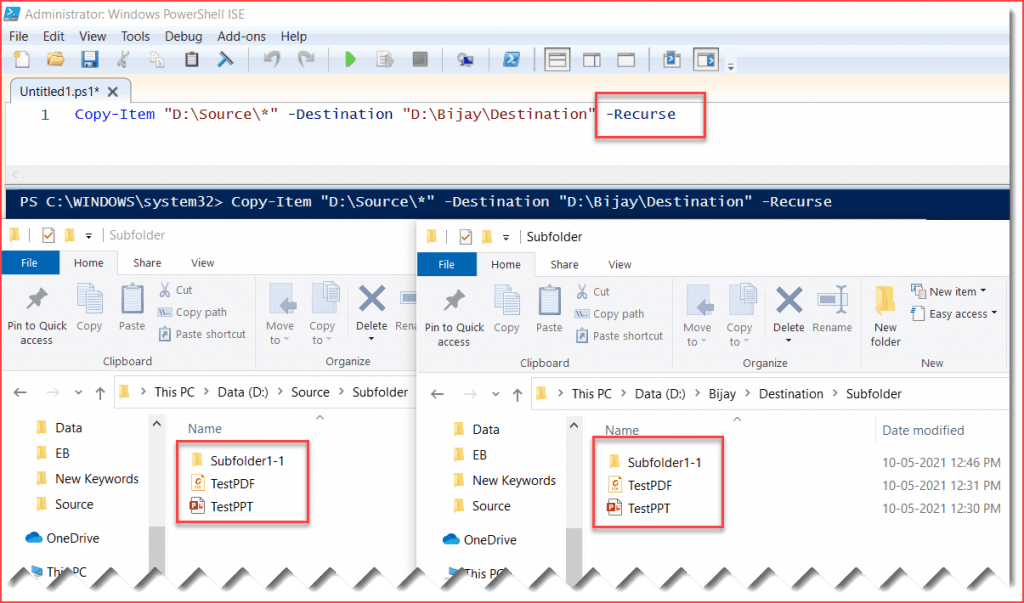
This is an example of PowerShell Copy Item recurse subfolders.
PowerShell Copy Item create folder
Now, let us see how to copy items from the source folder and save them into a new folder in the destination. In this case, the files and folders from the Source folder will be copied to a new folder as NewFolder in the destination location.
Copy-Item "D:\Source" -Destination "D:\Bijay\Destination\NewFolder" -Recurse
Here in the Destination Parameter, we need to provide a folder name like above.
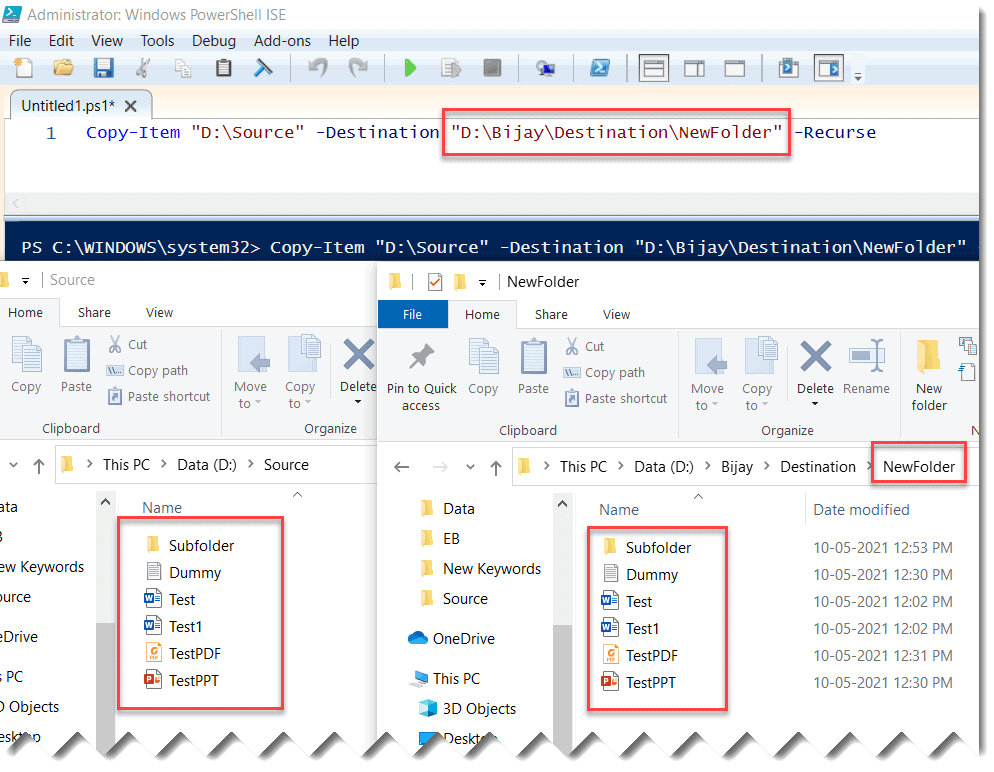
This is how we can copy files to a new folder in the destination using the PowerShell copy-item cmdlet. The same script, we can use to PowerShell copy item to new directory.
