In this tutorial, I will explain what is CSOM in SharePoint and how to work with SharePoint CSOM with a few microsoft.sharepointonline.csom examples.
What is CSOM in SharePoint?
CSOM stands for SharePoint client object model and enables developers to interact with SharePoint data and services from C# client-side applications. CSOM allows applications to talk to SharePoint from the client side, which can be a web browser or a client application. This is useful when you cannot or do not want to deploy custom code directly to the SharePoint server.
SharePoint makes CSOM available in multiple languages and libraries, including .NET, Silverlight, and JavaScript, so developers can use it in different types of applications.
With CSOM, you can do a lot of operations in SharePoint, such as creating, reading, updating, and deleting SharePoint list items, working with files, managing SharePoint sites, accessing user profiles, and much more.
CSOM communicates with SharePoint via a web service. The client application uses CSOM to send XML or JSON data requests to the SharePoint server, which processes these requests and sends back the appropriate responses.
You can write the CSOM code using a C#.net desktop or console application in Visual Studio.
SharePoint Online csom download
To work with .Net client object model, we need the below two dlls.
- Microsoft.SharePoint.Client.dll
- Microsoft.SharePoint.Client.Runtime.dll
So, in the Asp.Net application or Windows or console application, we need to add these two dlls.
Based on the functionalities you will use, you will have to add the other dlls like:
- Microsoft.SharePoint.Client.DocumentManagement.dll
- Microsoft.SharePoint.Client.Publishing.dll
- Microsoft.SharePoint.Client.Taxonomy.dll
- Microsoft.SharePoint.Client.UserProfiles.dll
- Microsoft.SharePoint.WorkflowServices.Client.dll
Since SharePoint is not installed on the system by default, there are other ways to get or add these two dlls to the .net application.
We can download and install SharePoint client components sdk in the system. Based on the SharePoint version, you can download SharePoint client components SDK.
- Download SharePoint Online client components SDK
- Download SharePoint server 2013 client components SDK
- Download SharePoint server 2016 client components SDK
Once you download and install SharePoint Online client components sdk, you can see all the client-side dlls, in the below directory.
C:\Program Files\Common Files\microsoft shared\Web Server Extensions\16\ISAPI
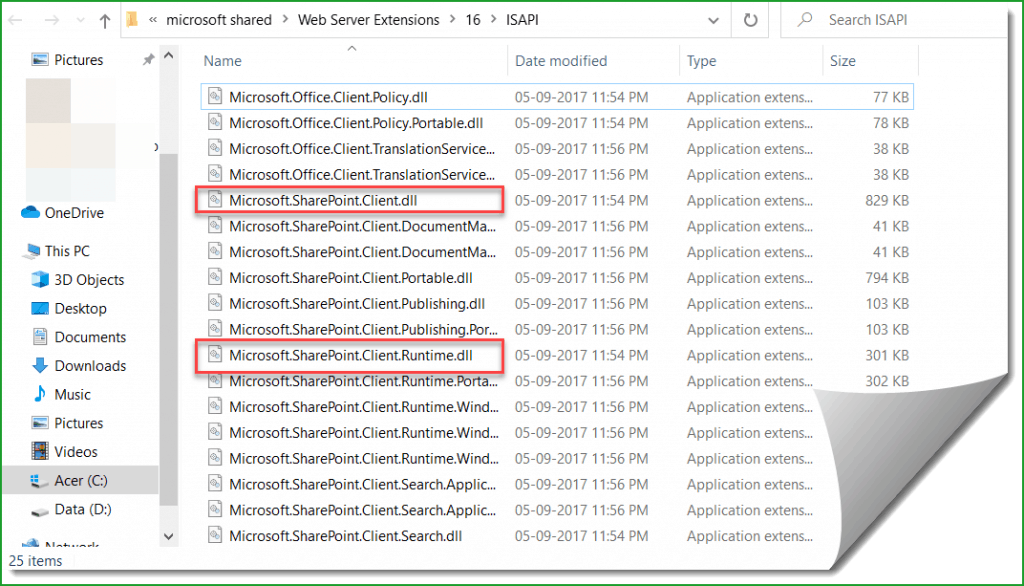
CSOM SharePoint Online console application
Now, let us see how to create a console application using Visual Studio for CSOM SharePoint Online.
