Recently, while working on a PowerShell automation task, I got a requirement to split an array into smaller arrays in PowerShell. In this PowerShell tutorial, I will explain how to split an array into smaller arrays in PowerShell.
To split an array into smaller arrays in PowerShell, you can use a loop to iterate over the elements and collect them into sub-arrays. Here’s a concise example using a for
loop:
$originalArray = 1..20
$size = 5
$smallerArrays = for ($i = 0; $i -lt $originalArray.Count; $i += $size) { $originalArray[$i..($i+$size-1)] }
This snippet creates an array $originalArray
, defines a chunk size $size
, and then uses a for
loop to slice the original array into smaller arrays of the specified size, which are stored in $smallerArrays
.
Split an Array into Smaller Arrays in PowerShell
Before diving into splitting arrays, it’s important to understand what an array is. In PowerShell, an array is a data structure that stores a collection of items. These items can be of any data type, and you can access them by referring to their index number, which starts at 0.
For example, here’s how you can create an array in PowerShell:
$myArray = @(1, 2, 3, 4, 5)
Method 1: Using Loops to Split an Array
The most straightforward way to split an array into smaller arrays is by using loops in PowerShell. You can use a for
loop to iterate over the elements and group them into new arrays.
Here’s a simple example:
function Split-Array {
param(
[array]$ArrayToSplit,
[int]$Size
)
$result = @()
$group = @()
for ($i = 0; $i -lt $ArrayToSplit.Length; $i++) {
$group += $ArrayToSplit[$i]
if (($i + 1) % $Size -eq 0 -or $i -eq $ArrayToSplit.Length - 1) {
$result += ,@($group)
$group = @()
}
}
return $result
}
# Example usage:
$originalArray = @(1..20)
$splitArrays = Split-Array -ArrayToSplit $originalArray -Size 5
$splitArrays
This function Split-Array
takes an array and the desired size of the smaller arrays as inputs. It then groups elements into new arrays and adds these to the result array.
You can see the output in the screenshot below after I ran the code using VS code:
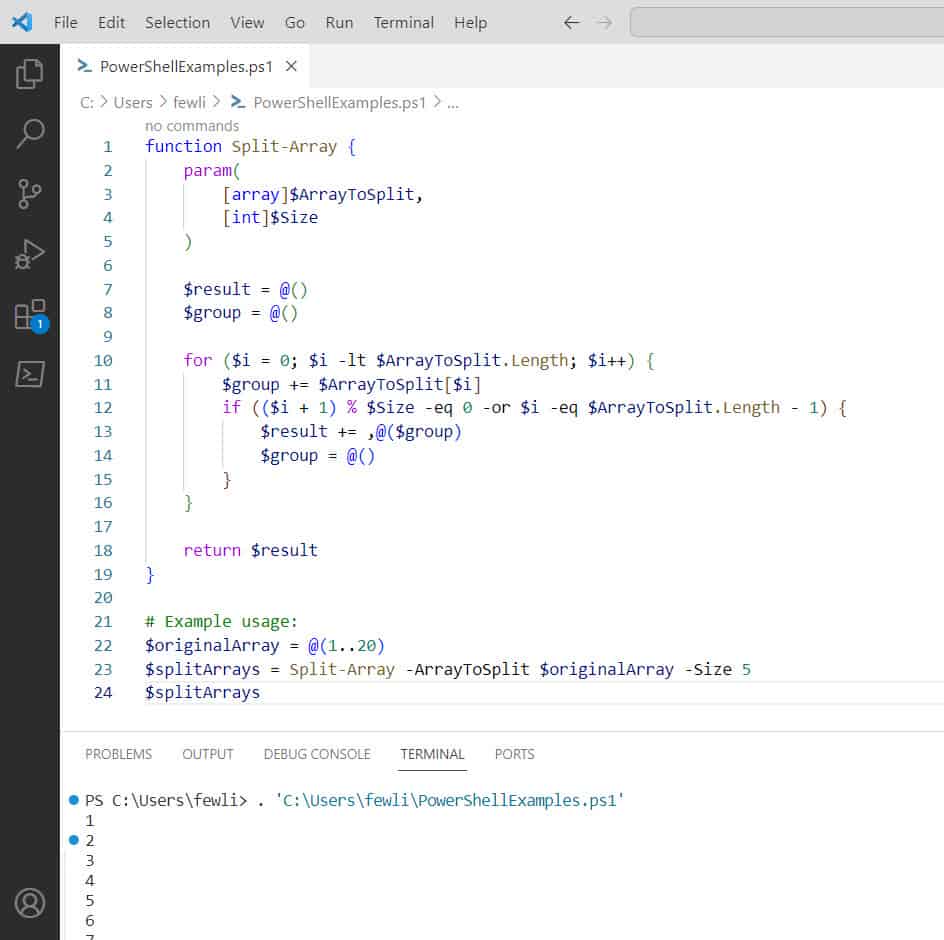
Method 2: Using the Range Operator and Array Slicing
Another method to split an array is by using the range operator and array slicing in PowerShell. This is a more concise way to achieve the same result without explicitly using loops.
