In this typescript tutorial, we will see how to convert string to keyOf in typescript. We will see different examples to convert string to keyOf in typescript.
Here are the topics we are going to cover:
- What is keyOf in typescript?
- Different examples to convert string to keyof in typescript
- Converting a string to keyOf type
- Using an object literal with an index signature
- Using the
as
keyword to assert a string literal type - Using a type assertion to convert a string to a union of string literal types
What is keyOf in typescript?
The keyOf in typescript is a keyword, which is used to create a union type of all keys in an object type. When you want to create a variable that can only have a value that is a key of a specified object type, this might be beneficial.
Let’s see an example of how we can use keyOf in typescript.
For example, we will define an interface as a Candidate, with three properties name
(of type string), age (of type number), and gender (of type ‘male’ | ‘female’ | ‘other’).
Then we will use the keyof keyword to create a type CandidateKey which is a union of all the keys in the Person interface (“name”, “age”, and “gender”).
Next, we will define a variable candidate of type Candidate with values for each property. After that, we define a function ‘getProperty‘ that accepts a Candidate object and a key of type keyof Candidate and returns the value of the property with that key.
Lastly, we will call the ‘getProperty‘ function with the candidate object and each of the keys in CandidateKey, and the type of the returned value is correctly inferred based on the type of the corresponding property in the Candidate interface.
Open the code editor, and create a file named ‘keyOf.ts’ and write the below code:
interface Candidate {
name:string;
age: number;
gender: 'male' | 'female' | 'other';
}
type CandidateKey = keyof Candidate; // type is "name" | "age" | "gender"
const candidate: Candidate = {
name: "Alex",
age: 30,
gender: "other",
};
function getProperty(obj: Candidate, key: CandidateKey) {
return obj[key];
}
const candidateName = getProperty(candidate, "name"); // type of name is string
const candidateAge = getProperty(candidate, "age"); // type of age is number
const candidateGender = getProperty(candidate, "gender"); // type of gender is "male" | "female" | "other"
console.log(candidate);
console.log(candidateName);
console.log(candidateAge);
console.log(candidateGender);
To compile the code, run the below command and you can see the result in the console.
ts-node keyOf
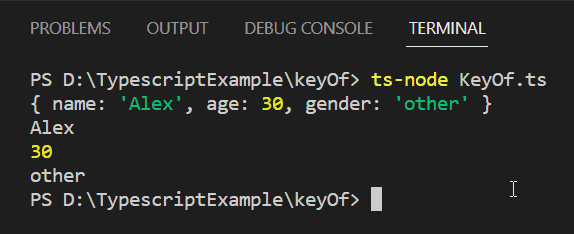
This is how we can use keyOf in typescript.
Different methods to convert string to keyof in typescript
Here we will see different examples to convert string to keyOf in typescript.
Example 1: Converting a string to keyOf type
For example, we will define an interface as Candidate, with three properties name
(of type string), age (of type number), and gender (of type ‘male’ | ‘female’ | ‘other’).
We will create a function ‘getProperty‘ which is called with an object obj of type T and a key ‘key’ of type ‘K’ that extends the keyof ‘T’. The value of the property with key ‘key’ in the object obj is returned by the getProperty function.
To pass a string as the key parameter to ‘getProperty‘, we must first confirm that the string is a valid Candidate type key. We can do this by defining a variable propertyName with the value “name,” which is a suitable key for the Candidate type. We can then use this variable as the key parameter to getProperty.
At last, when we execute getProperty(candidate, propertyName), TypeScript infers that the type of propertyName is “name,” which is a string literal type that may be assigned to keyof Person.
As a result, TypeScript can check that the key passed to getProperty is a valid key of the Candidate type and that the return type of getProperty is inferred correctly to be a string.
In the keyOf.ts file write the below code:
interface Candidate {
name: string;
age: number;
}
function getProperty<T, K extends keyof T>(obj: T, key: K) {
return obj[key];
}
const candidate: Candidate = { name: "Alice", age: 30 };
const propertyName = "name";
const candidateName = getProperty(candidate, propertyName); // type of name is string
console.log(candidateName);
To compile the code run the below command and you can see the result in the console.
ts-node keyOf
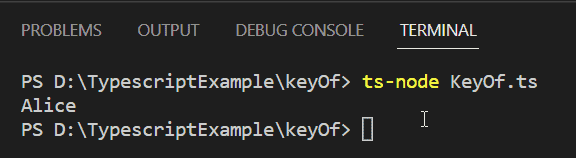
This is a simple example of how we can convert string to keyOf type in typescript.
Example 2: Using an object literal with an index signature
Here we will see how we can use an object literal with an index signature to convert string to keyOf type.