Open Visual Studio 2019 and then click on Create a New Project. Then, in the Create a new project, search for console and then choose Console App (.NET Framework). Then click on Next.
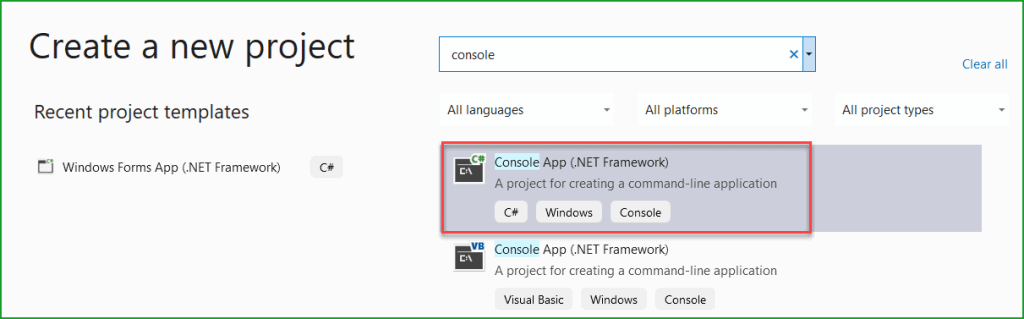
Then, in the Configure your new project, provide the details like:
- Project name
- Location (You can choose the default location)
- Solution name
- Framework – Select the .NET Framework.
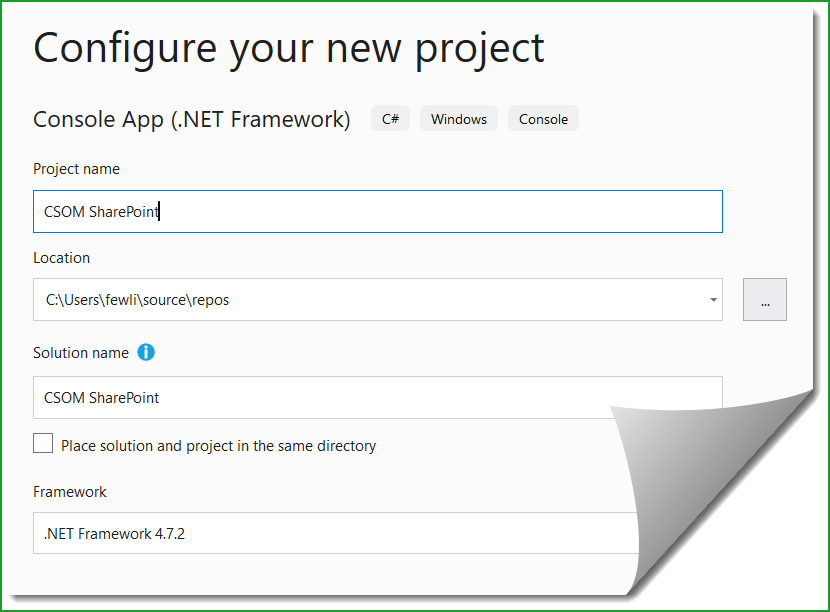
Then click on the Create button.
Then, it will create the console application, and you can see the solution explorer below. Here, we can write the code in the Program.cs file.
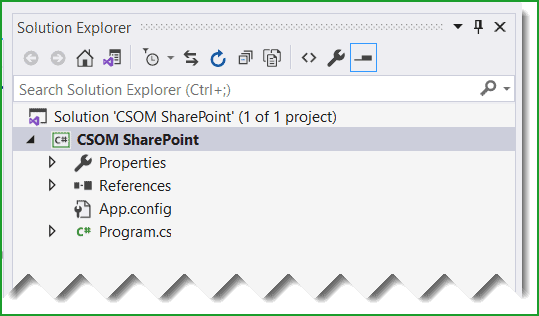
Here, the first thing we need to do is to add the below two dlls into the solution.
- Microsoft.SharePoint.Client.dll
- Microsoft.SharePoint.Client.Runtime.dll
As I explained above, if you have already installed SharePoint Online client component SDK, you can provide a reference from the ISAPI folder.
