This tutorial is everything about PowerShell Naming Conventions. Here, we’ll discuss the best ways to name things in PowerShell, like cmdlets, functions, and variables. Good naming helps make your scripts easy to understand and professional.
PowerShell naming conventions are critical in creating readable, maintainable, and consistently structured scripts. These conventions help users to intuitively understand the purpose of a function, commandlet, or variable, which is essential for collaboration and long-term code management.
PowerShell Naming Conventions – Basic Principles
PowerShell’s naming conventions are designed to provide clarity and consistency in script development. These conventions help to ensure that commands are self-descriptive and easily understood by users.
Verb-Noun Format
In PowerShell, cmdlets follow a Verb-Noun naming structure where the verb represents the action that the cmdlet performs, and the noun describes the entity upon which the action is performed. This strict pairing helps in predictably determining what a cmdlet does. For example, Get-Process
retrieves information about system processes.
Approved Verbs
PowerShell maintains a specific set of approved verbs to standardize cmdlet names and make them easily discoverable. These verbs are categorized by their intended effect, such as Get
for retrieval operations or Set
for modification tasks. To find the full list of approved verbs, one can use the command Get-Verb
.
Singular Nouns
Cmdlet names in PowerShell use singular nouns rather than plural. The use of singular nouns ensures that each PowerShell cmdlet name clearly refers to a single entity type that the verb acts upon. For example, Get-Command
retrieves a list of all commands available, even though it might return multiple items.
PowerShell Variable Naming Rules
Variable naming in PowerShell is significant for script clarity and maintainability. The following guidelines should ensure variables are easily identifiable and understandable.
Use Descriptive Names
Variables should have descriptive names that convey their purpose or content. For instance, a variable storing a list of user accounts could be named $userAccountsList
rather than something ambiguous like $list
.
Avoid Using Reserved Words
Variables should not use PowerShell reserved words or conflict with cmdlet names. For instance, avoid naming your variable $for
or $while
. A full list of reserved words can be found in the PowerShell documentation.
Consider Scope
When naming variables, one must consider their scope:
$local:VarName
for local scope$script:VarName
for script scope$global:VarName
for global scope$private:VarName
for private scope in a script or script block
Choose the scope that best suits the variable’s use to avoid unintended overwriting or access.
Prefix Type
It is a common practice to prefix a variable name with an abbreviation of its type when the type is critical to understand the context, although this is not a strict rule. For example:
Type | Prefix | Example |
---|---|---|
int | i | $iCounter |
string | str | $strName |
Capitalization Guidelines
Variable names in PowerShell are not case-sensitive, but it can be helpful to use a consistent capitalization pattern for readability. The popular choices are CamelCase ($userData
) or snake_case ($user_data
). Choose one style and apply it consistently across your scripts.
PowerShell Function and Cmdlet Naming Guidelines
In PowerShell, maintaining a consistent and clear naming convention for functions and cmdlets is essential for readability and usability. This involves careful verb selection, noun uniqueness, deliberate parameter naming, and appropriate cmdlet prefixes.
Verb Selection
The verb in a cmdlet name signifies the action the cmdlet performs. PowerShell recommends using standard verbs predefined by the Get-Verb
cmdlet to ensure consistency. A function or cmdlet should only use these approved verbs. For example, use Get-
for retrieval operations, Set-
for modification tasks, and New-
when creating resources.
Approved Verb | Action |
---|---|
Get | Retrieves information |
Set | Changes existing information |
New | Creates a new resource |
Noun Uniqueness
The noun in a cmdlet name identifies the entity on which the operation is performed. Nouns should be singular and specific to minimize ambiguity. They must not conflict with nouns from other modules unless they are directly related. For instance, if a cmdlet interacts with a specific service, include the service name in the noun.
