This spfx tutorial will see how to install and integrate Jquery and JQueryUI accordion into the spfx web part. So here we will cover the below points to add the create spfx web part with JQuery and JQueryUI accordion:
- What is JQuery?
- What is the JQueryUI accordion?
- Create spfx web part with JqueryUI accordion
- Create an spfx web part solution
- How to Install JQuery and JQueryUI NPM packages
- Construct the Accordion HTML in the spfx web part
- Add the Accordion HTML to the spfx web part
- Add jQuery and jQueryUI to the spfx web part
- Render the Accordion
If you are new to spfx, check out, Set up SharePoint Framework (SPFx) development environment
What is JQuery?
JQuery is a JavaScript library that is quick, compact, and packed with features. With an easy-to-use API that works across a wide range of browsers, it simplifies HTML document navigation and manipulation, event handling, animation, and Ajax. jQuery has altered the way millions of people write JavaScript due to its versatility and extensibility.
What is the JQueryUI accordion?
The jQuery UI Accordion is an expandable and collapsible content container with portions that resemble tabs.
We can use the JQueryUI accordion in two ways:
Way 1: The accordion (options) function indicates that an HTML element and its contents should be considered accordion menus and maintained as such. The options parameter is an object that determines how the menu will appear and behave.
Syntax:
$(selector, context).accordion (options);
Way 2: The accordion (“action”, params) method is used to conduct actions on accordion components, such as choosing or deselecting the accordion menu. In the first parameter, the action is provided as a string (e.g., “disable” disables all menus).
$(selector, context).accordion ("action", params);
Create spfx web part with JqueryUI accordion
Here we will see how to create an spfx web part with JQueryUI accordion.
First, we will create an spfx web part and then we will see how to install JQuery and JQueryUI accordion. Also, we will see how to use JQuery UI accordion to create an expandable and collapsible content container with portions that resemble tabs in the web part with SharePoint Framework.
In below screenshot, you can see the example
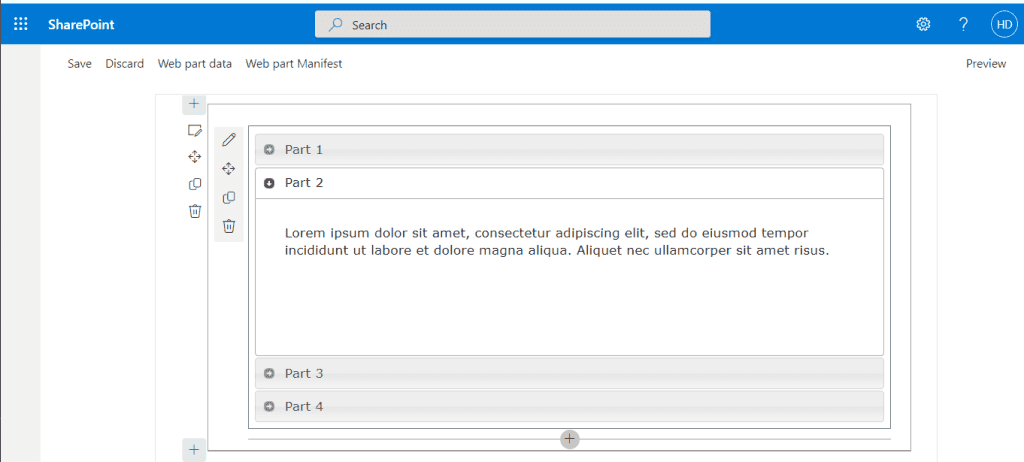
Create an spfx web part solution
First, create a directory and then run the below command to create an spfx solution:
yo @microsoft/sharepoint
A sequence of questions will be presented to you by the Yeoman SharePoint Generator.
- Which type of client-side component to create? WebPart
- What is your Web part name? JQueryWebpart
- Which template would you like to use? No javascript framework
At this stage, Yeoman installs the relevant dependencies and scaffolds the solution files, including the web element. This might take a while.
Read (SPFx) SharePoint framework client web part example
How to Install JQuery and JQueryUI NPM packages
The web part makes use of jQuery and the Accordion component from the jQuery UI project. Add the following to the project’s dependencies to utilize them.
To install the jQuery NPM package, run the following commands in the console:
npm install jquery@2
Next, run the following commands to install the jQueryUI NPM package:
npm install jqueryui
To facilitate the development process, we must install TypeScript type declarations for our project. So, for installing the type declaration packages, run the following statements in the console:
npm install @types/jquery@2 --save-dev
npm install @types/jqueryui --save-dev
As we have installed all the required dependencies to use JQuery and JQueryUI accordion, now we can add the Accordion Html to the spfx web part.
Before that, we need to exclude the dependencies from the web part bundle, which will decrease the file size.