Right-click on the References folder and then click on Add Reference… Then browse to the ISAPI folder and then select the above two dlls.
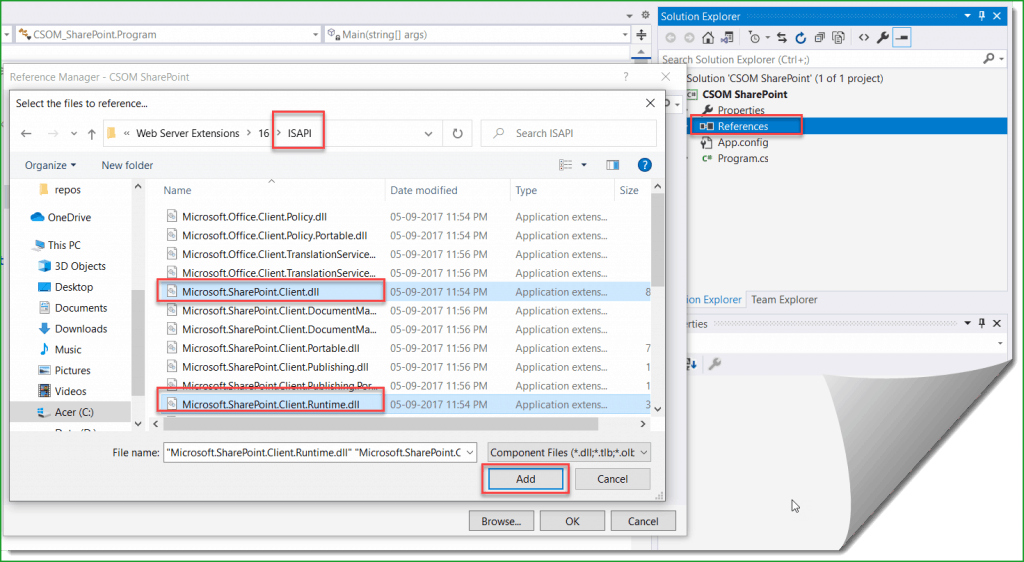
If you have not installed SharePoint online client sdks, then you can also add the dlls from the Nuget package manager.
CSOM SharePoint Online nuget
Now, let us see how to add the client dlls from Nuget.
Right-click on the References folder, then click on Manage NuGet Packages…
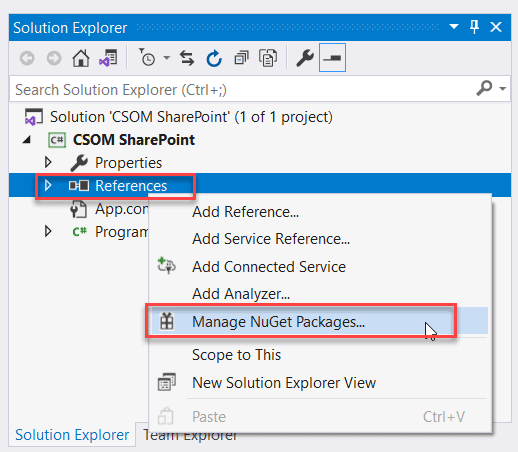
Then go to the Browse tab and then search for Microsoft.SharePointOnline.CSOM, and you will see the dll from Microsoft, select it then click Install.