Cmdlet | Noun | Description |
---|---|---|
Get-Process | Process | Refers to a single process instance |
Get-VMNetworkAdapter | VMNetworkAdapter | Targets a VM’s network adapter |
Parameter Naming
Cmdlet parameters should have clear, self-explanatory names that convey their purpose without additional context. Use PascalCase and avoid aliases which can obscure meaning. The parameter names should follow the noun-verb format where applicable and avoid using reserved parameter names like Debug
or ErrorAction
.
- Idempotent: Indicate actions that do not change system state.
- Force: Use for parameters that override restrictions or confirmations.
Cmdlet Prefixes
A cmdlet prefix usually abbreviates the module name and provides a namespace to avoid name collisions with cmdlets from other modules. For example, all cmdlets in the Active Directory
module start with AD
, like Get-ADUser
.
Module | Prefix | Example |
---|---|---|
Active Directory | AD | Get-ADUser |
Azure | Az | Get-AzVM |
Script and Module Naming Conventions in PowerShell
In PowerShell, following a consistent naming convention for scripts and modules enhances clarity and maintenance.
Use Consistent Suffixes
Scripts and modules should have appropriate suffixes to indicate their file type. For scripts, .ps1
is the standard suffix. Modules, on the other hand, should use .psm1
for script modules and .psd1
for module manifests. A table of file types and their suffixes is provided for reference:
File Type | Suffix |
---|---|
PowerShell Script | .ps1 |
Script Module | .psm1 |
Module Manifest | .psd1 |
Version Numbering
Including a version number in the name of a module is beneficial for managing updates and dependencies. The common practice is to append the version number at the end of the module name using the pattern ModuleName.V#.#.#
, where #.#.#
corresponds to the Major, Minor, and Patch version numbers as defined by Semantic Versioning.
Version Part | Purpose |
---|---|
Major | Indicates significant changes |
Minor | Adds backward-compatible features |
Patch | Fixes bugs without adding new features |
Including Verb-Noun Pair
PowerShell best practices dictate the use of a verb-noun pair for the naming of cmdlets, and this extends to script and module names as well. This scheme involves pairing an approved PowerShell verb with a specific noun to concisely convey the script or module’s purpose. For instance, Get-InventoryReport.ps1
immediately informs the user that the script retrieves an inventory report.
- Approved PowerShell Verb: Provides clarity on the action performed.
- Specific Noun: Identifies the target or subject of the action.
PowerShell File and Folder Naming Practices
Effective file and folder naming in PowerShell is essential for clarity, consistency, and avoiding errors during script execution. These practices pertain to naming conventions within PowerShell scripts and the use of PowerShell for managing file systems.
Character Restrictions
In PowerShell, certain characters are prohibited in file and folder names due to their special meaning within the file system or the PowerShell language itself. The characters not allowed are:
< (less than)
> (greater than)
: (colon)
" (double quote)
/ (forward slash)
\ (backslash)
| (vertical bar or pipe)
? (question mark)
* (asterisk)
Additionally, it’s also important to avoid trailing spaces or periods for compatibility with Windows file systems.
Path Length Considerations
PowerShell and the Windows file system traditionally impose a maximum path length limit of 260 characters. This includes the drive letter, colon, backslash, folder names, the file name, and the file extension. Care must be taken to structure directories and name files such that the total character count remains within this limit to prevent errors.