PowerShell copy item without folder
If you want to copy items or files without folders, then we can write the PowerShell Copy-Item like below:
Get-ChildItem D:\Source\ -File | Copy-Item -Destination D:\Bijay\Destination
The above command will copy items or files from the main folder only, it will not copy files from sub folders.
But if you want to copy files recursively from subfolders or nest subfolders then we need to use the -Recurse parameter like below:
Get-ChildItem D:\Source\ -File -Recurse | Copy-Item -Destination D:\Bijay\Destination
PowerShell copy specific file type
If you want to copy only specific files, like let us say, we just want to copy the .pdf files using the PowerShell Copy-Item, then you can write the below script.
Copy-Item -Path D:\Source\* -Destination D:\Bijay\Destination -Filter '*.pdf'
You can see below it just copy the .pdf file.
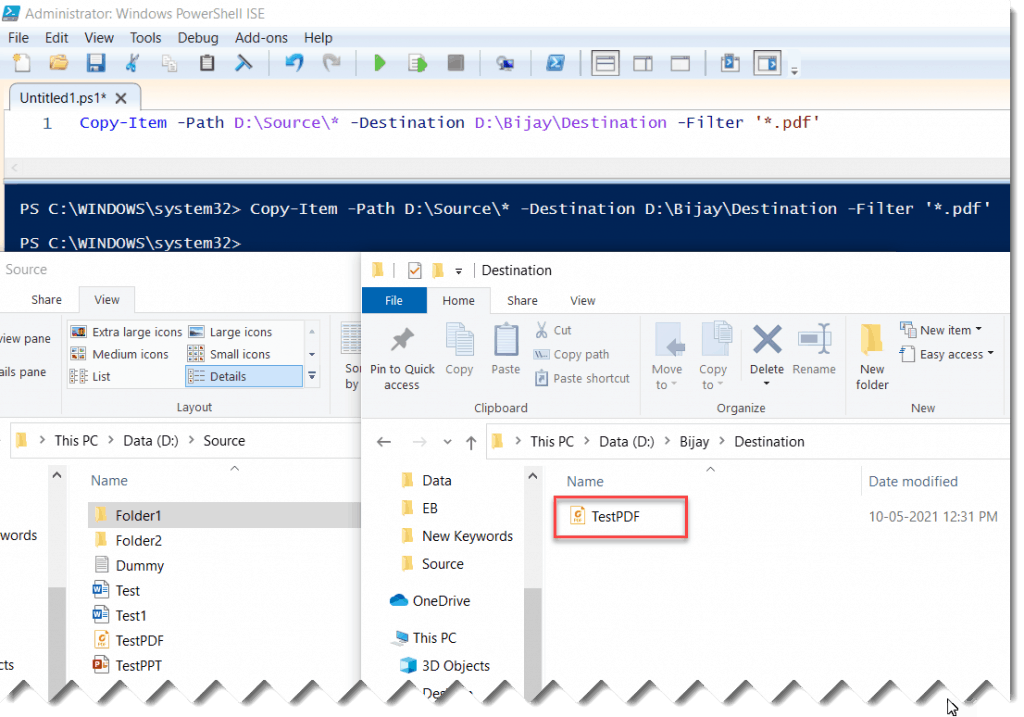
PowerShell copy-item exclude files
Now, let us see how to exclude files while using PowerShell copy-item cmdlets. Here is an example of PowerShell copy-item exclude files.
