A reset form in a web application plays an important role to reset the form so that the user can insert the new input to the form. In this tutorial, we will discuss how to reset form with controlled inputs and uncontrolled inputs, and a reset form with different libraries.
So, this tutorial illustrates the below content on how users can create a reset form:
- Reset form in react js
- Reset the Uncontrolled inputs in the Form in React js
- Reset the Controlled inputs in the Form in React js
- Reset form in react js functional component
- reset form after submit in react js
- how to reset formik form in react js
- how to reset form on button click in react js
- react ref reset form
Read React js form examples for beginners
Reset form in react js
Here we will see how to reset the form in react js in controlled component and uncontrolled component.
If you employ uncontrolled form inputs, a reset input type will return the form inputs to their original values. When using controlled inputs, each input state variable value must be set to its default value.
Reset the Uncontrolled inputs in the Form in React js
Now we will see how to reset the uncontrolled values in the React js.
For example, we will create a form, that contains 4 fields i.e. Name, Address, contact number, and Vaccination status with the reset button. By clicking on the Reset button the form will get reset.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
import React, {Component} from "react";
class App extends Component {
render() {
return (
<div className='App'>
<Form />
</div>
);
}
}
export default App;
In the Form component write the below code:
import React, {useState} from "react";
const Form = () => {
const [status, setStatus] = useState(" ");
return(
<form>
<h2> Vaccination Form</h2>
<div><span> Full Name:</span><input/></div>
<br/>
<div><span> Address:</span><input/></div>
<br/>
<div><span>Contact Number:</span><input/></div>
<br></br>
<div><span>Vacination Status:</span>
<select value={status} onChange={(e) => setStatus(e.target.value)}>
<option value="">Select</option>
<option value="Dose 1">Dose 1</option>
<option value="Dose 2">Dose 2</option>
</select>
</div>
<br/>
<div><input type="reset" value="Reset Form"/></div>
</form>
)
}
export default Form;
Now run the application, with the npm start command, and you can see the form render on the page. Then fill out the form, and to reset the form click on the reset button.
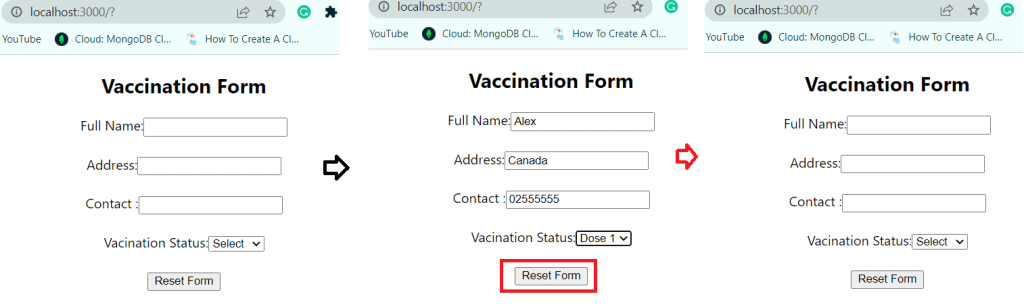
Reset the Controlled inputs in the Form in React js
Here we will see how to reset the controlled inputs in the Form in react js.