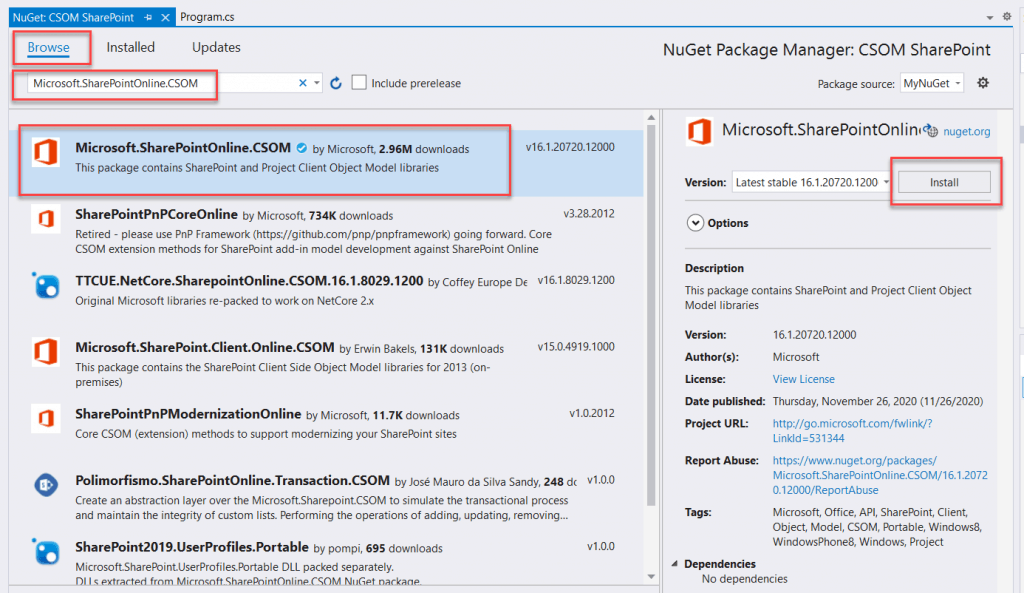
Accept the license agreement, and then it will install all the client dlls. All the references are below.
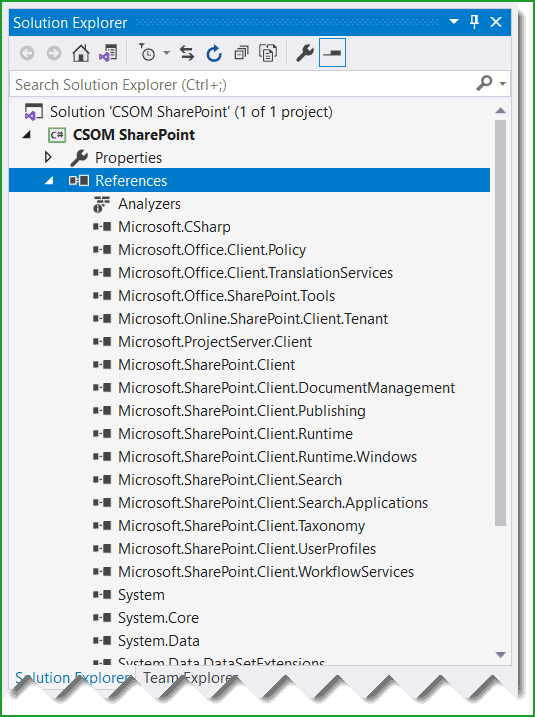
You can also install the SharePoint Online CSOM Nuget package from the command prompt.
Install-Package Microsoft.SharePointOnline.CSOM -Version 16.1.20720.12000
Now we can write the code using CSOM SharePoint online.
microsoft.sharepointonline.csom examples or SharePoint CSOM Examples
Now, let us check out a few microsoft.sharepointonline.csom examples.
Example-1: Get WebSite Title
To connect with a SharePoint Online site from the CSOM SharePoint console application, we need to add the username and password to the SharePointOnlineCredentials.
Here, the password will be a secure password; you can pass it like a string.
The first thing you need to do is two add the below using statements:
using System.Security;
using Microsoft.SharePoint.Client;
To get sharepoint online site context using csom, we can use the ClientContext class like:
ClientContext ctx = new ClientContext("https://tsinfo.sharepoint.com/sites/SPGuides/")
ctx.AuthenticationMode = ClientAuthenticationMode.Default;
The complete code looks like below:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security;
using System.Text;
using System.Threading.Tasks;
using Microsoft.SharePoint.Client;
namespace CSOM_SharePoint
{
class Program
{
static void Main(string[] args)
{
using (ClientContext ctx = new ClientContext("https://tsinfo.sharepoint.com/sites/SPGuides/"))
{
ctx.AuthenticationMode = ClientAuthenticationMode.Default;
SecureString securePassword = new SecureString();
foreach (char c in "YourPassword")
securePassword.AppendChar(c);
ctx.Credentials = new SharePointOnlineCredentials("bijay@tsinfo.onmicrosoft.com", securePassword);
}
}
}
}
This is how we can authenticate csom sharepoint online console application. This is how the csom sharepoint online authentication works.
