In this React js tutorial, we will see all about props in React js, so,
- What are props in React js
- why we use props in react js
- Props in React js functional component
- props in React js Class component
- react default props
- What are render props in react js
- React js props type
- props.children in react js
- how to pass multiple props in react js
- conditional props in react js
- react js destructuring props
What are props in React js?
In React js, props are arguments sent into React component. Props are sent to components through Html attributes. And props stand for properties.
Props are nothing but an object which stores the value of attributes of a tag and works similarly to Html attributes. It provides a way to send data from one component to another. It is just like a functional argument because props are passed to the react component in an exact way as an argument given in a function.
In React js props are immutable/unchangeable, so we cannot modify the props inside the React component. We can add attributes, inside the React component called props. With this. props contain all the attributes in the react component, and with the render method, we can render dynamic data.
The syntax for the functional component
function Welcome(props) {
return <h1>Hii, {props.name}</h1>;
}
The syntax for the class component
class Welcome extends React.Component {
render() {
return <h1>Hii, {this.props.name}</h1>;
}
}
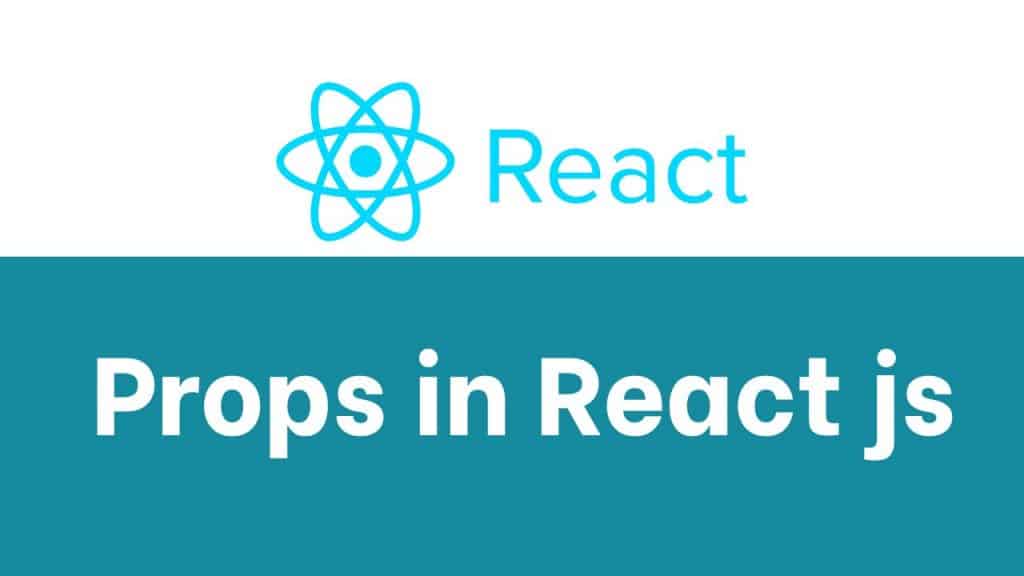
Read What is JSX in React js
Why we use props in react js
In React js, we use props to send the data from one component to another component, i.e from parent component to child component. These are useful when dynamically you want to flow the data in the react app.
Props in React js functional component
To access props in the Functional component, we don’t need to use the ‘this’ keyword, because in React Functional component accepts props as parameters and can be accessed directly.
Example of props in React js functional component
Let’s say index.html contains the below statement inside the body tag:
<div id="root"></div>
Next in the index.js file write the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
function Welcome(props){
return <h1>Hello, {props.name}</h1>
}
const root = ReactDOM.createRoot(document.getElementById('root'));
const elem= <Welcome name ="Alia"></Welcome>
root.render(elem);
So the above code renders Hello, Alia on the page:
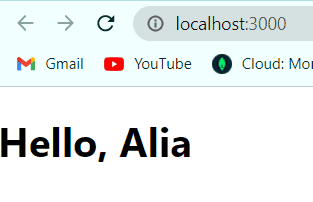
This is how we can set props in React js functional component
Read State in React js Hooks
Props in React js Class component
We can access props in the ES6 class component using the ‘this’ keyword i.e. this.props.name. This. prop is a type of global object which have all component props. And the propName is the props name that is the key of the object.