When using controlled inputs, it is critical to reset the state variables that retain input values.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
import React, {Component} from "react";
class App extends Component {
render() {
return (
<div className='App'>
<Form />
</div>
);
}
}
export default App;
In the Form component write the below code:
import { useState } from "react"
export const Form = () => {
const [name, setName] = useState()
const [password, setpassword] = useState()
const [category, setCategory] = useState()
const resetForm = () => {
setName("")
setpassword("")
setCategory("")
}
return(
<>
<form>
<div><span>Name:</span><input value={name} onChange={(e) => setName(e.target.value)}/></div>
<br/>
<div><span>Password:</span><input type= "password" value={password} onChange={(e) => setpassword(e.target.value)}/></div>
<br/>
<div><span>Category:</span>
<select value={category} onChange={(e) => setCategory(e.target.value)}>
<option value="">Select</option>
<option value="Seller">Seller</option>
<option value="Buyer">Buyer</option>
</select>
</div>
<br/>
<div>
<input type="button" value="Reset Form" onClick={() => resetForm()}/>
</div>
</form>
<br/>
{name && password && category &&
<div> <p>{name}</p>
<p>{category}</p>
</div>
}
</>
)
}
export default Form;
Now run the application with the npm start command, and the form will render on the page
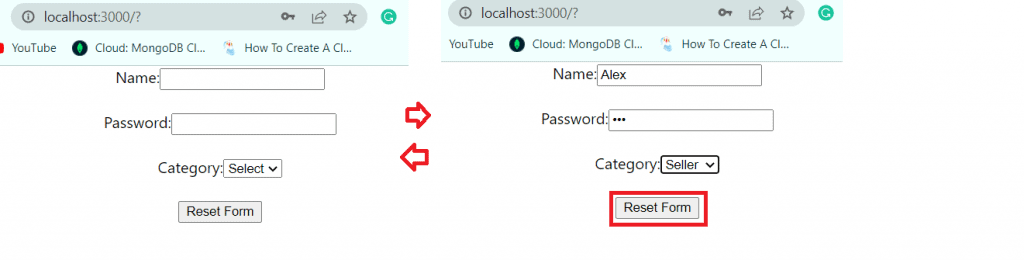
Read Conditional rendering react js
Reset form in react js functional component
Here we will see how to reset the form in the functional component in React js.
We will create a simple react js form with one text input field. You can clear the value of an input with a ref inside a functional component by setting the ref.current.value to an empty string.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
import React, {Component} from "react";
class App extends Component {
render() {
return (
<div className='App'>
<Form />
</div>
);
}
}
export default App;
In the Form component write the below code:
import React from "react"
const Form = () => {
const textInput = React.useRef();
const resetInput = () => (textInput.current.value = "");
return (
<form>
<h2> Fill the Form👇</h2>
<label> Name:
<input type="text" ref={textInput} />
<button onClick={resetInput}>Reset</button>
</label>
</form>
);
}
export default Form;
Now run the application with the npm start command and you can see the form with one field rendered on the page. Then fill out the Form and click on the Reset button to reset the form.
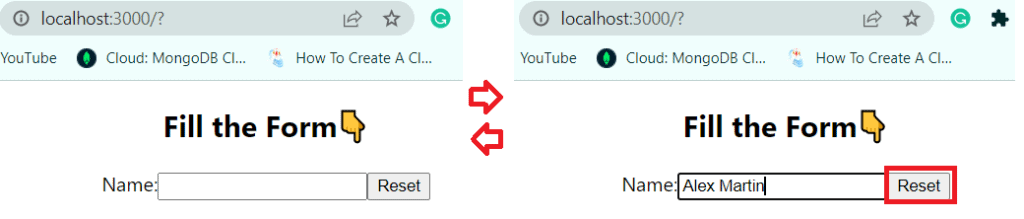
Read Handling Events in React js
Reset form after submit in react js
Here we will see how to reset the form after submitting in react js.
To clear/reset the input values after submitting a form in React:
The values of the input fields are saved in state variables.
Set the form element’s onSubmit property.
Set the state variables to empty strings when the submit button is pressed.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
import React, {Component} from "react";
class App extends Component {
render() {
return (
<div className='App'>
<Form />
</div>
);
}
}
export default App;
In the Form component write the below code:
import { useState } from 'react';
const Form = () => {
const [fullName, setFullName] = useState('');
const [department, setDepartment] = useState('');
const handleSubmitForm = event => {
alert('form submitted');
event.preventDefault(); // 👈️ prevent page refresh
// 👇️ clear all input values in the form
setFullName('');
setDepartment('');
};
return (
<div>
<form onSubmit={handleSubmitForm}>
<h2> Fill the form 📄</h2>
<label> Full name:
<input
id="full_name"
name="full_name"
type="text"
onChange={event => setFullName(event.target.value)}
value={fullName}
/>
</label>
<br></br>
<label> Department:
<input
id="department"
name="department"
type="text"
value={department}
onChange={event => setDepartment(event.target.value)}
/>
</label>
<br></br>
<button type="submit">Submit form</button>
</form>
</div>
);
};
export default Form;
Now run the application with the npm start command and you can see the form render on the page. Fill out the form and click on submit button, you can see the message ‘Form submitted’ pop in the window, and also the field gets reset.