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination\" -Recurse -Exclude TestPDF.pdf,Test.docx
The above PowerShell script will exclude the TestPDF.pdf and Test.docx files from the Source directory. Copy-Item will also exclude both the files from the subfolders and the nested subfolders.
Or we can even write the PowerShell script like the one below:
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination\" -Recurse -Exclude @("TestPDF.pdf","Test.docx")
We can even write the script like the below:
$exclusionFiles = @("TestPDF.pdf","Test.docx")
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination\" -Recurse -Exclude $exclusionFiles
This is how we can use the PowerShell copy-item exclude files.
PowerShell copy item exclude folder
Now, let us see an example of PowerShell copy item exclude folder.
Here, PowerShell copy-item will exclude folders while copying files.
Copy-Item -Path (Get-Item -Path "D:\Source\*" -Exclude ('Subfolder')).FullName -Destination "D:\Bijay\Destination\" -Recurse -Force
Here it will exclude the Subfolder from the Source while copying.
If you want to exclude multiple folders, like suppose you want to exclude folders name as Folder1, Folder2, then you can write the PowerShell copy-item script like below:
Copy-Item -Path (Get-Item -Path "D:\Source\*" -Exclude ('Folder1', 'Folder2')).FullName -Destination "D:\Bijay\Destination\" -Recurse -Force
As you can see below, the Copy-Item command did not copy the Folder1 and Folder2 to the destination folder.
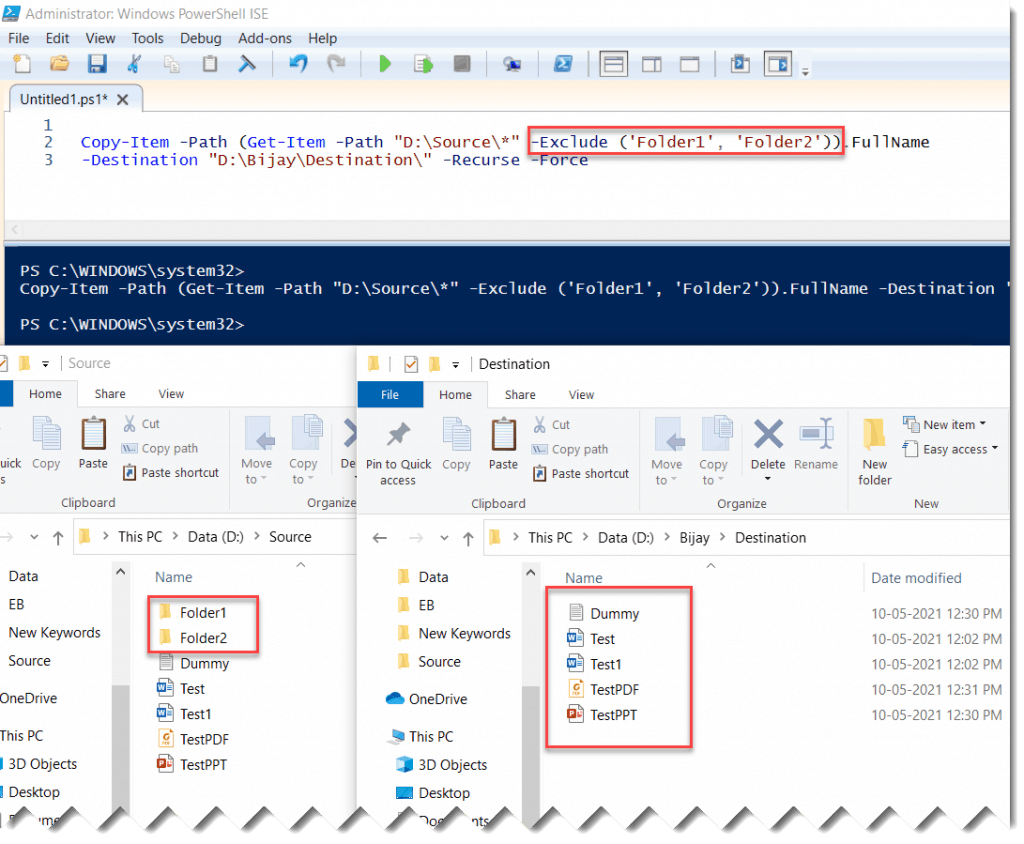
This is how we can exclude folders while copying files using PowerShell copy-item.