I have added a simple csom sharepoint online example to get the properties of the SharePoint website. Here, I have retrieved the website title.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security;
using System.Text;
using System.Threading.Tasks;
using Microsoft.SharePoint.Client;
namespace CSOM_SharePoint
{
class Program
{
static void Main(string[] args)
{
using (ClientContext ctx = new ClientContext("https://tsinfo.sharepoint.com/sites/SPGuides/"))
{
ctx.AuthenticationMode = ClientAuthenticationMode.Default;
SecureString securePassword = new SecureString();
foreach (char c in "YourPassword")
securePassword.AppendChar(c);
ctx.Credentials = new SharePointOnlineCredentials("bijay@tsinfo.onmicrosoft.com", securePassword);
Web web = ctx.Web;
ctx.Load(web);
ctx.ExecuteQuery();
Console.WriteLine("The web site title: "+web.Title);
Console.Read();
}
}
}
}
Once you run the csom sharepoint online console application, you can see the output like below:
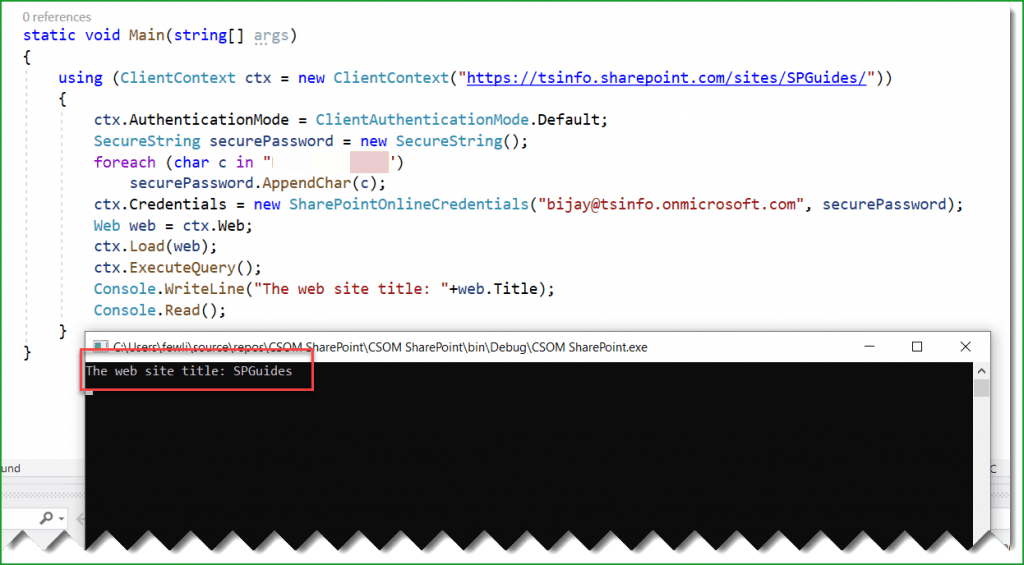
Here, we learned how to get sharepoint online site context using csom and how the csom sharepoint online authentication works.
Here, we need to understand the two important methods of CSOM SharePoint Online.
Load() method
The SharePoint CSOM Load() method does not actually retrieve anything, it just loads the properties only when the ExecuteQuery() method is called does it provide notification that these are the property values that should be loaded for the object.
ExecuteQuery() method
The SharePoint CSOM ExecuteQuery() method sends the request to the server. There is no network traffic until the application calls this method. Until the ExecuteQuery() method is called only the requests are registered by the application.