Example of props in React js Class component
Let’s say the index.html file contains the below statement inside the body tag:
<div id="root"></div>
And in the index.js file write the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
class Welcome extends React.Component {
render() {
return <h1>Welcome, {this.props.name}</h1>;
}
}
const root = ReactDOM.createRoot(document.getElementById('root'));
const elem= <Welcome name ="Alia"></Welcome>
root.render(elem);
So the above code renders ‘Welcome Alia’ on the page.
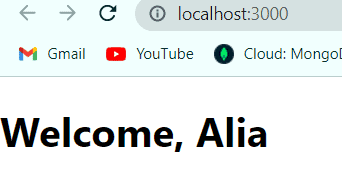
This is how we can set props in React js Class component.
Read Components in React js
React default props
Here we will see how to set default props in React js.
We will set the defaultProps property when a component is rendered. but the prop is not specified.
Default props are beneficial while you need to have a default prop in case the person doesn’t specify one. And we can declare defaultProps within a React class component as a static property.
In other words, we can say, that if the properties are not specified by the parent component then defaultProps will be used to verify that this.props.name will contain a value.
Example of React defaultProps
Let’s say the index.html file contains the below statement inside the body tag:
<div id="root"></div>
And in the index.js file write the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
class Welcome extends React.Component {
render() {
return ( <div><h1>Welcome, {this.props.name}</h1>
<h1> Designation is: {this.props.designation}</h1>
<h1>Department: {this.props.department} department</h1>
</div>
);
}
}
Welcome.defaultProps ={
name:'Alia',
designation:"Software Engineer",
department:"CS"
};
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(< Welcome />)
So the above code renders the default value on the page:
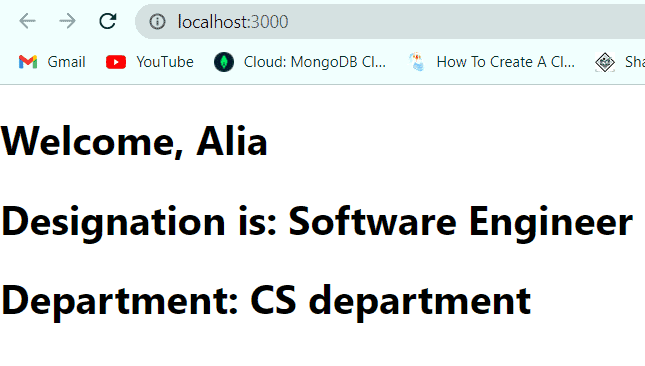
This is how we can set the React default props in React js.
Read What is react js and react native
React js props type
Here we will see all about React js props type.
What are propTypes?
PropTypes is used to check that the passed value in props is of the correct data type. These propTypes ensure that we don’t receive an error from the console at the end of the app, which may not be easy to debug.
Different types of propTypes
Here we will see different types of propTypes available in React js:
Basics types of propTypes
The basic way to check a propTypes is by checking if it comes under the category of primitive types in JS, such as boolean, string, object, and so on. Here are these:
PropTypes | Syntax | Example |
---|---|---|
String | PropTypes.string | ‘hello’ |
Object | PropType.object | {name: “Rohit”} |
Boolean | PropType.bool | True/false |
Number | PropType.number | 12 |
Function | PropType.func | const message={console.log(“hello”)} |
Symbol | PropType.symbol | Symbol(‘n’) |
Collective Type
Here we will discuss many more ways that props can be passed and used like collective types like an array number, strings, and so on.
Array types
PropTypes | Syntax | Example |
---|---|---|
Array | propTypes.Array | [] |
Array of objects | propTypes.oneOfTypes([type]) | PropTypes.string, Prop.Types.instanceOf(Title) |
Array of numbers | propTypes.arrayOf([type]) | [1,2,3,4] |
Array of strings | propTypes.oneOf([arr]) | [“Alia”, “Alex”,”David”] |
Object Types
Alike the array types, here is the list of the collection object types.
propTypes | Syntax | Example |
---|---|---|
Object | PropTypes.object | {name:’Ali’} |
Number of Objects | propTypes.objectOf() | {age: 25} |
Shape of objects | propTypes.Shape() | { name: propTypes.string, phone: propTypes.string } |
Instance | PropTypes.objectOf() | new message() |
How to use propTypes
Since, the release of React 15.5, the propTypes are available in the React package. But now we need to add a prop-types library to our project.
To install the prop-types library use the below command in the terminal.
npm install prop-types save
As we know propTypes is used to validate any data which we receive in props. But to use in propTypes, we need to import as always in our app:
import PropTypes from 'prop-types';
Example of propTypes
So here we will take the customer class and we will define two properties’ name(string) and age(number).