For example, we will declare a type ‘Candidate’ which is a union of string literal types “Alex”, “John”, and “Ron”. Next, we will define a variable candidate of type ‘Candidate’ and assign the value “Alex” to it.
The variable ages are then declared using an index signature that maps each key in Candidate to a number. We may obtain the age of a certain candidate by indexing into ages with the variable candidate. Because the age object in Candidate includes a number value for each key, the type of the age variable is assumed to be a number.
In the keyOf.ts file write the below code:
type Candidate = "Alex" | "John" | "Ron";
const candidate: Candidate = "Alex";
const ages: { [key in Candidate]: number } = {
Alex: 24,
John: 28,
Ron: 30,
};
const age = ages[candidate]; // type of age is number
console.log(age);
To compile the code run the below command and you can see the result in the console.
ts-node keyOf
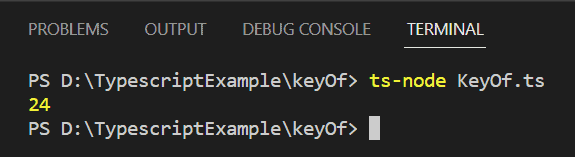
This is an example of converting string to keyOf type in typescript using an object literal with an index signature.
Example 3: Using the as
keyword to assert a string literal type
Here we will see an example of converting string to keyOf type in typescript by using the as
keyword to assert a string literal type.
For example, we define an interface Candidate with three attributes: name, age, and gender. We next define a variable candidate of type Candidate and assign it an object literal with values for each property.
We then declare a variable key with the value “age” as the type keyof Candidate. We use the as keyword to verify that “age” is a valid Candidate key type.
At last, We can use the key variable to access the ‘age’ property of the candidate object. The type of the value variable is inferred to be number since the ‘age’ property of Candidate is of type number.
In the keyOf.ts file write the below code:
interface Candidate {
name:string;
age: number;
gender: 'male' | 'female' | 'other';
}
const candidate: Candidate = {
name: "Alex",
age: 30,
gender: "other",
};
const key = "age" as keyof Candidate;
const value = candidate[key];
console.log(value);
To compile the code run the below command and you can see the result in the console.
ts-node keyOf
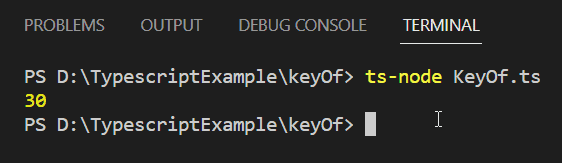
This is an example of how we can convert string to keyOf type using the as keyword to assert a string literal type in typescript.
Example 4: Using a type assertion to convert a string to a union of string literal types
Here we will see an example of converting string to keyOf type by using a type assertion to convert a string to a union of string literal types in typescript.
For example, we declare a type Candidate which is a union of string literal types “Alex”, “John”, and “Ron”. Next, we will define a variable candidate of type ‘Candidate‘ and assign the value “John” to it.
Then we will declare a variable ages with an index signature that maps each key in Candidate to a number. We can access the age of a specific candidate by indexing into ages with the candidate variable.
As the candidate is of type ‘Candidate’ and not keyof typeof ages , we need to use a type assertion to convert candidate to a keyof type that can be used to index into ages.
In the keyOf.ts file write the below code:
type Candidate = "Alex" | "John" | "Ron";
const candidate: Candidate = "John";
const ages: Record<Candidate, number> = {
Alex: 24,
John: 28,
Ron: 30,
};
const candidateAge = ages[candidate as keyof typeof ages];
console.log(candidateAge);
To compile the code run the below command and you can see the result in the console.
ts-node KeyOf.ts
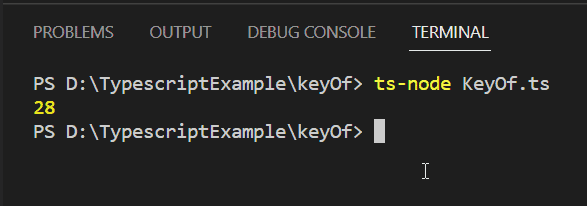
This is an example of how we can convert string to keyOf type by using a type assertion to convert a string to a union of string literal types.
Conclusion
In this typescript tutorial, we saw what is keyOf in typescript and then we discussed different examples to convert string to keyOf type in typescript. So, here are the topics we covered:
- What is keyOf in typescript?
- Different examples to convert string to keyof in typescript
- Converting a string to keyOf type
- Using an object literal with an index signature
- Using the
as
keyword to assert a string literal type - Using a type assertion to convert a string to a union of string literal types
You may like the following typescript tutorials:
- Typescript string capitalize first letter
- Typescript string union vs enum
- Typescript split string by dot
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com