Example-2: Create site collection using SharePoint CSOM
Below is the complete code to create a SharePoint Online collection using CSOM.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Online.SharePoint.TenantAdministration;
using Microsoft.SharePoint.Client;
namespace CSOM_SharePoint
{
class Program
{
static void Main(string[] args)
{
using (ClientContext ctx = new ClientContext("https://tsinfo-admin.sharepoint.com/"))
{
ctx.AuthenticationMode = ClientAuthenticationMode.Default;
SecureString securePassword = new SecureString();
foreach (char c in "MyPassword")
securePassword.AppendChar(c);
ctx.Credentials = new SharePointOnlineCredentials("bijay@tsinfo.onmicrosoft.com", securePassword);
var tenant = new Tenant(ctx);
var siteCollectionProperties = new SiteCreationProperties();
siteCollectionProperties.Url = "https://tsinfo.sharepoint.com/sites/NewSPGuideSite";
siteCollectionProperties.Title = "New SPGuides Site";
siteCollectionProperties.Owner = "bijay@tsinfo.onmicrosoft.com";
siteCollectionProperties.Template = "STS#3";
SpoOperation spo = tenant.CreateSite(siteCollectionProperties);
ctx.Load(tenant);
ctx.ExecuteQuery();
Console.WriteLine("SharePoint site collection created successfully!");
Console.Read();
}
}
}
}
Now, when you open the SharePoint Online admin center, you can see the newly created site collection under Active sites like below:
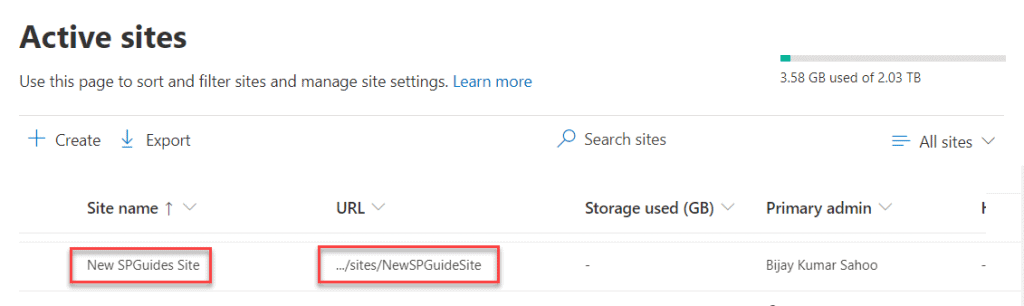
This is how to create a site collection using CSOM in SharePoint Online.
Example-3: Create a communication site in SharePoint online using CSOM
Now, let us see how to create a communication site collection in SharePoint Online using CSOM. The steps to create a communication site are similar to creating a team site in SharePoint Online. The only difference is the site template ID.
The SharePoint Online communication site template id is: SITEPAGEPUBLISHING#0
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Online.SharePoint.TenantAdministration;
using Microsoft.SharePoint.Client;
namespace CSOM_SharePoint
{
class Program
{
static void Main(string[] args)
{
using (ClientContext ctx = new ClientContext("https://tsinfo-admin.sharepoint.com/"))
{
ctx.AuthenticationMode = ClientAuthenticationMode.Default;
SecureString securePassword = new SecureString();
foreach (char c in "YourPassword")
securePassword.AppendChar(c);
ctx.Credentials = new SharePointOnlineCredentials("bijay@tsinfo.onmicrosoft.com", securePassword);
var tenant = new Tenant(ctx);
var siteCollectionProperties = new SiteCreationProperties();
siteCollectionProperties.Url = "https://tsinfo.sharepoint.com/sites/NewCommunicationSite";
siteCollectionProperties.Title = "New Communication Site";
siteCollectionProperties.Owner = "bijay@tsinfo.onmicrosoft.com";
siteCollectionProperties.Template = "SITEPAGEPUBLISHING#0";
SpoOperation spo = tenant.CreateSite(siteCollectionProperties);
ctx.Load(tenant);
ctx.ExecuteQuery();
Console.WriteLine("SharePoint Communication site collection created successfully!");
Console.Read();
}
}
}
}
Once you execute the code, the SharePoint communication site is created successfully using CSOM SharePoint Online.