Let’s say the index.html file contains the below statement inside the body tag:
<div id="root"></div>
And in the index.js file write the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import PropTypes from 'prop-type'
class Greeting extends React.Component {
render() {
return (
<>
<h1>Hello, {this.props.customer.name}</h1>
<h1>Age is {this.props.customer.age}</h1>
</>
);
}
}
var customer ={
name: "Alex",
age:25
};
Greeting.propTypes ={
name: PropTypes.string,
age: PropTypes.number
};
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<Greeting customer={customer} />);
Now you can see the above code renders the Customer details on page.
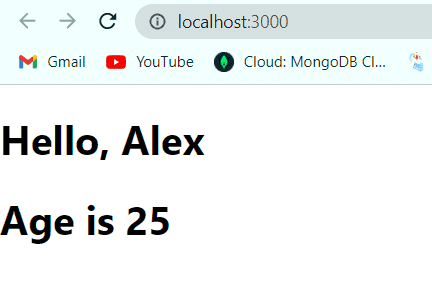
This is all about propTypes in React js.
Read React js form tutorial
What are render props in React js?
A render prop is an approach in React js to share code between React components with the help of a Prop whose value is a function. In React js Child component accepts render props as a function and calls it rather than executing its own render logic.
Example of render props in react js
Let’s say the index.html file contains the below statement inside the body tag:
<div id="root"></div>
And in the index.js file write the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
Next, in App.js write the below code, and App is the default component:
import './App.css';
import React from 'react';
const name= "Alex";
class App extends React.Component {
render() {
return (
<div>
<h1>Hey {name}</h1>
<Greeting />
</div>
)
}
}
// Child component getting render props
class Messages extends React.Component {
render() {
return (
<div>
<h2>Welcome to React js</h2>
{this.props.render()}
</div>
)
}
}
// This Parent component sending render props to the child
class Greeting extends React.Component {
render() {
return (
<Messages
// Passing render props to the child component
render={() => {
return (
<div>
<h3>
Thank You for choosing React js
</h3>
</div>
)
}}
/>
)
}
Read State in React js
Props.children in react js
Here we will discuss all props. children in React.js with an example.
What are props.children in react js?
In React js we can use props. children are used to accessing and using what we place inside the open and closing tag when you create an instance of a component.
Example of props.children in react js
Let’s say the index.html file contains the below statement inside the body tag:
<div id="root"></div>
And index.js file contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
Next, create a file with the name profile.js file, so here we will call the props value and child component/ HTML element to render dynamically.
function Profile(props){
console.log(props)
return<h1> name : {props.name}
{props.children}
</h1>
}
export default Profile;
Next in the App.js file, we imported the profile.js and assign the properties, and create a child component or HTML element inside the parent component.
import './App.css';
import Profile from './Components/Profile';
function App() {
return (
<div className="App">
<Profile name="Alex">
{/* child props */}
<h2> This is my profile</h2>
</Profile>
</div>
);
}
export default App;
Now you can see the above code render on the page:
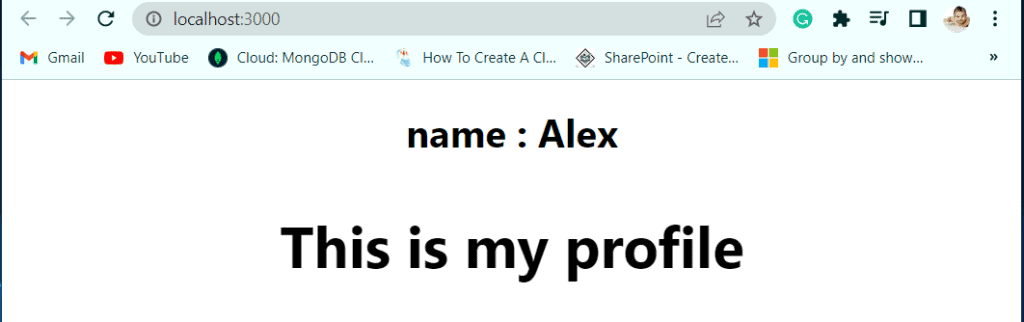
How to pass multiple props in react js
Here we will see an example of how to pass multiple props in react js.
For example, we have two properties first and last names, so we have to pass these two properties from one component to another.
