As in react js application the cancel button plays a major role when we want to create a form and we want to cancel the values in the form, without saving any changes made by the user. So this tutorial will go through the below content:
- React js cancel button
- cancel and save button in react js
- React js how to build an edit cancel text input
- oncancel react
- Formik cancel button
React js cancel button
In React js, the cancel button cancels editing but leaves the state with the altered value after editing has begun.
So here we will see a simple example of how to handle the cancel button in react js. In this example, we will create a function called ‘handleCancel’ which will manage the cancel when the user clicks on the cancel button.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
function App() {
return (
<div className="App">
<Form></Form>
</div>
);
}
export default App;
The Form.js contains the below code:
import React, {useState} from "react";
export default function Form (){
const [ inputValue, setInputValue ] = useState("Value from onchanges");
const handleCancel = () => {
setInputValue("");
};
return(
<div>
<p> Click on cancel to clear the field </p>
<input type="text" value= {inputValue} onChange={(e) => setInputValue(e.target.value)}></input>
<button type="submit" onClick={handleCancel}>Cancel❌</button>
</div>
)
}
Now run the application with the npm start command and you can see the input field with some text, so when you click on the cancel button, the text gets cleared or canceled.
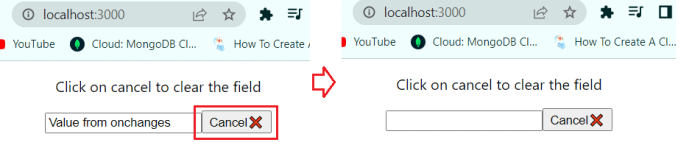
Read How to upload file in react js?
Cancel and save button in react js
Here we will see how to handle the cancel and save buttons in react js.
For example, we will create a component, that will have one input field, a cancel button, and a save button. So here if we click on the cancel button the field gets cleared and when we click on the save button we can see the inputs in the console.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
function App() {
return (
<div className="App">
<Form></Form>
</div>
);
}
export default App;
The Form.js contains the below code:
import React, {useState} from "react";
export default function Form (){
const [ inputValue, setInputValue ] = useState("Value from onchanges");
const handleCancel = () => {
setInputValue("");
};
const handleSave=(event) =>{
console.log(inputValue, event);
}
return(
<div>
<p> Click on cancel to clear the field, or click on save to save the data </p>
<label> Name:
<input type="text" value= {inputValue} onChange={(e) => setInputValue(e.target.value)}></input>
</label>
<button type="submit" onClick={handleCancel}>Cancel❌</button>
<button type="submit" onClick={handleSave}>Save✔</button>
</div>
)
}
Now run the application, with the npm start command, and you can see the input field and you can see the buttons. So when you click on the cancel button, you can see the field get clear, when you click on the save, the input value you can see in the console.

Read How to reset form in react js
React js how to build an edit cancel text input
Here we will see how to build an edit cancel text input in React js.
For example, we will create an application, to edit the text we need to double click on it, once you are in edit mode, you can type, with that we have two buttons, one is the canceled button another is the ok button. If the user will click on the ok button the changes will reflect on the page, and if the user will click on the cancel button it will render the previous text.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
function App() {
return (
<div className="App">
<Form></Form>
</div>
);
}
export default App;
The Form.js contains the below code:
import React, { Component } from "react";
class Form extends Component {
state = {
value: "Double click on me😊",
isInEditMode: false
}
changeEditMode = () => {
this.setState({
isInEditMode: !this.state.isInEditMode
})
}
updateValue = () => {
this.setState({
isInEditMode: false,
value: this.refs.theTextInput.value
})
}
editViewRender = () => {
return <div>
<input type="text" defaultValue="Some text here" ref="theTextInput" />
<button onClick={this.changeEditMode}>Cancel❌</button>
<button onClick={this.updateValue}>🆗</button>
</div>
}
defaultViewRender = () => {
return <div onDoubleClick={this.changeEditMode}>
{this.state.value}
</div>
}
render() {
return <div><h2>Double click on the below text to edit</h2>
{
this.state.isInEditMode ? this.editViewRender() : this.defaultViewRender()
}
</div>
}
}
export default Form;
Now run the application, with the npm start command, and you can see some text render on the page. So, double click on the ‘ Double click on me😊’ and you can see the text box and buttons. Then type something in the text box, and click on the ok button.

Now, double click on the ‘hey are you there?’, and you can see the text box, type something, and then click on the cancel button, you can see the file’s previous text appear on the page.