Read State in React js Hooks
How to reset form on button click in react js
Here we will see how to reset the form on the button click in React js.
So to reset the form value on the button click by using the react-hook-form library in React js.
What is the react-hook-form library?
React Hook Form is a library that allows you to validate forms in React. It is a minimal library with no extra dependencies that is both performant and simple to use, requiring developers to write fewer lines of code than other form libraries.
React hook form includes some useful APIs that will make your life easier. We will show how to use the register, handleSubmit, and reset APIs to dynamically manage form data.
- register(): This method allows you to register an input or select element and build the groundwork for React Hook Form’s validation criteria. All validation rules are HTML-based and permit custom validation.
- handleSubmit() : If form validation is successful, this method returns the form data.
- reset(): This approach is useful for resetting the full form state or a specific section of the form.
The hook form module keeps form complications at bay and makes dealing with forms simple and enjoyable.
In this example, we will see how to set form input values in a React project using React hooks (useEffect and useState) and the react hook form module.
With the below command we can install the React-hook-form in our project.
npm install react-hook-form
Once we install it, now we will see how to create a form with a reset button using react hook form library.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
import React from 'react'
function App() {
return (
<div className='App'>
<Form />
</div>
)
}
export default App;
Now the Form component contains the below code:
import React, { useState, useEffect } from 'react'
import { useForm } from 'react-hook-form'
export default function Form() {
const { register, handleSubmit, reset } = useForm()
const [emp, initemp] = useState(null)
useEffect(() => {
setTimeout(
() =>
initemp({
name: 'John Holland',
email: 'abc@email.com',
mobile: '08923456790',
}),
1000,
)
}, [])
useEffect(() => {
reset(emp)
}, [emp])
function onFormSubmit(dataRes) {
console.log(dataRes);
alert("Form submitted successfully")
return false
}
return (
<div >
<h2>Reset Form Values using React Hook Form</h2>
{emp && (
<form onSubmit={handleSubmit(onFormSubmit)}>
<div >
<label>Name</label>
<input
type="text"
name="name"
{...register('name')}
/>
</div>
<div >
<label>Email</label>
<input
type="email"
name="email"
{...register('email')}
/>
</div>
<div >
<label>mobile</label>
<input
type="text"
name="mobile"
{...register('mobile')}
/>
</div>
<div >
<button type="submit" >
Submit
</button>
<button
type="button"
onClick={() =>
reset({
name: '',
email: '',
mobile: '',
})
}
>
Reset
</button>
</div>
</form>
)}
{!emp && (
<div>
<span></span>
</div>
)}
</div>
)
}
Now run the application with the npm start command and you can see the form render on the page. The field contains the default value, by clicking on the reset button, you can clear the field.
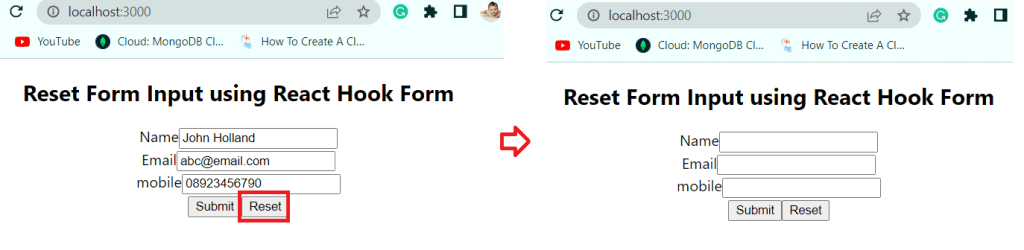
Now fill out the form, and click on submit you can see the values are logged in the console and the form submitted success message will pop on the window. Then you can reset the form to enter the new data.