PowerShell copy item exclude (Files and Folders)
Now, let us see another example of PowerShell copy item exclude—this time, we will see how to exclude files and folders while using the PowerShell copy item.
Copy-Item -Path (Get-Item -Path "D:\Source\*" -Exclude ('Folder1', 'Test.docx')).FullName -Destination "D:\Bijay\Destination\" -Recurse -Force
The above PowerShell script will exclude a folder name as Folder1, and it also excludes a file name as Test.docx.
In the -Exclude parameter, you can pass many folder names and file names.
PowerShell copy-item exclude file types
Now, let us see how to exclude file types in PowerShell copy-item.
In this example, let us say I do not want to copy the PDF files; then we can write the PowerShell script like below:
$exclusionFiles = @("*.pdf")
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination\" -Recurse -Exclude $exclusionFiles
Note: Here it will exclude the .pdf files from the subfolders and nested subfolders while copying files also.
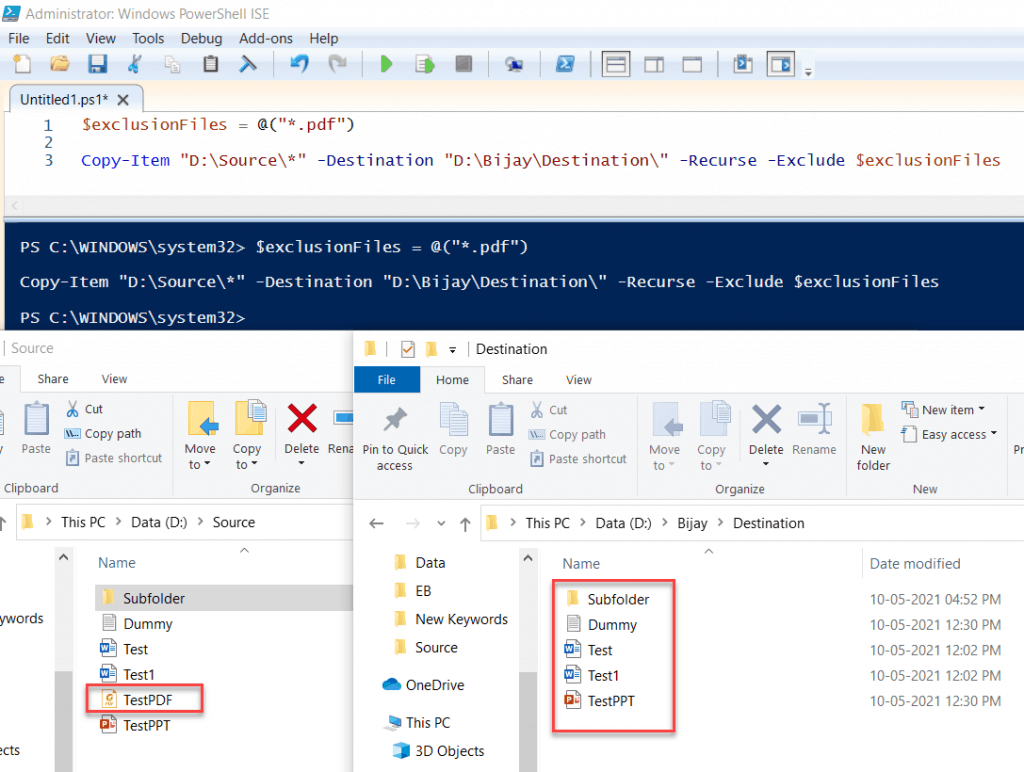
You can see it copy all files from the Source folder and ignore the .pdf files.