Read React js form examples for beginners
oncancel react
Here we will see how to define and use the onCancel function in react.
For example, we will create a form, and to handle canceling a form we will use the onCancel function.
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
function App() {
return (
<div className="App">
<Form></Form>
</div>
);
}
export default App;
The Form.js contains the below code:
import React, {useState} from "react";
export default function Form (){
const [ firstName, setFirstName ] = useState("");
const[lastName, setLastName]= useState("")
const handleCancel = () => {
setFirstName("");
setLastName("");
};
return <form onCancel={handleCancel} >
<div>
<p> Click on cancel to clear the field </p>
<label>First Name:
<input type="text" value= {firstName} onChange={(e) => setFirstName(e.target.value)}></input>
</label>
<label> Last name:
<input type="text" value={lastName} onChange={(e)=> setLastName(e.target.value)}></input>
</label>
<button type="submit" onCancel={handleCancel}>Cancel❌</button>
</div>
</form>
}
Now run the application with the npm start command, and you can see the form render on the page. By clicking on the cancel button, you can see the Form values are canceled.

Read Conditional rendering react js
Formik cancel button
Here we will see how to create formik form with cancel button in React js
For example, we will create a form with Formik and Yup to validate the input field in the form. So the form contains the two Field, Full name and contact number, and also two buttons, Send and Cancel. If we click on send button the values get log on the console, and when we click on the Cancel form get cleared.
What is Formik in React js?
Formik is a third-party React form library. It offers simple form programming and validation. It is built on controlled components and drastically cuts down on form programming time.
To install Formik library in the application by using the below command:
npm i formik
What is yup in React js?
Yup is a tool for validating and parsing values in JavaScript. Create a schema, modify a value to match, check the shape of a value, or do both. Yes, schema is quite expressive and enables modeling of intricate, interconnected validations or value transformations.
To install yup in the project by using the below command:
npm i yup
Let’s say the index.html contains the below code:
<div id="root"></div>
The Index.js file is the starting point of the React app, so it contains the below code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<App />
);
reportWebVitals();
The App.js file contains the below code:
import './App.css';
import Form from './Form';
function App() {
return (
<div className="App">
<Form></Form>
</div>
);
}
export default App;
The Form.js contains the below code:
import React from 'react';
import { Formik } from 'formik';
import * as Yup from 'yup';
class Form extends React.Component {
render() {
return (
<section>
<h2>Cancel Inputs with Formik</h2>
<Formik
initialValues={{ FullName: '', Contact: '' }}
validationSchema={Yup.object({
FullName: Yup.string(),
Contact: Yup.number()
})}
onSubmit={(values ) => {
console.log('Hi :', values);
}}>
{
formik => (
<form onSubmit={formik.handleSubmit}>
<label htmlFor="FullName">Full Name</label>
<input
type="text"
id="FullName"
onChange={formik.handleChange}
value={formik.values.FullName}
></input>
<label htmlFor="Contact">Contact</label>
<input
type="text"
id="Contact"
onChange={formik.handleChange}
value={formik.values.Contact}
></input>
<button type="submit" value="Send">Send</button>
<button type="reset" value="Cancel" onClick={formik.resetForm}>Cancel</button>
</form>
)
}
</Formik>
</section>
)
}
}
export default Form;
Now run the application with npm start command and you can see the form render on the page. Fill the Form and click on send the value is logged in the console and if click on cancel the inputs are cleared.
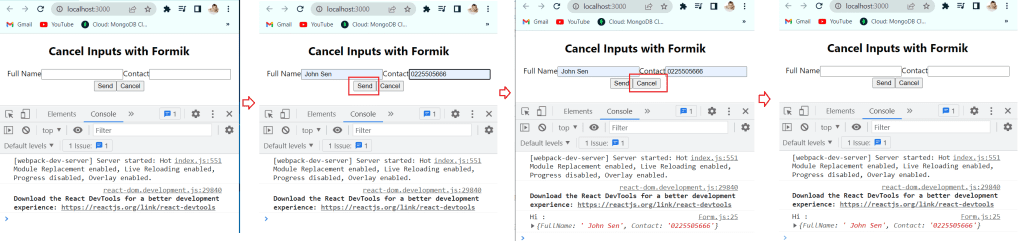
Conclusion
Through this react js tutorial, we learned how to create react cancel button, also we discussed the below points:
- React js cancel button
- Cancel and save button in react js
- React js how to build a edit cancel text input
- oncancel react
- Formik cancel button
- React cancel button event
You may also like:
- Handling Events in React js
- State in React js Hooks
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com