Do you have any requirements for getting the file’s last modified date using PowerShell? This tutorial explains with examples, “PowerShell get file last modified date“.
PowerShell to Get the file’s Last Modified Date
The last modified date of a file is a piece of metadata that the operating system maintains, which records the last time the file was changed. This could be when the file was last saved, when its content was altered, or when its properties were changed.
Now, let us see how to get a file’s last modified date using PowerShell.
PowerShell makes it easy to find the last modified date of a file using a few simple commands. The primary attribute we are interested in is LastWriteTime
, which is a property of the file object that PowerShell can access.
The Get-Item
Cmdlet
The Get-Item
cmdlet is used to retrieve the file object from the file system, and from there, you can access its LastWriteTime
property. Here’s a basic example:
$file = Get-Item "C:\MyFolder\MyNewFile.txt"
$file.LastWriteTime
This command will output the last modified date and time of the specified file.
The Get-ChildItem
Cmdlet
If you want to retrieve the last modified dates for all files in a directory, you can use the PowerShell Get-ChildItem
cmdlet:
Get-ChildItem "C:\MyFolder\" | Select-Object Name, LastWriteTime
This command will list all the files in the specified directory along with their last modified dates.
Formatting the Date
If you need the date in a specific format, you can use the ToString()
method:
$file = Get-Item "C:\MyFolder\MyNewFile.txt"
$file.LastWriteTime.ToString("MM/dd/yyyy HH:mm:ss")
This will output the date in the format of month/day/year followed by the time in hours:minutes.
Once you run the code, you can see the output in the below screenshot.
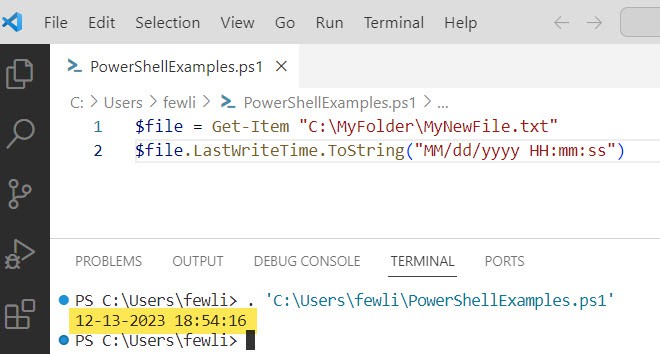
PowerShell Get Files Modified After a Certain Date
You might want to find all files that were modified after a certain date. You can do this by comparing the LastWriteTime
property to a specific date in PowerShell:
Here is a PowerShell script to get all the files modified 30 days ago using PowerShell.
$cutOffDate = (Get-Date).AddDays(-30) # 30 days ago
Get-ChildItem "C:\MyFolder\" | Where-Object {$_.LastWriteTime -gt $cutOffDate} | Select-Object Name, LastWriteTime
This command will list all files in the specified directory that have been modified in the last 30 days using PowerShell.
Here is the output after I ran the PowerShell script using Visual Studio Code.
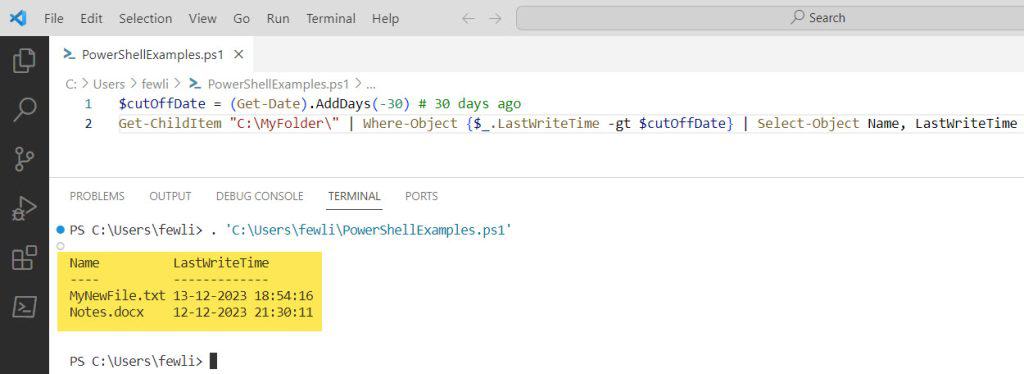
Conclusion
In this PowerShell tutorial, I have explained how to Get the File’s Last Modified Date in PowerShell with examples. Also, I have explained how to get files modified 30 days ago using PowerShell.
You may also like:
- PowerShell Get-Date Minus 1 Day
- PowerShell get-date -uformat
- PowerShell check if file modified in last 24 hours
- PowerShell get file size
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com