Are you looking to sort a typescript array alphabetically? In this tutorial, I have explained different ways to sort an array alphabetically in Typescript.
To Sort an array alphabetically in Typescript is a breeze with the built-in sort() method. This method modifies the original array and arranges its elements in alphabetical order by default. For example, if you have an array of like [‘Chicago’, ‘San Francisco’, ‘New York’, ‘Boston’], you can simply call cities.sort() to get the array sorted as [‘Boston’, ‘Chicago’, ‘New York’, ‘San Francisco’].
TypeScript Sort Array Alphabetically
Now, let us check different methods to sort an array alphabetically in Typescript, starting with the sort() method.
Sort Array Alphabetically in Typescript using the Built-in sort() Function
TypeScript inherits JavaScript’s built-in sort() method for arrays, which can sort an array alphabetically by default. Here’s a simple example:
const cities: string[] = ['Chicago', 'San Francisco', 'New York', 'Boston'];
cities.sort();
console.log(cities);
You can see this here in the screenshot below; I run the code using Visual Studio Code, and after executing the program using ts-node arrayexamples.ts it sorts the array and the output as:
[ 'Boston', 'Chicago', 'New York', 'San Francisco' ]
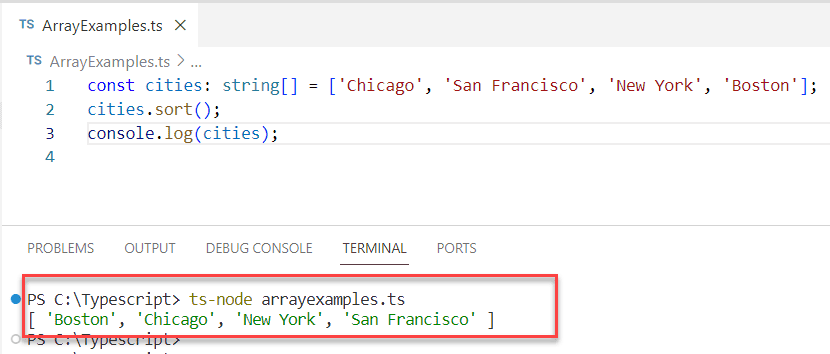
This is how to sort an array alphabetically using the sort() method in Typescript.
Case-Insensitive Sorting
If you have a Typescript array that contains both uppercase and lowercase characters, the default sort() function may not work as you expect. This is because the ASCII value of uppercase characters is less than that of lowercase characters.
To sort without considering the case, you can pass a custom comparison function to the sort() method in typescript.
Here is the code:
const mixedCaseCities: string[] = ['Chicago', 'san Francisco', 'new York', 'Boston'];
mixedCaseCities.sort((a, b) => a.toLowerCase().localeCompare(b.toLowerCase()));
console.log(mixedCaseCities); // Output: ['Boston', 'Chicago', 'new York', 'san Francisco']
Sort Array Alphabetically in Typescript using Custom Sorting Functions
We can also sort an array alphabetically in Typescript using custom sorting functions.
You can customize the sorting behavior by providing your own comparison function as an argument to the sort() method. The comparison function should return:
- A negative value if a should appear before b
- A positive value if a should appear after b
- Zero if a and b are equal
Here’s an example:
const customCities: string[] = ['Chicago', 'san Francisco', 'New York', 'Boston'];
customCities.sort((a, b) => {
const aLower = a.toLowerCase();
const bLower = b.toLowerCase();
if (aLower < bLower) return -1;
if (aLower > bLower) return 1;
return 0;
});
console.log(customCities); // Output: ['Boston', 'Chicago', 'New York', 'san Francisco']
Sort a Typescript Array Alphabetically using the manual sorting method
While the sort() function is convenient, sometimes you may want to implement your own sorting algorithm for better control. Below is an example using the Bubble Sort algorithm to sort an array alphabetically in Typescript.
Here is an example.
function bubbleSort(arr: string[]): string[] {
let len = arr.length;
for (let i = 0; i < len; i++) {
for (let j = 0; j < len - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
const manualCities: string[] = ['Chicago', 'San Francisco', 'New York', 'Boston'];
bubbleSort(manualCities);
console.log(manualCities);
The output will come like below:
['Boston', 'Chicago', 'New York', 'San Francisco']
You can see in the screenshot below I ran the code using Visual Studio Code.
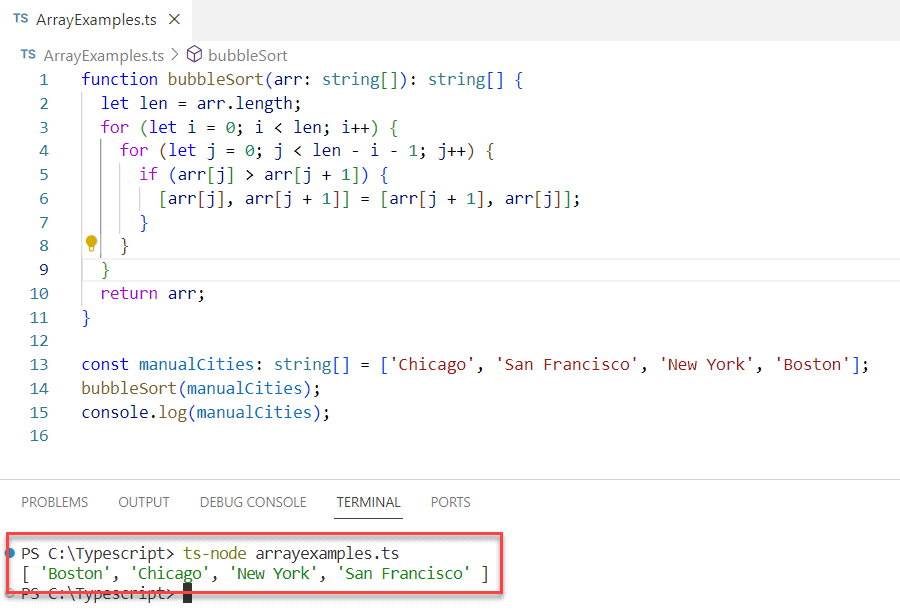
Conclusion
Sorting an array alphabetically in TypeScript is straightforward and flexible using multiple methods like the built-in sort() function, custom sorting functions, and even manual sorting methods like Bubble Sort. Now you know how to sort arrays alphabetically in TypeScript.
You may also like:
- How to compare strings for sorting in typescript
- How to map an array of objects to another array of objects in Typescript
- How to Convert Typescript Array to String With Separator
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com