If you want more than one file type, then you can write the PowerShell script like below. It will exclude all the files with extensions such as .pdf and .txt.
$exclusionFiles = @("*.pdf","*.txt")
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination\" -Recurse -Exclude $exclusionFiles
This is how we can exclude file types in PowerShell copy-item.
PowerShell copy-item exclude empty folders
Here, we will see another example on PowerShell copy-item exclude empty folders.
The PowerShell script will check and if any folder is empty, it will not copy that folder to the destination folder.
$Source = "D:\Source\"
$items = Get-ChildItem $Source -Force
$Destination="D:\Bijay\Destination\"
ForEach ($item in $items)
{
if((Get-ChildItem -Path $($item.FullName)).Count -gt 0)
{
Copy-Item -Path $item.FullName -Destination $Destination -Force -Recurse
}
}
You can see in the below fig, it ignores or excludes Folder2 as it is an empty folder.
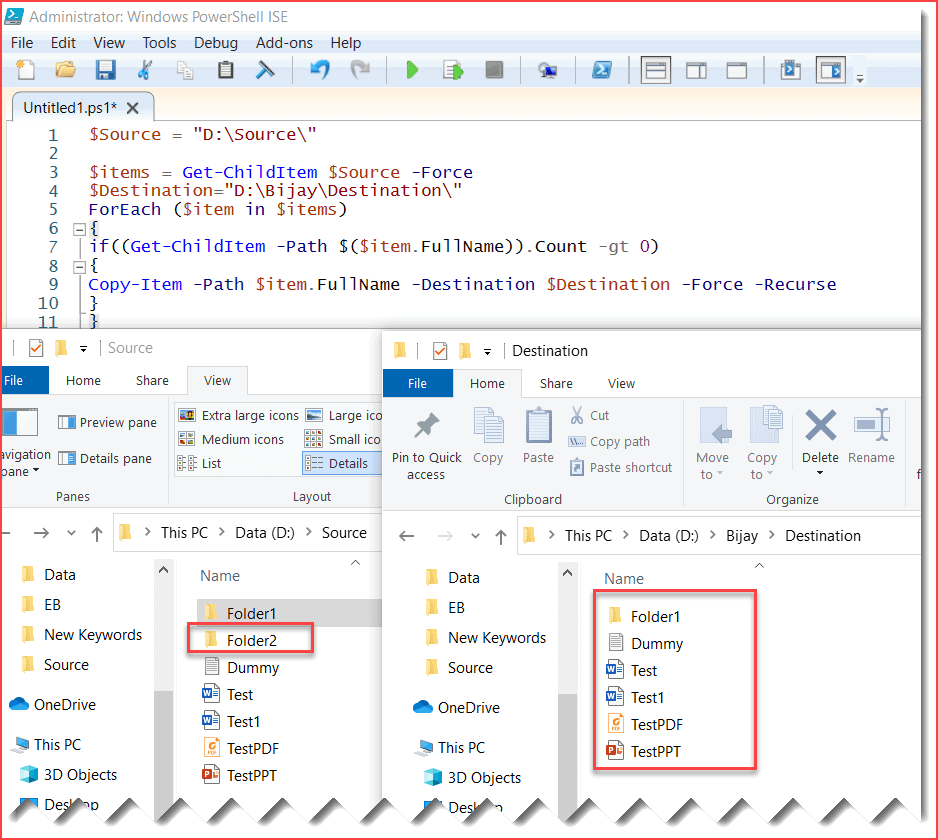
This is how we can exclude empty folders in PowerShell copy-item.
PowerShell copy-item exclude hidden files
There will be a number of times you might not want to copy the hidden files. This example is about PowerShell copy-item exclude hidden files.