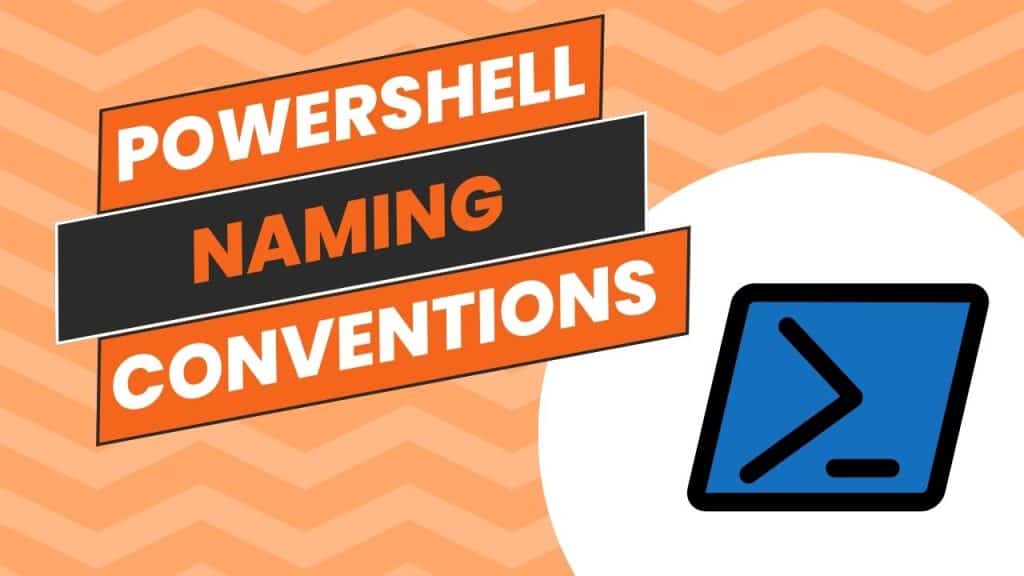
Advanced Naming Concepts in PowerShell
In PowerShell, advanced naming concepts provide script clarity and maintainability, especially as the complexity increases. These conventions establish a common language for developers to follow, ensuring that function purpose, scope, and type are immediately evident.
Using Pseudo-Hungarian Notation
Pseudo-Hungarian Notation incorporates a variable’s data type or purpose into its name. For instance:
- Strings may start with
str
, as instrUsername
- Integers might begin with
int
, as inintCount
- Booleans often use
is
,has
,can
, indicating a condition such asisFound
,hasItems
When used sparingly and appropriately, this approach can make the code easier to understand.
Distinguishing Private and Public Functions
PowerShell supports both public and private functions in scripts and modules. Naming conventions help to differentiate them.
- Public Functions: These follow a Verb-Noun format and are intended for external use, such as
Get-Inventory
- Private Functions: These are for internal script/module use only. A common practice is prefixing names with an underscore, like
_ResolvePath
Naming Conventions for Constants
In PowerShell, constants are variables whose values do not change. They are typically declared with [const]
and written in uppercase letters to stand out, a widespread convention across programming languages.
- Examples:
MAX_RETRIES
,DEFAULT_TIMEOUT
Naming constants clearly and in uppercase serves as an immediate identifier of their immutable nature.
Conclusion
PowerShell scripting benefits greatly from consistent naming conventions, lending script clarity and predictability. In practice, they ensure scripts are:
- Easier to understand: Familiar patterns guide users through the script’s logic.
- Maintainability: Consistency allows for simpler updates and troubleshooting.
- Collaboration friendly: Teams can work together seamlessly when following a common standard.
Adhering to the established conventions guarantees that scripts will be readily comprehensible not just to their authors, but also to other users and administrators. These best practices, such as Verb-Noun pairings for cmdlets and the use of PascalCase for function names, are critical for creating professional-grade scripts. Moreover, PowerShell’s flexibility in aliasing commands and the existence of approved verbs lists from Microsoft further streamline the scripting process.
For variable names, concise yet descriptive identifiers are imperative, following camelCase to distinguish local variables and PascalCase for global ones. One must avoid using reserved words and ensure that advanced functions utilize CmdletBinding to leverage cmdlet behaviors.
Finally, comment-based help accompanying functions and scripts encapsulate the essence of PowerShell’s self-documenting nature, solidifying understanding and usage across diverse environments.
I hope now you have a complete idea of PowerShell naming conventions.
You may also like:
- PowerShell Functions
- PowerShell Array Tutorial
- PowerShell ArrayList
- PowerShell Reference Variable
- PowerShell Variable Naming Conventions
- PowerShell try catch with examples
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com