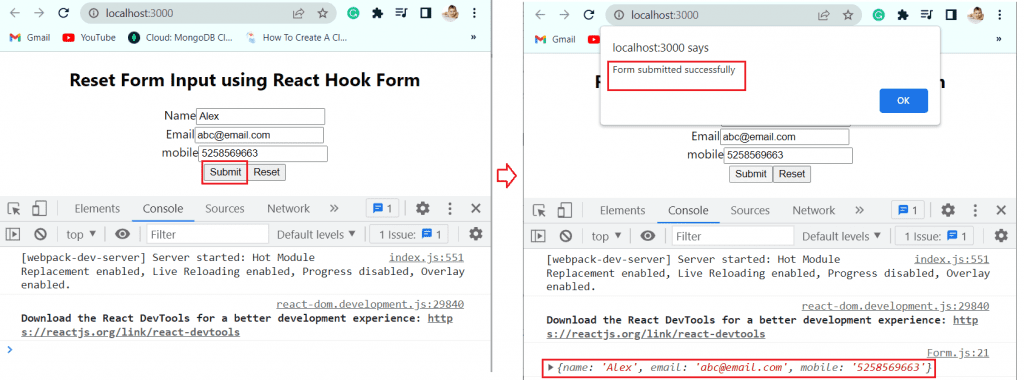
This is how we can reset a form on button click in react js.
Read State in React js
How to reset formik form in react js
Here we will see how to reset the formik form in react js.
Now we will discuss how to reset the form with Formik in react js. Before that, we need to know what is the use of Formik in React js.
Formik comes with all of the capabilities needed to manage forms, such as
- Form state initialization.
When the value of a control changes, the value state is updated. - On form control change, updating errors / touched state
- Handler functions such as handleBlur, handleChange, which alters the form state, and handleSubmit, which invokes the onSubmit handler when the form is submitted.
- The mechanism for form validation
- Form states such as valid, dirty, and submission count.
To use Formik we need to install it in your application, using the below command:
npm install --save formik
We can manage forms with formik by utilizing the Formik /> HOC or the useFormik() hook. The useFormik() hook will be used in this example.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
import React from 'react'
function App() {
return (
<div className='App'>
<Form />
</div>
)
}
export default App;
Now the Form component contains the below code:
import React from 'react';
import { useFormik } from 'formik';
export default function Form() {
const formik = useFormik({
initialValues: {
fullName: '',
email: '',
contactNumber: undefined,
gender: '',
address: ''
},
onSubmit: values => {
alert(
'Form Submitted \n ' + JSON.stringify(values, null, 2)
);
formik.resetForm();
}
});
return (
<div >
<div >
<h1> Survey </h1>
<form onSubmit={formik.handleSubmit}>
<div >
<label> Full Name </label>
<input type="text" {...formik.getFieldProps('fullName')} />
</div>
<div >
<label> Email Id </label>
<input type="text" {...formik.getFieldProps('email')} />
</div>
<div >
<label> Contact Number </label>
<input type="text" {...formik.getFieldProps('contactNumber')} />
</div>
<div >
<label> Gender </label>
<select {...formik.getFieldProps('gender')}>
<option value="">Select</option>
<option value="male">Male</option>
<option value="female">Female</option>
<option value="Other">Other</option>
</select>
</div>
<div >
<label> Address </label>
<textarea type="text" {...formik.getFieldProps('address')} />
</div>
<div>
<button type="submit">
Submit
</button>
<button
type="reset"
onClick={formik.resetForm}
>
Reset
</button>
</div>
</form>
</div>
<div>
<h4>Form State</h4>
<h5>Values:</h5>
<code>
<pre>{JSON.stringify(formik.values, null, 2)}</pre>
</code>
</div>
</div>
);
}
Now run the application with the npm start command and you can see the form render on the page. Fill out the form and click on submit, you can see the information pop on the window.