While copying item using PowerShell copy-item, it will exclude the hidden files from the Source folder.
ForEach ($FileItem in (gci -Path 'D:\Source' -Recurse))
{ Copy-Item $FileItem.FullName -Destination 'D:\Bijay\Destination' }
Here the gci will do the work for us. If any file is hidden, it will not consider those items.
PowerShell copy item exclude existing files
By default, when you run the PowerShell Copy-Item cmdlet, it will overwrite the file if it already exists.
Here, we will see an example on PowerShell copy item exclude existing files.
Here is the scenario: if a file exists in the destination folder, then it should not copy the item. It should only copy if the file is not exist in the destination folder.
The below PowerShell script will check if the Test.docx file is exists in the destination folder, if it exists, it will ignore the file. If it does not exists, then the Copy-Item will copy the file to the destination folder.
$sourceFile = 'D:\Source\Test.docx'
$destinationFile = 'D:\Bijay\Destination\Test.docx'
if (-not (test-path $destinationFile))
{
Copy-Item $sourceFile -Destination $destinationFile
}
The above PowerShell script will check a single file.
The below PowerShell script will check for each files in the folder. And if the file exists in the destination folder, then it will not copy the file else it will copy the file to the destination folder. It will also check files for folders and subfolders.
$Source = "D:\Source\"
$items = Get-ChildItem $Source -Force
$Destination="D:\Bijay\Destination\"
ForEach ($item in $items)
{
$destinationFile = $Destination+$item.FullName
if (-not (test-path $destinationFile))
{
Copy-Item -Path $item.FullName -Destination $Destination -Force -Recurse
}
}
This is how we can exclude existing files while copying files using PowerShell Copy-Item cmdlets. This is an example of powershell copy-item don’t overwrite.
PowerShell copy item overwrite
By default, when you will copy item in PowerShell using the Copy-Item, it will overwrite the files in the destination folder.
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination" -Recurse
The above PowerShell script will overwrite the files but it will show an error for folders:
Copy-Item : An item with the specified name D:\Bijay\Destination\Folder1 already exists.

We can also add a confirmation message by adding the Confirm:$true parameter like below:
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination" -Recurse -Confirm:$true -Force
When you run the above PowerShell script, it will ask you to confirm. This is how to do, PowerShell copy item overwrite.
PowerShell copy-item overwrite directory
Now, let us see an example on PowerShell copy-item overwrite directory.
The above error can be resolved by adding the -Force parameter to the Copy-Item like below:
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination" -Recurse -Force
The above PowerShell script will overwrite the directory or folder also, it will not give any error like, Copy-Item : An item with the specified name folder name already exists.
PowerShell copy-item progress
If you are copying more files from one folder to another folder using PowerShell, then we might want to display a progress bar. Here is an example of PowerShell copy-item progress.
This PowerShell script, I found in StackOverFlow, and I have used as it is, and it is working fine.