You may set up the project to remove the dependencies from the web part bundle and instead direct the SharePoint Framework runtime to load them as dependencies before loading the web part’s bundle.
Open the configconfig.json file in the code editor. This file includes details about your bundle(s) and their external dependencies. In this file you can see:
- The default bundle information is stored in the bundle’s property. The jQuery web component bundle is in this case. When you add more web parts to your solution, each one has its own entry.
- Libraries that should not be included in the generated bundle are listed in the externals property. Values of this field will also be used by the bundling process to configure the SharePoint Framework’s module loader to load the references libraries before the web component bundle is loaded.
{
"$schema": "https://developer.microsoft.com/json-schemas/spfx-build/config.2.0.schema.json",
"version": "2.0",
"bundles": {
"jquery-webpart-web-part": {
"components": [
{
"entrypoint": "./lib/webparts/jqueryWebpart/JqueryWebpartWebPart.js",
"manifest": "./src/webparts/jqueryWebpart/JqueryWebpartWebPart.manifest.json"
}
]
}
},
"externals": {}
,
"localizedResources": {
"JqueryWebpartWebPartStrings": "lib/webparts/jqueryWebpart/loc/{locale}.js"
}
}
To exclude jQuery and jQueryUI from the resulting bundle by putting the following two modules into the externals property.
When you build your project, jQuery and jQueryUI are no longer included in the default web component bundle.
"externals": {
"jquery": "node_modules/jquery/dist/jquery.min.js",
"jqueryui": "node_modules/jqueryui/jquery-ui.min.js"
}
Now you can refer to the full code:
{
"$schema": "https://developer.microsoft.com/json-schemas/spfx-build/config.2.0.schema.json",
"version": "2.0",
"bundles": {
"jquery-webpart-web-part": {
"components": [
{
"entrypoint": "./lib/webparts/jqueryWebpart/JqueryWebpartWebPart.js",
"manifest": "./src/webparts/jqueryWebpart/JqueryWebpartWebPart.manifest.json"
}
]
}
},
"externals": {
"jquery": "node_modules/jquery/dist/jquery.min.js",
"jqueryui": "node_modules/jqueryui/jquery-ui.min.js"
}
,
"localizedResources": {
"JqueryWebpartWebPartStrings": "lib/webparts/jqueryWebpart/loc/{locale}.js"
}
}
At this stage, the project is set up to rely on jQuery and jQueryUI but not include them in the solution’s bundle. The next step is to add the Accordion to the web component and then implement it.
Read How to Create Site Columns, Content Type and Custom List using SharePoint Framework
Construct the Accordion HTML in the spfx web part
Here we will see how we can create Accordion Html and integrate them in the spfx web part.
Add a new file MyAccordionTemplate.ts to the./src/webparts/jQuery folder in the Code editor
Add the class AccordionTemplate, which includes the accordion’s HTML. To the AccordionTemplate.ts file, add the following code:
export default class AccordionTemplate {
public static templateHtml: string = `
<div class="accordion">
<h3>Part 1</h3>
<div>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor
incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam,
quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
</p>
</div>
<h3>Part 2</h3>
<div>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Aliquet nec ullamcorper sit amet risus.
</p>
</div>
<h3>Part 3</h3>
<div>
<p>
Quis blandit turpis cursus in. Luctus accumsan tortor posuere ac ut consequat semper viverra.
Diam maecenas sed enim ut sem viverra aliquet. Curabitur gravida arcu ac tortor dignissim.
Purus non enim praesent elementum facilisis leo vel fringilla. Vivamus at augue eget arcu dictum.
</p>
<ul>
<li>List item one</li>
<li>List item two</li>
<li>List item three</li>
</ul>
</div>
<h3>Part 4</h3>
<div>
<p>
Quisque id diam vel quam elementum. Nibh ipsum consequat nisl vel pretium
lectus quam id. Quis ipsum suspendisse ultrices gravida dictum.
</p>
<p>
Auctor elit sed vulputate mi sit amet mauris. Sociis natoque penatibus et magnis dis parturient montes nascetur.
Sapien et ligula ullamcorper malesuada proin libero nunc. Et tortor consequat id porta nibh venenatis cras sed.
Ut ornare lectus sit amet est
</p>
</div>
</div>`;
}
Add the Accordion HTML to the spfx web part
Here we will import the Accordion Html to the spfx web part.
Open./src/webparts/jQuery/JqueryWebpartWebPart.ts in the Code editor. Add the following import statement directly after the current import statements at the start of the file:
import AccordionTemplate from '../JQ/AccordianTemplate';
Add jQuery and jQueryUI
Here we will import JQuery and JQueryUI to use in the web part
Import jQuery to your web component, in the same manner, you did AccordionTemplate.
