In this tutorial, we will go through different methods to check if a file has been modified in the last 24 hours using PowerShell.
To check if a file was modified in the last 24 hours using PowerShell, use the Get-Item cmdlet to retrieve the file’s properties and compare its LastWriteTime property with the current time minus 24 hours. Here’s a concise script snippet:
$file = Get-Item “C:\path\to\your\file.txt”
if ($file.LastWriteTime -gt (Get-Date).AddHours(-24)) { Write-Host “File was modified in the last 24 hours.” }
This script will output a message if the specified file has been modified within the last 24 hours.
Check if a file modified in last 24 hours in PowerShell
Now, let us explore various methods to check if a file has been modified in the last 24 hours in PowerShell.
Method 1: Using Get-Item and LastWriteTime
The simplest way to check if a file was modified in the last 24 hours in PowerShell is by using the Get-Item cmdlet to retrieve the file properties and then comparing the LastWriteTime property with the current time. Here is the complete PowerShell script:
# Define the path to the file
$filePath = "C:\MyFolder\Resume.pdf"
# Get the file item
$file = Get-Item $filePath
# Check if the file was modified in the last 24 hours
if ($file.LastWriteTime -gt (Get-Date).AddHours(-24)) {
Write-Host "The file '$filePath' has been modified in the last 24 hours."
} else {
Write-Host "The file '$filePath' has not been modified in the last 24 hours."
}
Once you run the PowerShell script using Visual Studio Code, you can see the output in the below screenshot.
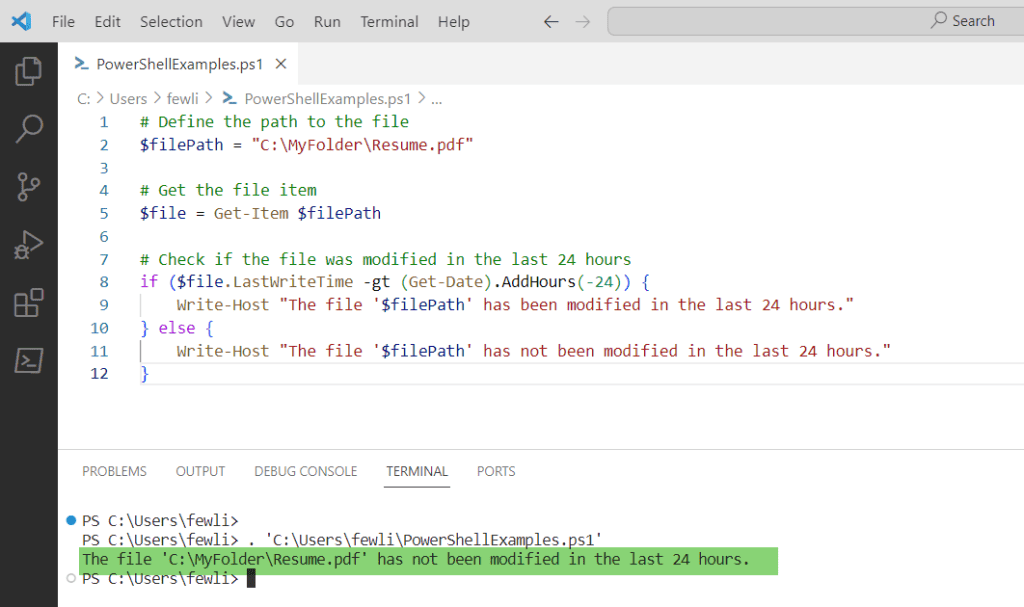
Method 2: Using Get-ChildItem for Multiple Files
If you need to check multiple files in a directory if they are files modified in the last 24 hours, you can use the Get-ChildItem cmdlet to retrieve all files and then filter them based on the LastWriteTime property in PowerShell. Here is the complete code:
# Define the path to the file
$directoryPath = "C:\MyFolder"
# Get all files in the directory
$files = Get-ChildItem $directoryPath
# Check each file to see if it was modified in the last 24 hours
foreach ($file in $files) {
if ($file.LastWriteTime -gt (Get-Date).AddHours(-24)) {
Write-Host "The file '$($file.FullName)' has been modified in the last 24 hours."
}
}
Here you can see the screenshot below after I ran the code using VS Code.