Function Copy-WithProgress
{
[CmdletBinding()]
Param
(
[Parameter(Mandatory=$true,
ValueFromPipelineByPropertyName=$true,
Position=0)]
$Source,
[Parameter(Mandatory=$true,
ValueFromPipelineByPropertyName=$true,
Position=0)]
$Destination
)
$Source=$Source.tolower()
$Filelist=Get-Childitem "$Source" –Recurse
$Total=$Filelist.count
$Position=0
foreach ($File in $Filelist)
{
$Filename=$File.Fullname.tolower().replace($Source,'')
$DestinationFile=($Destination+$Filename)
Write-Progress -Activity "Copying data from '$source' to '$Destination'" -Status "Copying File $Filename" -PercentComplete (($Position/$total)*100)
Copy-Item $File.FullName -Destination $DestinationFile
$Position++
}
}
$src="D:\Data\Songs"
$dest="D:\Bijay\Destination"
Copy-WithProgress -Source $src -Destination $dest
You can see the progress bar look like below:
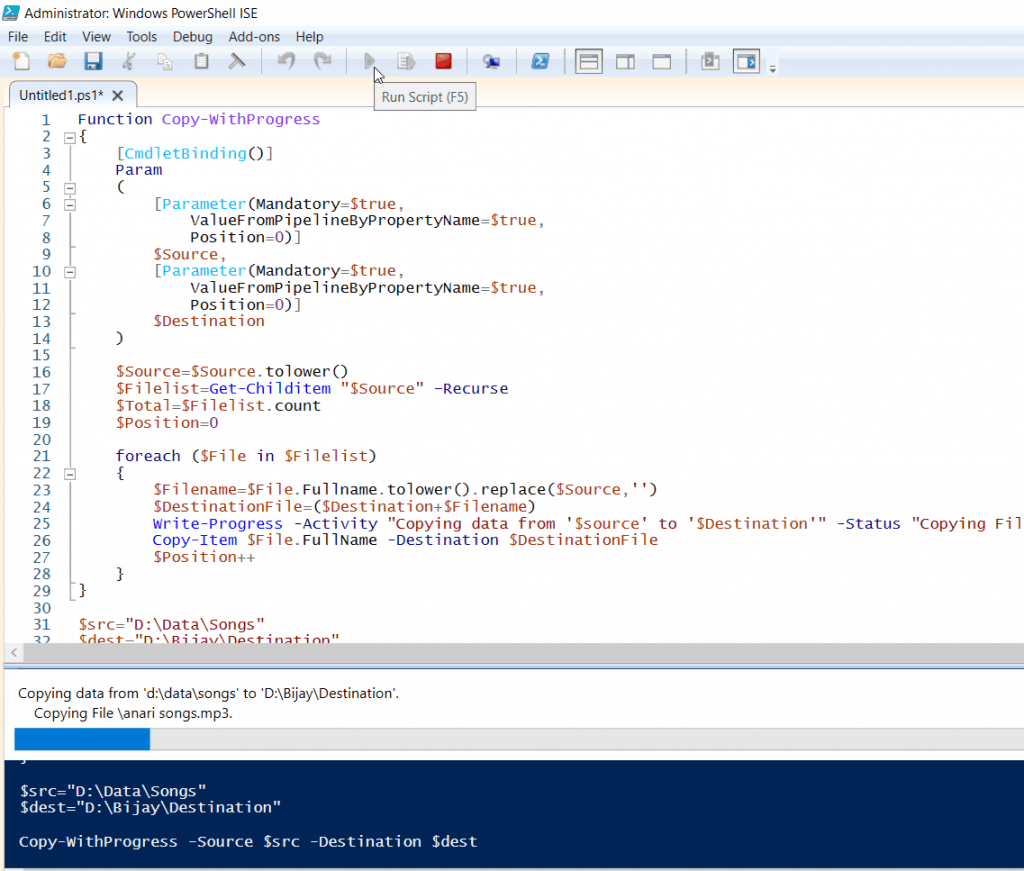
You can see another progress bar as well.