Let’s say the index.html file contains the below statement inside the body tag:
<div id="root"></div>
And index.js file contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
Next, create a file with the name profile.js file, so here we will call the multiple props value (prop. name and props.lastName) and child component/ HTML element to render dynamically.
function Profile(props){
console.log(props)
return<h1> name : {props.name} {props.lastName}
{props.children}
</h1>
}
export default Profile;
Next in the App.js file, we imported the profile.js and assign values to the properties and create a child component or HTML element inside the parent component.
import './App.css';
import Profile from './Components/Profile';
function App() {
return (
<div className="App">
<Profile name="Alex" lastName= "Cubon">
{/* child props */}
<h2> This is my profile</h2>
</Profile>
</div>
);
}
export default App;
Now the above codes get rendered on the page.
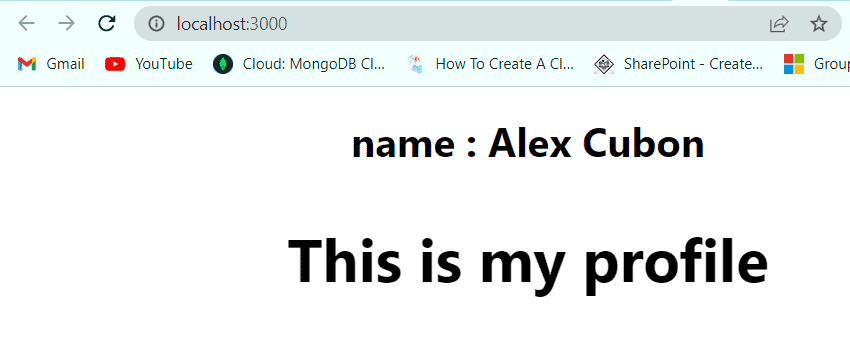
Conditional props in react js
Here we will see how to do conditional rendering using props in React js.
For example, we will use isLoggedIn prop, if the user is new then it shows the message “Please Sign up and if the user is existing then it will show “Welcome back”.
Let’s say the index.html file contains the below statement inside the body tag:
<div id="root"></div>
And then we will create one component to show greetings for users in the UserGreeting.js file and export it to the index.js file.
function UserGreeting(props) {
return <h2>Welcome back!</h2>
}
export default UserGreeting
Next, we will create another component to show greetings for the user GuestGreetings.js file and export it to the index.js file.
function GuestGreetings(props) {
return <h1>Please sign up.</h1>;
}
export default GuestGreetings
Next, in the index js file, we will import both the components and set the properties as true/false, to render the components on the page.
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import UserGreeting from './Components/UserGreeting'
import GuestGreetings from './Components/GuestGreetings';
function Greeting(props) {
const isLoggedIn = props.isLoggedIn;
if (isLoggedIn) {
return <UserGreeting />;
}
return <GuestGreetings />;
}
const root = ReactDOM.createRoot(document.getElementById('root'));
// Try changing to isLoggedIn={false}:
root.render(<Greeting isLoggedIn={true} />);
Now you can see the result on the page based on the prop value, as it is set to true, so it will render “Welcome back”.
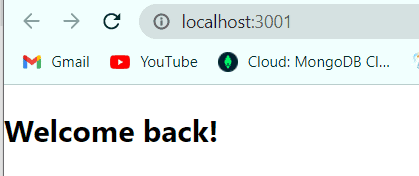
React js destructuring props
Here we will discuss all destructuring of props in React js.
What is destructuring?
- In Javascript is destructing is a characteristic that is used to take out sections of data from an array of objects, then we can assign them to a new variable.
- Destructing does not change an object or an array, it just makes a copy of the desired object or array element by storing them in a new variable. Later we can use this new variable in React component.
- When we want to access the props we use this keyword, we use this.props throughout the code, this is called restructuring, but with restructuring, we can remove this.prop by assigning them to a new variable. It is very hard to get the props in complex applications, so by assigning them to a new variable, we can make code easy to understand and readable.
Advantages of Destructuring
The advantage of using destructuring in React js:
- Assigning a new variable makes the developer’s life easy.
- Nested data is not simple it takes time to access, but with destructuring, we can access nested data easily.
- It improves the legibility and maintenance of code.
- It reduces the amount of code used in an application.
- It reduces the steps taken to access the data properties
- It optimizes the iteration over an array of objects multiple times
- With destructuring, we can improve the legibility of the ternary operator, as we are using the ternary operator multiple times in the render method.