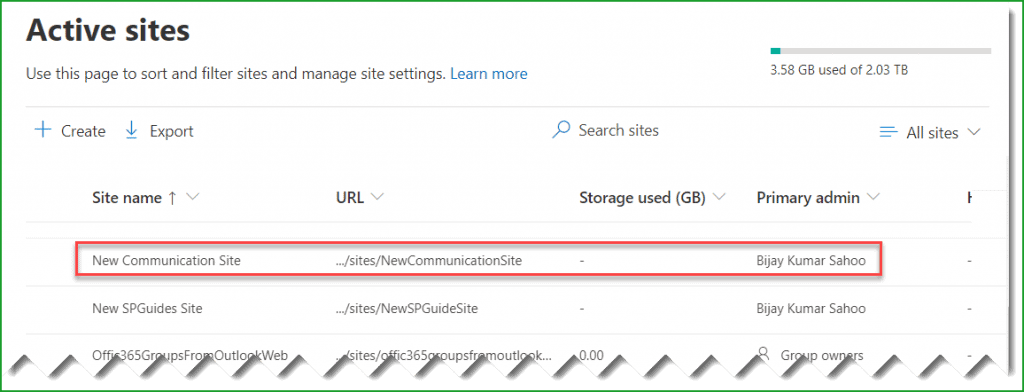
Example-4: Get all SharePoint Site Collections using CSOM
Now, let us see how to get all site collections using SharePoint CSOM.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Online.SharePoint.TenantAdministration;
using Microsoft.SharePoint.Client;
namespace CSOM_SharePoint
{
class Program
{
static void Main(string[] args)
{
using (ClientContext ctx = new ClientContext("https://tsinfo-admin.sharepoint.com/"))
{
ctx.AuthenticationMode = ClientAuthenticationMode.Default;
SecureString securePassword = new SecureString();
foreach (char c in "YourPassword")
securePassword.AppendChar(c);
ctx.Credentials = new SharePointOnlineCredentials("bijay@tsinfo.onmicrosoft.com", securePassword);
var tenant = new Tenant(ctx);
SPOSitePropertiesEnumerable siteProps = tenant.GetSitePropertiesFromSharePoint("0", true);
ctx.Load(siteProps);
ctx.ExecuteQuery();
Console.WriteLine("Total Site Collections: " + siteProps.Count.ToString());
foreach (var site in siteProps)
{
Console.WriteLine(site.Title + "\t" + site.Template.ToString());
}
Console.ReadLine();
}
}
}
}
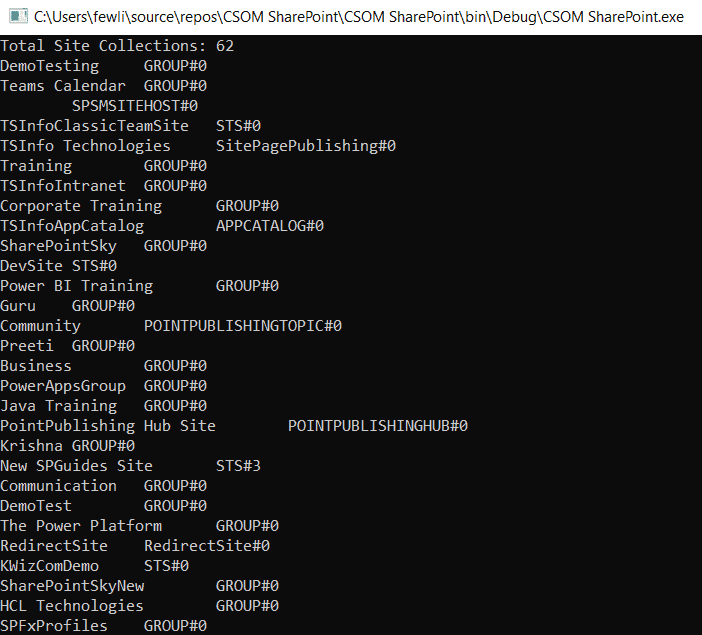
Example-5: Delete SharePoint List Programmatically using CSOM
Now, let us see how to delete a SharePoint list programmatically c# (CSOM).
Below is the C#.net method to delete the sharepoint list programmatically using c#.
public void DeleteTemporaryList(string URL)
{
using (ClientContext clientContext = new ClientContext(URL))
{
clientContext.AuthenticationMode = ClientAuthenticationMode.Default;
clientContext.Credentials = new SharePointOnlineCredentials(GetSPOAccountName(), GetSPOSecureStringPassword());
List tempList;
try
{
tempList = clientContext.Web.Lists.GetByTitle("MyTempList");
clientContext.Load(tempList);
clientContext.ExecuteQuery();
tempList.DeleteObject();
clientContext.ExecuteQuery();
}
catch (Exception ex)
{
}
}
}
private static string GetSPOAccountName()
{
try
{
return ConfigurationManager.AppSettings["SPOAccount"];
}
catch
{
throw;
}
}
private static SecureString GetSPOSecureStringPassword()
{
try
{
var secureString = new SecureString();
foreach (char c in ConfigurationManager.AppSettings["SPOPassword"])
{
secureString.AppendChar(c);
}
return secureString;
}
catch
{
throw;
}
}
This is how to delete a list programmatically using CSOM C#.Net managed object model code.