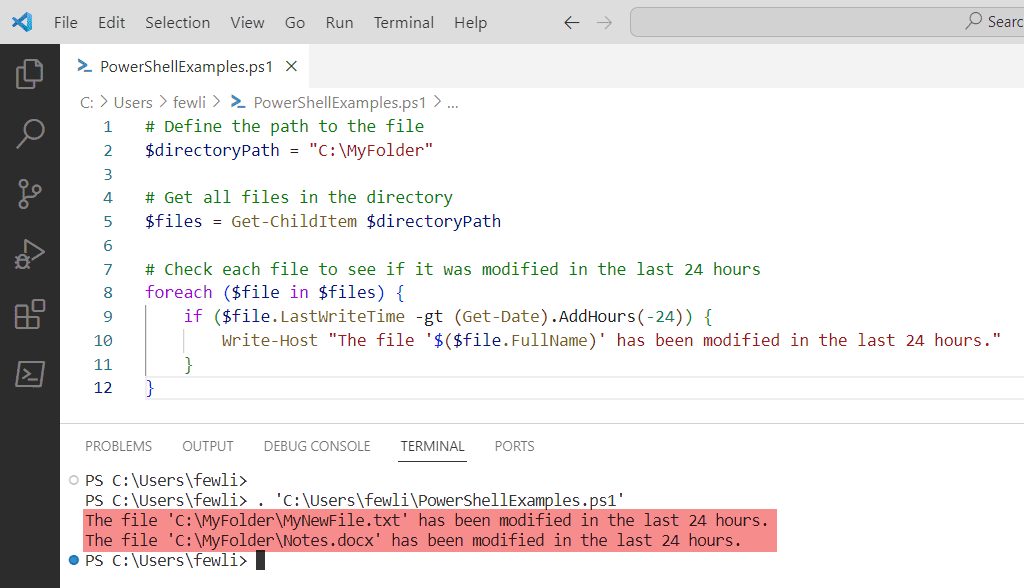
Method 3: Using Test-Path and LastWriteTime
If you need to first check if the file exists before checking its modification time, you can combine Test-Path with the LastWriteTime property check. Here is the complete PowerShell script.
# Define the path to the file
$filePath = "C:\MyFolder\Resume.pdf"
# Check if the file exists
if (Test-Path $filePath) {
# Get the file item
$file = Get-Item $filePath
# Check if the file was modified in the last 24 hours
if ($file.LastWriteTime -gt (Get-Date).AddHours(-24)) {
Write-Host "The file '$filePath' has been modified in the last 24 hours."
} else {
Write-Host "The file '$filePath' has not been modified in the last 24 hours."
}
} else {
Write-Host "The file '$filePath' does not exist."
}
PowerShell find files created in last 24 hours
Let us see the PowerShell commands to identify and list files within a specific directory that have been created within the past 24-hour period.
To accomplish this task, PowerShell provides cmdlets such as Get-ChildItem, which can be used to retrieve a list of files and directories in a specified path, and properties like CreationTime, which indicates when a file or directory was created.
Here’s a basic example of how to use PowerShell to find files that were created in the last 24 hours:
# Define the path to the directory you want to check
$directoryPath = "C:\MyFolder"
# Get the current date and time
$currentDate = Get-Date
# Find and list files in the directory that were created in the last 24 hours
Get-ChildItem -Path $directoryPath | Where-Object { $_.CreationTime -gt $currentDate.AddHours(-24) }
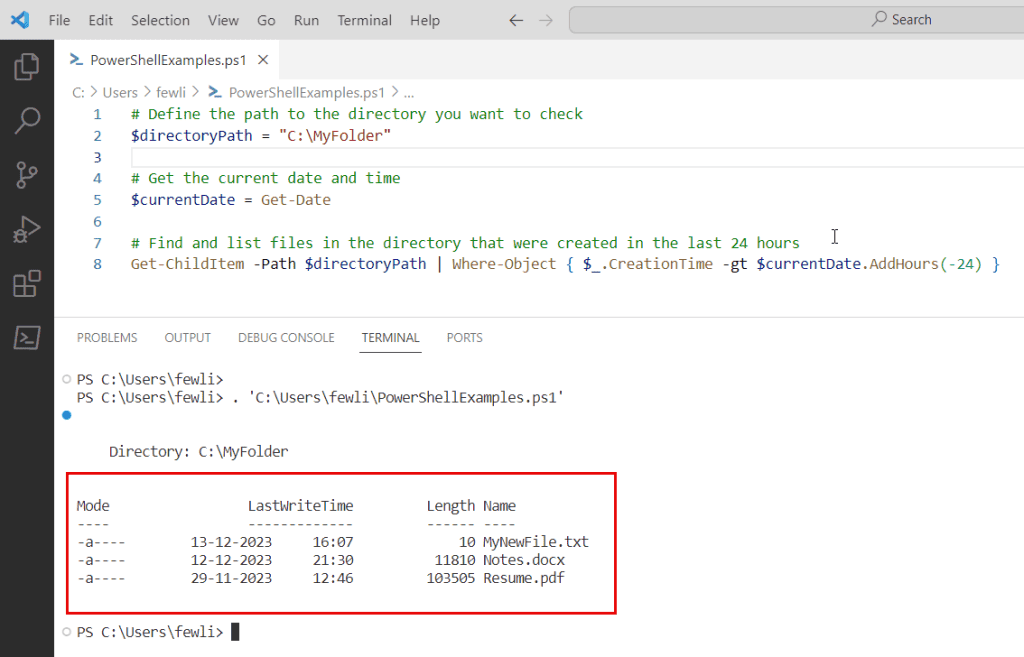
This PowerShell script retrieves a list of all files in the specified directory and filters them using the Where-Object cmdlet to only include those whose CreationTime is greater than (more recent than) the current date and time minus 24 hours. The result is a list of files that have been created within the last 24 hours in that directory.