After the current import statements, add the following import statement:
import * as jQuery from 'jquery';
import 'jqueryui';
The jQueryUI project implements its user experience using an external CSS file. This CSS file must be loaded by your web component during runtime:
- To retrieve a CSS file at runtime, utilize the SharePoint module loader and the SPComponentLoader object.
import { SPComponentLoader } from '@microsoft/sp-loader';
Import the jQueryUI styles into the JQueryWebPart web component class by creating a constructor and using the SPComponentLoader.
public constructor() {
super();
SPComponentLoader.loadCss('//code.jquery.com/ui/1.11.4/themes/smoothness/jquery-ui.css');
}
This code accomplishes the following:
- To initialize the web component calls the parent constructor with the context.
- Loads the accordion styles in the CSS file from a CDN asynchronously.
Render the Accordion
Locate the render() function in the jQueryWebPart.ts file. Replace the contents of the render() function with the following to render the accordion HTML in the web part’s inner HTML:
this.domElement.innerHTML = AccordionTemplate.templateHtml;
You may configure the accordion using a few settings in jQueryUI Accordion. After the existing this, define a few choices for your accordion. line of code: this.domElement.innerHTML = AccordionTemplate.templateHtml;
const accordionOptions: JQueryUI.AccordionOptions = {
animate: true,
collapsible: false,
icons: {
header: 'ui-icon-circle-arrow-e',
activeHeader: 'ui-icon-circle-arrow-s'
}
};
You may declare a typed variable called JQueryUI using the jQueryUI typed declaration. AccordionOptions and indicate the characteristics that are supported.
If you experiment with IntelliSense, you’ll discover that it supports all of the accessible methods in JQueryUI, as well as the method arguments.
Now, define the accordion:
jQuery('.accordion', this.domElement).accordion(accordionOptions);
As you can see, you make use of the variable jQuery, which was created when you imported the jquery module. The accordion is then initialized.
This is how the final render() function should look:
public render(): void {
this.domElement.innerHTML = AccordionTemplate.templateHtml;
const accordionOptions: JQueryUI.AccordionOptions = {
animate: true,
collapsible: false,
icons: {
header: 'ui-icon-circle-arrow-e',
activeHeader: 'ui-icon-circle-arrow-s'
}
};
jQuery('.accordion', this.domElement).accordion(accordionOptions);
}
Now we can check the solution by running the below command:
gulp serve
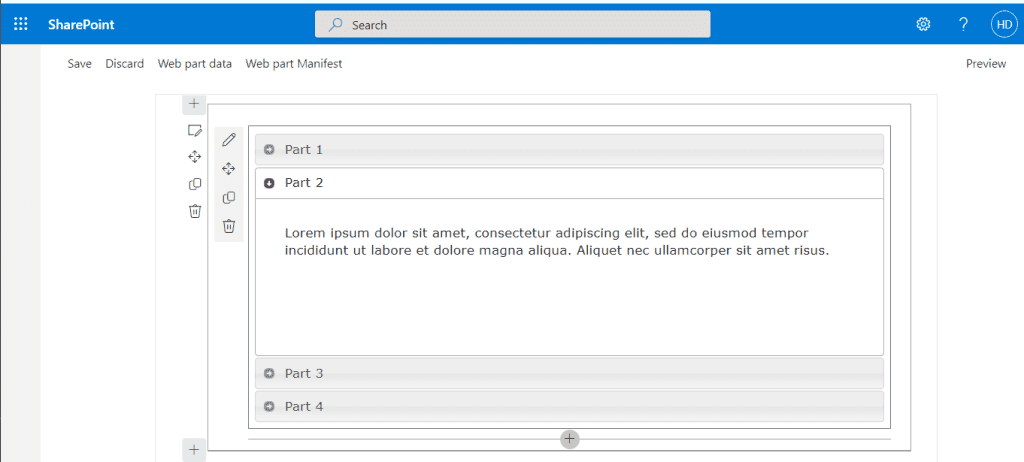
This is how we can create an spfx web part with jquery accordion.
Download Spfx solution
To use this spfx solution, download the solution file, unzip it and run the below command.
npm i
Conclusion
In this spfx tutorial, we saw how we can integrate the JQuery and JQueryUI accordion in the spfx web part. Here are the topics we covered:
- What is JQuery?
- What is the JQueryUI accordion?
- Create spfx web part with JqueryUI accordion
- Create an spfx web part solution
- How to Install JQuery and JQueryUI NPM packages
- Construct the Accordion HTML in the spfx web part
- Add the Accordion HTML to the spfx web part
- Add jQuery and jQueryUI to the spfx web part
- Render the Accordion
You may like the following spfx tutorials:
- SharePoint client-side web part configurable properties in Property Pane using spfx
- How to add custom controls in SPFx property pane
- @microsoft/generator-sharepoint update check failed
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com