Example-6: SharePoint Online copy list items to another list programmatically (C#.net)
Let us see how to copy list items to another list programmatically using CSOM.
Here, I have a list named SourceList, which has 3 columns like the below:
- Title (Single line text)
- EmailID (Single line text)
- Address (Multiple line text)
It has a few items, and the list looks like below:
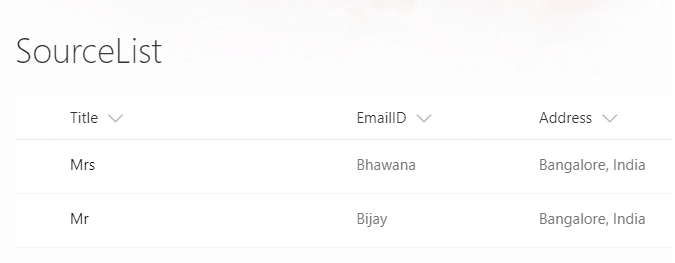
Here, we will move these items to another list on the same site, which has the same column names.
Below is the code to copy SharePoint list items to another list programmatically using C#.Net.
public static void CopyItemsFromOneListToAnotherList()
{
using (ClientContext ctx = new ClientContext(“https://<tenant-name>.sharepoint.com/sites/Finance/”))
{
ctx.AuthenticationMode = ClientAuthenticationMode.Default;
ctx.Credentials = new SharePointOnlineCredentials(GetSPOAccountName(), GetSPOSecureStringPassword());
ctx.Load(ctx.Web);
ctx.ExecuteQuery();
List sourceList= ctx.Web.Lists.GetByTitle(“SourceList”);
ctx.Load(sourceList);
ctx.ExecuteQuery();
List destList = ctx.Web.Lists.GetByTitle(“DestinationList”);
ctx.Load(sourceList);
ctx.ExecuteQuery();
CamlQuery camlQuery = new CamlQuery();
camlQuery.ViewXml = “<View/>”;
ListItemCollection listItems = sourceList.GetItems(camlQuery);
ctx.Load(listItems);
ctx.ExecuteQuery();
foreach (ListItem item in listItems)
{
ListItemCreationInformation newItemInfo = new ListItemCreationInformation();
ListItem newItem = destList.AddItem(newItemInfo);
newItem[“Title”] = item[“Title”];
newItem[“EmailID”] = item[“EmailID”];
newItem[“Address”] = item[“Address”];
newItem.Update();
}
ctx.ExecuteQuery();
}
}
private static string GetSPOAccountName()
{
try
{
return ConfigurationManager.AppSettings[“SPOAccount”];
}
catch
{
throw;
}
}
private static SecureString GetSPOSecureStringPassword()
{
try
{
var secureString = new SecureString();
foreach (char c in ConfigurationManager.AppSettings[“SPOPassword”])
{
secureString.AppendChar(c);
}
return secureString;
}
catch
{
throw;
}
}
Once you run the above code, it will copy the items below:
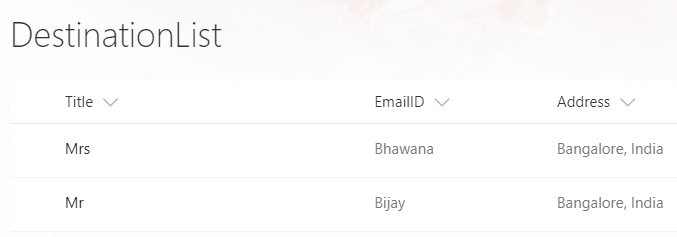
This is how to copy list items to another list programmatically using CSOM in SharePoint.
Conclusion
I hope you can now work with CSOM SharePoint as a SharePoint developer. We saw a few useful SharePoint CSOM examples with C#.
You may also like:
- SharePoint Calculated Column
- SharePoint Column Validation Formula Examples
- SharePoint Rest API
- SharePoint Server Object Model Tutorial
- JavaScript Object Model
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com