PowerShell find files modified in last hour
Let us see, how to use PowerShell to search for and identify files in a specific directory that have been modified within the previous hour. PowerShell offers the Get-ChildItem cmdlet to list files and directories, and it allows you to access file attributes, including LastWriteTime, which indicates the last time a file was written to or modified.
Here is an example of how you can use PowerShell to find files that were modified in the last hour:
# Define the path to the directory you want to search
$directoryPath = "C:\MyFolder"
# Calculate the time one hour ago from the current time
$oneHourAgo = (Get-Date).AddHours(-1)
# Retrieve and list files modified in the last hour
Get-ChildItem -Path $directoryPath -File | Where-Object { $_.LastWriteTime -gt $oneHourAgo }
This script lists all the files in the specified directory that have a LastWriteTime greater than the time calculated for one hour ago. The -File switch is used with Get-ChildItem to ensure that only files (not directories) are considered. The resulting output will include all files modified within the last hour from the time the script is run.
For the output, check the below screenshot.
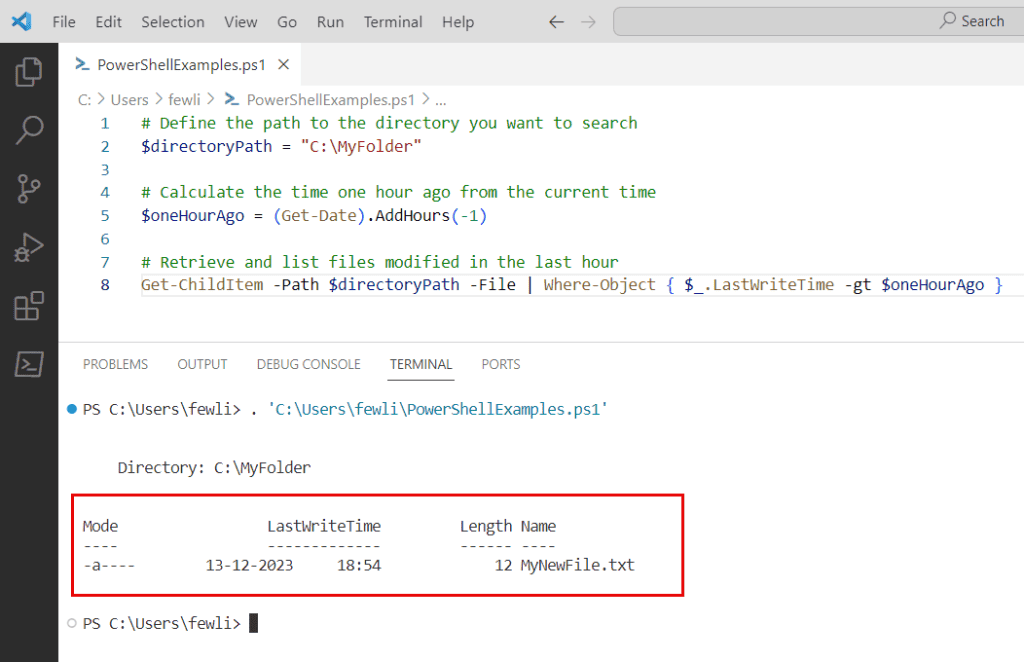
Conclusion
In this PowerShell tutorial, you have learned different methods to check if a file has been modified in the last 24 hours using PowerShell. I have also explained how to find files created in the last 24 hours in PowerShell and also how to find files modified in the last hour in PowerShell.
You may also like:
- PowerShell create folder if not exists
- PowerShell create file if not exists
- PowerShell get file size
- PowerShell Create Log File
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com
I would like to see the same thing except in SharePoint Online.
Thanks for these valuable information. I hope next time, you’ll post new commands that would tell us WHO on the network modified the files. I wonder if this is possible even when Audit is not turned on.