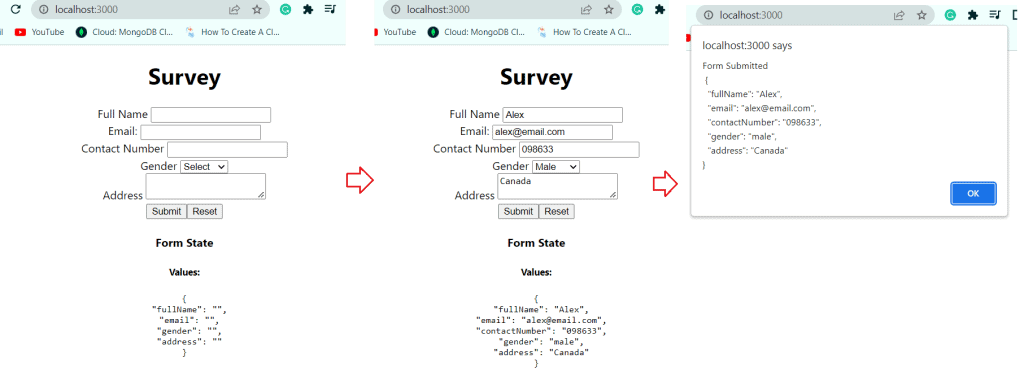
Now fill out the form and click on the reset button, you click on the reset button to reset the form.
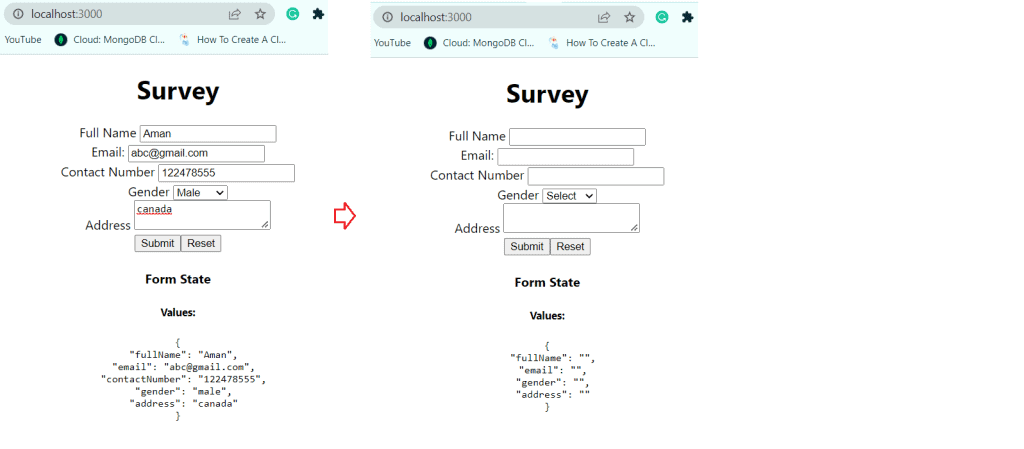
Read Props in React js
React ref reset form
Here we will see how to reset the form with the useRef hook in React js.
If you deal with uncontrolled input fields and track their values with the useRef hook, you can clear the form’s input data with the reset() method.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
import React from 'react'
function App() {
return (
<div className='App'>
<Form />
</div>
)
}
export default App;
Now the Form component contains the below code:
import {useRef} from 'react';
const App = () => {
const fullnameRef = useRef(null);
const departmentRef = useRef(null);
const handleSubmit = event => {
console.log('form submitted');
event.preventDefault();
alert("Form submitted successfully");
// 👇️ clear all input values in the form
event.target.reset();
};
return (
<div>
<form onSubmit={handleSubmit}>
<h2>Fill the form</h2>
<label> Full name:
<input
ref={fullnameRef}
id="full_name"
name="full_name"
type="text"
/>
</label>
<br></br>
<label> Department:
<input
ref={departmentRef}
id="department"
name="department"
type="text"
/>
</label>
<br></br>
<button type="submit">Submit</button>
</form>
</div>
);
};
export default App;
Now run the application with the npm start command, and you can see the form render on the page.

As this react js tutorial provides you with different ways to reset a form in react js. The content we discussed in this tutorial:
- Reset form in react js
- Reset the Uncontrolled inputs in the Form in React js
- Reset the Controlled inputs in the Form in React js
- Reset form in react js functional component
- reset form after submit in react js
- how to reset formik form in react js
- how to reset form on button click in react js
- react ref reset form
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com