Here’s an example:
function Split-Array {
param(
[array]$ArrayToSplit,
[int]$Size
)
$result = @()
for ($i = 0; $i -lt $ArrayToSplit.Length; $i += $Size) {
$result += ,@($ArrayToSplit[$i..($i + $Size - 1)])
}
return $result
}
# Example usage:
$originalArray = 1..20
$splitArrays = Split-Array -ArrayToSplit $originalArray -Size 5
$splitArrays
In this function, the range operator ..
is used to create a sub-array of the desired size, which is then added to the result array.
Method 3: Using Custom PowerShell Functions
Sometimes, you might find that you need a more flexible or powerful solution. In that case, you can write a custom PowerShell function to split your array.
Here is a complete PowerShell script for splitting an array into smaller arrays using a custom function. This example assumes a function that takes two parameters: the array to be split and the size of the smaller arrays. It then processes the array and returns an array of arrays.
function Split-Array {
param(
[Parameter(Mandatory=$true)][array]$ArrayToSplit,
[Parameter(Mandatory=$true)][int]$Size
)
$result = @()
$index = 0
while ($index -lt $ArrayToSplit.Length) {
$end = $index + $Size
if ($end -gt $ArrayToSplit.Length) {
$end = $ArrayToSplit.Length
}
$result += ,@($ArrayToSplit[$index..($end - 1)])
$index += $Size
}
return $result
}
# Example usage:
$originalArray = 1..20
$splitArrays = Split-Array -ArrayToSplit $originalArray -Size 5
# Output the result
$splitArrays | ForEach-Object { Write-Output $_ }
In this script, the Split-Array
function uses a while
loop to iterate over the original array in chunks defined by the $Size
parameter. It slices the array within the loop and adds each chunk as a sub-array to the result. The example usage demonstrates how to call this function with an array of numbers from 1 to 20, splitting it into smaller arrays of size 5. The last line outputs each of the smaller arrays to the console.
Method 4: Using .NET Methods
PowerShell is built on top of .NET, which means you can leverage .NET classes and methods to perform various tasks, including splitting an array.
For example, you can use the System.Array
class to create, manipulate, and manage arrays. Though there isn’t a direct method to split an array in the .NET framework, you can create a function that utilizes .NET methods to achieve this:
function Split-Array {
param(
[array]$ArrayToSplit,
[int]$Size
)
$result = New-Object System.Collections.ArrayList
for ($i = 0; $i -lt $ArrayToSplit.Length; $i += $Size) {
$chunk = [System.Array]::CreateInstance($ArrayToSplit.GetType().GetElementType(), $Size)
[System.Array]::Copy($ArrayToSplit, $i, $chunk, 0, $Size)
$result.Add($chunk)
}
return $result.ToArray()
}
# Example usage:
$originalArray = [int[]](1..20)
$splitArrays = Split-Array -ArrayToSplit $originalArray -Size 5
$splitArrays
In this example, the System.Array::CreateInstance
method is used to create a new array of the specified size, and the System.Array::Copy
method is used to copy a portion of the original array into the new array.
Conclusion
Splitting an array into smaller arrays in PowerShell can be achieved through several methods, each with its own advantages. PowerShell allows you to manipulate arrays to suit your needs, whether you choose to use loops, range operators, custom functions, or .NET methods.
In this PowerShell tutorial, I have explained how to split an array into smaller arrays in PowerShell using the below methods:
- Using Loops to Split an Array
- Using the Range Operator and Array Slicing
- Using Custom PowerShell Functions
- Using .NET Methods
You may also like:
- How to Replace a String in an Array Using PowerShell?
- How to Loop Through an Array of Objects in PowerShell?
- How to Convert Multiline String to Array in PowerShell?
- How To Sort Array Of Objects In PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com