When you need to split a string by a specific length, PowerShell offers various methods to do it. This PowerShell tutorial explains various methods to split a string by length in PowerShell
To split a string by length in PowerShell, you can use a loop with the Substring method to iteratively extract parts of the string, or leverage regular expressions with [regex]::Matches to match sequences of the desired length. Alternatively, a custom function can be written to handle the splitting process using a while loop manually. Each method efficiently divides the string into chunks of the specified length.
Method 1: Using Substring in a Loop
The best way to split a string by length is by using a loop with the Substring method. This method allows you to extract parts of the string iteratively.
Here’s an example:
function Split-StringByLength {
param (
[string]$string,
[int]$length
)
$result = @()
for ($i = 0; $i -lt $string.Length; $i += $length) {
if ($i + $length -le $string.Length) {
$result += $string.Substring($i, $length)
} else {
$result += $string.Substring($i)
}
}
return $result
}
# Usage
$string = "This is a sample string for splitting."
$length = 5
Split-StringByLength -string $string -length $length
In this script, we define a function Split-StringByLength
that takes a string and the desired length of each chunk. The loop iterates through the string, extracting substrings of the specified length and storing them in an array.
After executing the PowerShell script using VS code, you can see the output in the screenshot below.
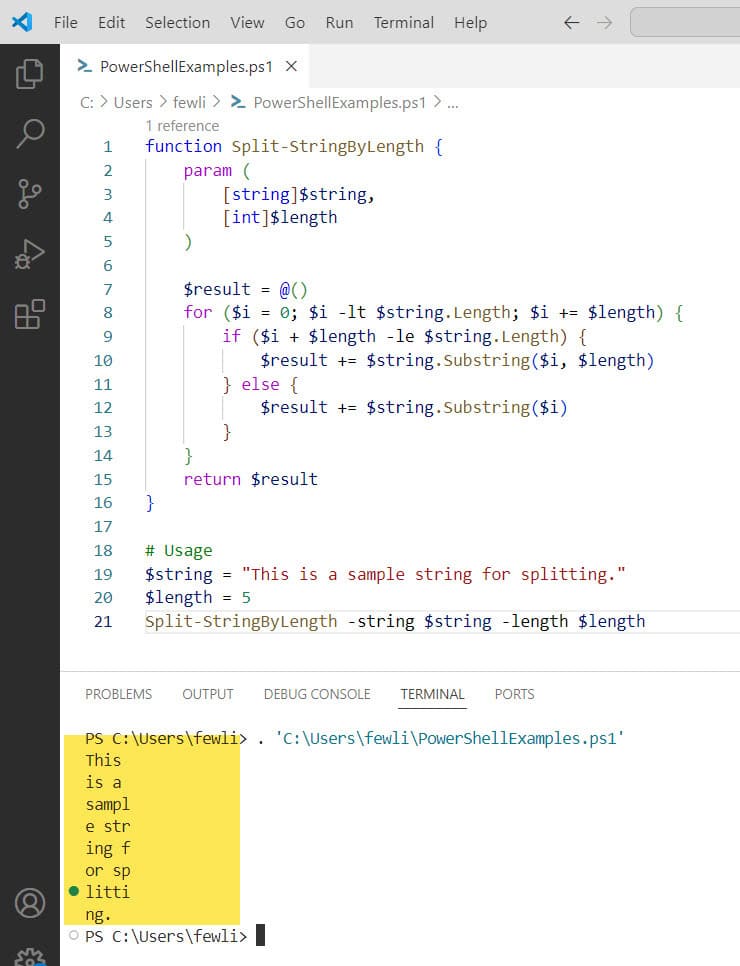
Method 2: Using Regular Expressions
Another method in PowerShell to split a string in PowerShell by length is to use regular expressions with the -match
operator or [regex]::Matches
method.
Here’s an example using [regex]::Matches
:
function Split-StringByLengthRegex {
param (
[string]$string,
[int]$length
)
$regex = ".{1,$length}"
$matches = [regex]::Matches($string, $regex)
$result = $matches | ForEach-Object { $_.Value }
return $result
}
# Usage
$string = "This is a sample string for splitting."
$length = 5
Split-StringByLengthRegex -string $string -length $length
In this function, we construct a regular expression pattern ".{1,$length}"
that matches any sequence of characters up to the specified length. The [regex]::Matches
method returns all matches, which we then extract and return as an array.
You can see the output in the screenshot below.
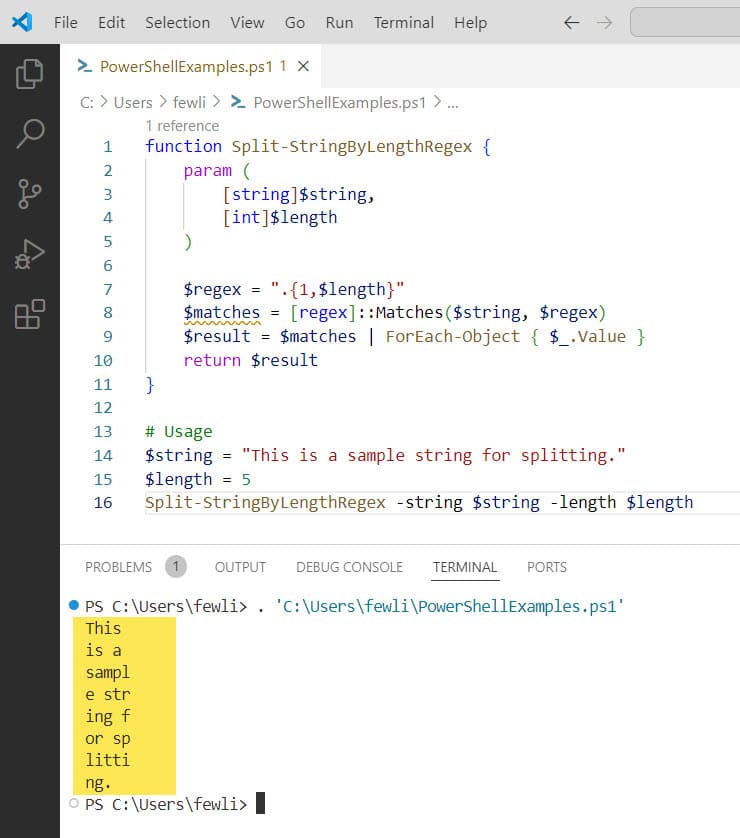
Method 3: Using a Custom Split Function
You can also write a custom function to split the string based on the desired length without using loops or regex.
Here’s how you can do it: follow the example below.
function Split-StringByLengthCustom {
param (
[string]$string,
[int]$length
)
$result = @()
$index = 0
while ($index -lt $string.Length) {
$chunk = $string.Substring($index, [math]::Min($length, $string.Length - $index))
$result += $chunk
$index += $length
}
return $result
}
# Usage
$string = "This is a sample string for splitting."
$length = 5
Split-StringByLengthCustom -string $string -length $length
In this function, we use a while
loop to iterate through the string and extract chunks of the specified length.
Conclusion
In this PowerShell tutorial, I have explained how to split a string by length in PowerShell using various methods with examples.
You may also like the following tutorials:
- Create SharePoint List & Add Columns Using PnP PowerShell
- Convert String to SecureString in PowerShell
- Convert String to Integer in PowerShell
- Convert Multiline String to Array in PowerShell
- Replace a String in Text File with PowerShell
- PowerShell Replace String Before Character
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com