How to use Destructuring in React js?
Here we will see how to use destructing in React js.
Example of Destructuring in React js
Let’s say the index.html file contains the below statement inside the body tag:
<div id="root"></div>
Next, write the below code in the App.js file, and the App is the default component.
import './App.css';
import Message from './Components/Message';
function App() {
return (
<div className="App">
<Message />
</div>
);
}
export default App;
Next, write the below code in the index.js file, which is the starting point, where the code gets rendered.
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
After that in the Message.js, we create a message component that will show the message on the page.
Without Destructing code:
import {Component} from "react";
class Message extends Component{
render(){
const message={
message1 : "Hey",
message2:"Welcome to the React JS"
}
return <h1>{message.message1} {message.message2}</h1>
// const{message1,message2}= message
// return <h1>{message1} {message2}</h1>;
}
}
export default Message
The above code gets rendered on the page:
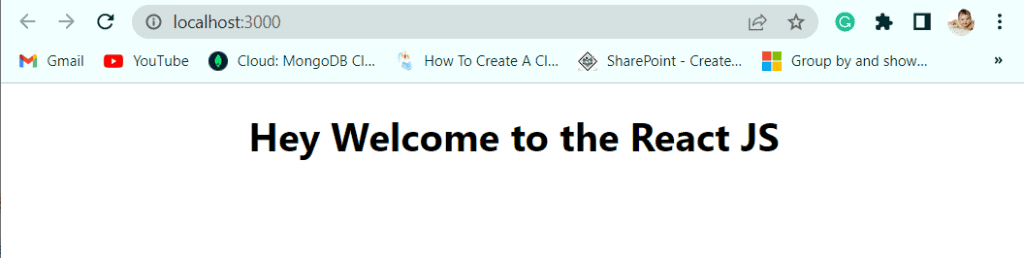
With destructing code
import {Component} from "react";
class Message extends Component{
render(){
const message={
message1 : "Hey",
message2:"Welcome to the React JS"
}
// destructuring code
const{message1,message2}= message
return <h1>{message1} {message2}</h1>;
}
}
export default Message

Example of Destructuring with props
So in this example, we will render the message based on the user, if the user is new then the user can see ” Please Sign up To use react js”, else for the existing users the message is “Welcome back! To react js”
Let’s say the index.html file contains the below statement inside the body tag:
<div id="root"></div>
Next, we will create a Guest greeting component(for new users) with props in GuestGreetings.js.
With destructuring
const message={
message1: "Please Sign up",
message2: "To access React js"
}
// destructing
const {message1,message2}= message;
function GuestGreetings(props) {
return <><h1>{message1} </h1>
<p>{message2}</p>
</>
}
export default GuestGreetings
Next, we will create a UserGreetings component(for new users) with props in UserGreetings.js.
With destructuring:
const message={
message1: "Welcome back",
message2: "To React js"
}
// destructing
const {message1,message2}= message;
function UserGreeting(props) {
return <>
<h2>{message1} </h2><br></br>
<p> {message2}</p>
</>
}
export default UserGreeting
Next, to render the message on the page we need to check the condition whether the use user is new, then isLoggedIn is set to ‘false’ else ‘true’ in index.js.
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import UserGreeting from './Components/UserGreeting'
import GuestGreetings from './Components/GuestGreetings';
function Greeting(props) {
const isLoggedIn = props.isLoggedIn;
if (isLoggedIn) {
return <UserGreeting />;
}
return <GuestGreetings />;
}
const root = ReactDOM.createRoot(document.getElementById('root'));
// Try changing to isLoggedIn={true}:
root.render(<Greeting isLoggedIn={false} />);
Now, the code gets rendered on the page, as you can see the passed value is false, so it will run the GuestGreeting component.
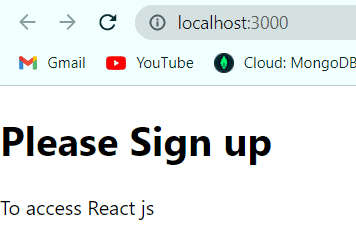
This is how destructuring props in React js works.
Conclusion
In this React js tutorial, we learned what is props in react js and how to use react js with examples. And also we cover the below topics
- What are props in React js
- Why we use props in react js
- Props in React js functional component
- Props in React js Class component
- React default props
- What are render props in react js
- React js props type
- Props.children in react js
- How to pass multiple props in react js
- Conditional props in react js
- React js destructuring props
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com