Do you want to know about the array of objects in PowerShell? In this PowerShell tutorial, we will discuss what an array of objects is in PowerShell, how to declare one, and, most importantly, how to access an array of objects in PowerShell.
To access an array of objects in PowerShell, use index notation like $arrayOfObjects[index]
to retrieve an object, or loop through the array with foreach ($object in $arrayOfObjects) { }
to access each object. Object properties can be accessed with the dot notation, for example, $object.PropertyName
.
What is an Array of Objects in PowerShell?
An array is a data structure that holds a collection of items. In PowerShell, these items can be of any data type, including integers, strings, or objects. An array of objects, therefore, is a collection where each item is an object – which can be instances of custom classes or built-in types like PSCustomObject
, Hashtable
, or any other .NET objects.
Declaring an Array of Objects in PowerShell
To declare an array of objects in PowerShell, you can simply assign multiple objects to a variable. Let’s start with a basic example:
$object1 = New-Object -TypeName PSObject -Property @{
Name = "Object1"
Value = 10
}
$object2 = New-Object -TypeName PSObject -Property @{
Name = "Object2"
Value = 20
}
$arrayOfObjects = @($object1, $object2)
In this example, $arrayOfObjects
is an array that contains two objects, $object1
and $object2
.
Accessing Objects in an Array
Once you have an array of objects, you might want to access individual objects or iterate over them.
Access by Index
Each object in an array is associated with an index, starting with 0 for the first element. You can access an object by its index using the following syntax:
$object1 = New-Object -TypeName PSObject -Property @{
Name = "Object1"
Value = 10
}
$object2 = New-Object -TypeName PSObject -Property @{
Name = "Object2"
Value = 20
}
$arrayOfObjects = @($object1, $object2)
$firstObject = $arrayOfObjects[0] # Access the first object
$secondObject = $arrayOfObjects[1] # Access the second object
Iterating Over an Array
To perform operations on each object in an array, you can iterate over the array using a foreach
loop:
$object1 = New-Object -TypeName PSObject -Property @{
Name = "Object1"
Value = 10
}
$object2 = New-Object -TypeName PSObject -Property @{
Name = "Object2"
Value = 20
}
$arrayOfObjects = @($object1, $object2)
foreach ($obj in $arrayOfObjects) {
# Perform an action with $obj
Write-Host "Name: $($obj.Name), Value: $($obj.Value)"
}
Accessing Object Properties
To access the properties of objects within an array, you can use the dot notation:
$object1 = New-Object -TypeName PSObject -Property @{
Name = "Object1"
Value = 10
}
$object2 = New-Object -TypeName PSObject -Property @{
Name = "Object2"
Value = 20
}
$arrayOfObjects = @($object1, $object2)
foreach ($obj in $arrayOfObjects) {
$name = $obj.Name
$value = $obj.Value
Write-Host "Object Name: $name, Object Value: $value"
}
Once you execute the above PowerShell script, you can see the output in the screenshot below:
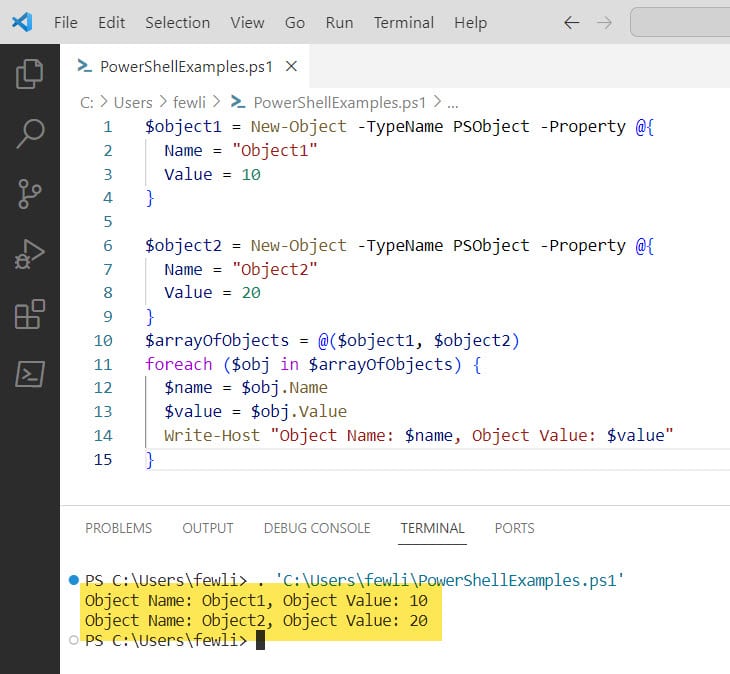
Modifying Objects in an Array
Modifying an object in an array is as straightforward as accessing it. You can change the property values of an object by accessing the object and using the dot notation:
$arrayOfObjects[0].Value = 30 # Change the value of the first object's 'Value' property
Adding and Removing Objects from an Array
PowerShell arrays are fixed in size, but you can work around this by creating a new array that includes the new elements or by using a collection type that allows for dynamic resizing, like an ArrayList
.
Adding an Object
$newObject = New-Object -TypeName PSObject -Property @{
Name = "Object3"
Value = 30
}
$arrayOfObjects += $newObject # Add a new object to the array
Removing an Object
Removing an object is not as straightforward since you cannot directly remove an item from an array. Instead, you can filter the array to exclude the object you want to remove:
$arrayOfObjects = $arrayOfObjects | Where-Object { $_.Name -ne "Object1" } # Remove object with Name 'Object1'
Sorting an Array of Objects
PowerShell also provides advanced methods to work with arrays, such as sorting and filtering based on object properties.
You can sort an array by a specific property using the Sort-Object
cmdlet:
$sortedArray = $arrayOfObjects | Sort-Object -Property Value # Sort the array by the 'Value' property
Filtering an Array of Objects
Similarly, you can filter an array to only include objects that meet certain criteria:
$filteredArray = $arrayOfObjects | Where-Object { $_.Value -gt 15 } # Include only objects with 'Value' greater than 15
Conclusion
In this PowerShell tutorial, I have explained everything about the PowerShell array of objects like:
- What is an Array of Objects in PowerShell?
- How to declare an Array of Objects in PowerShell
- How to access an array of objects in PowerShell?
- Sort an Array of Objects
- Filter an Array of Objects
You may also like:
- How to Pass Objects to Functions in PowerShell?
- How To Format An Array Of Objects As Table In PowerShell?
- How to Filter Array of Objects in PowerShell?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com