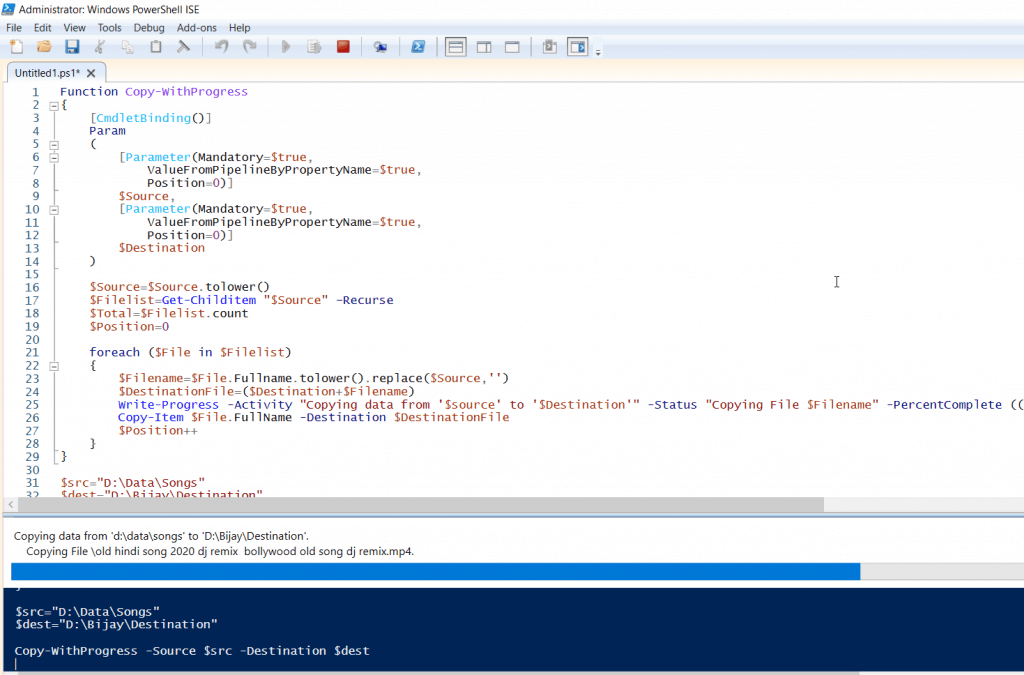
PowerShell copy item with folder structure
Now, let us see how to copy item with folder structure in PowerShell using the Copy-Item cmdlets.
To copy item with folder structure, we need to use -Recurse parameter only in the Copy-Item like below:
Copy-Item "D:\Source\*" -Destination "D:\Bijay\Destination" -Recurse -Force
Once you run the above cmdlets, then it will copy all the items with folder structure in PowerShell. It includes subfolders as well as nested subfolders.
PowerShell copy item from network share
Now, let us see an example on PowerShell copy item from network share.
$Session = New-PSSession -ComputerName "Server01" -Credential "Domain\username"
Copy-Item "D:\SourceFolder\" -Destination "C:\DestinationFolder\" -ToSession $Session
If you want to copy recursively, then add the -Recurse parameter like below:
$Session = New-PSSession -ComputerName "Server01" -Credential "Domain\username"
Copy-Item "D:\SourceFolder\" -Destination "C:\DestinationFolder\" -ToSession $Session -Recurse
This is how we can copy items from network share in PowerShell.
PowerShell copy item Merge multiple folders
Now, let us see how to merge multiple folders while using the PowerShell Copy-Item cmdlets.
Copy-Item -Path D:\Source\*,D:\Source1\*,D:\Source2\* -Destination D:\Bijay\Destination\
The above PowerShell script will copy all the files from Source, Source1 and Source2 folders to the Destination folder.
PowerShell copy item and rename the file
While copying a file using the Copy-Item, we can also rename it. You can follow the below PowerShell script.
Copy-Item -Path D:\Source\Test.docx -Destination D:\Bijay\Destination\NewTest.docx
Here Test.docx file will be copied to the Destination folder, but the file name will be NewTest.docx. The file will be renamed to the new provided file name.
You may like the following PowerShell tutorials:
- PowerShell Create Log File
- How to loop through a PowerShell array
- How to create and use PowerShell ArrayList
- What is PowerShell Array
- How to create an array in PowerShell from CSV file
- Missing argument in parameter list PowerShell
In this PowerShell tutorial, we learned everything about PowerShell Copy Item and covers the below examples:
- PowerShell Copy-Item
- PowerShell Copy Item recurse subfolders
- PowerShell Copy Item create folder
- PowerShell copy item without folder
- PowerShell copy specific file type
- PowerShell copy-item exclude files
- PowerShell copy item exclude folder
- PowerShell copy item exclude (Files and Folders)
- PowerShell copy-item exclude file types
- PowerShell copy-item exclude empty folders
- PowerShell copy-item exclude hidden files
- PowerShell copy item exclude existing files
- PowerShell copy item overwrite
- PowerShell copy-item overwrite directory
- PowerShell copy-item progress
- PowerShell copy item with folder structure
- PowerShell copy item from network share
- PowerShell copy item Merge multiple folders
- PowerShell copy item and rename the file
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com