In this SPFx tutorial, we will see an office-ui-fabric-react combobox example. In this office-ui-fabric-react combobox demo, we will see how to bind combobox from the choice values from a choice column from a SharePoint Online list. We will see here, office-ui-fabric-react combobox single select as well as office-ui-fabric-react combobox multiselect. And also we will see how to get values on office-ui-fabric-react combobox onchange.
Then we will see how to save combobox values to the SharePoint Online list using pnp.
At the end of the demo, we can see something like below and when user select an item from the single select combobox or select multiple items from the Multi select combobox, and then click on the Submit button, the data will be saved to the SharePoint Online list.
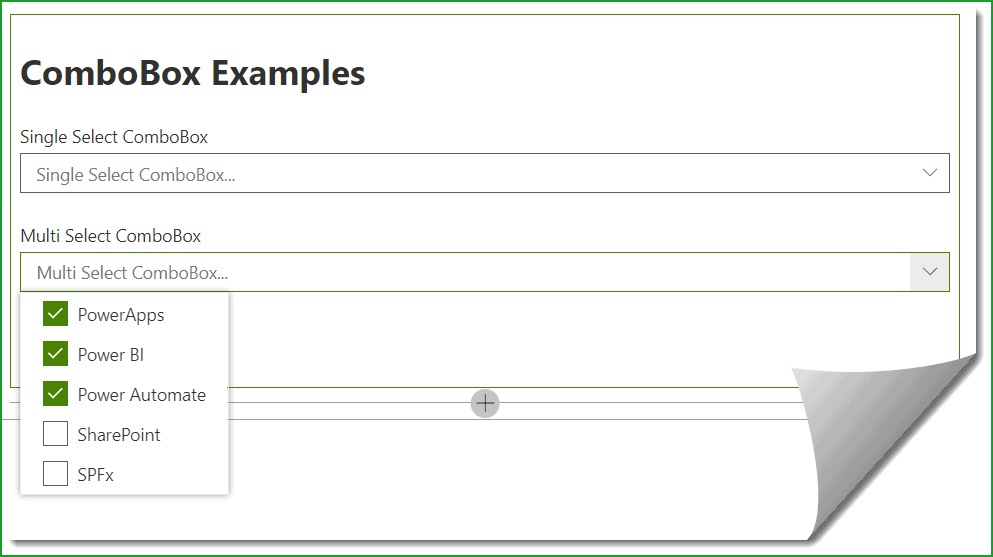
The data will be save to a SharePoint list and also the values of the office ui fabric combobox will be populated from the choices from the choices column.
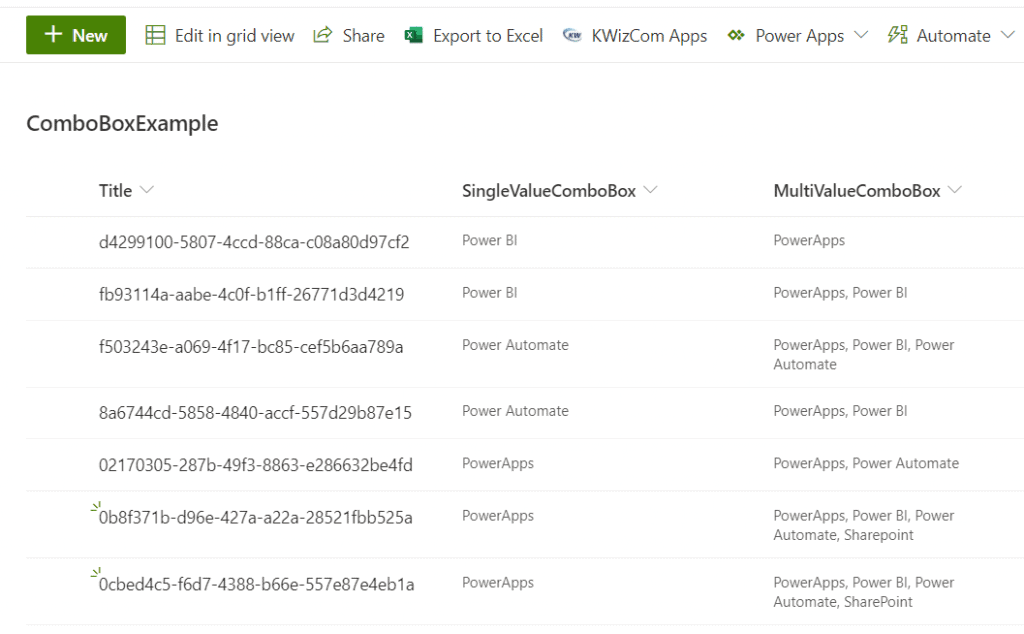
And also the office-ui-fabric-react combobox options will be loaded from the SharePoint Online list choice column like below:
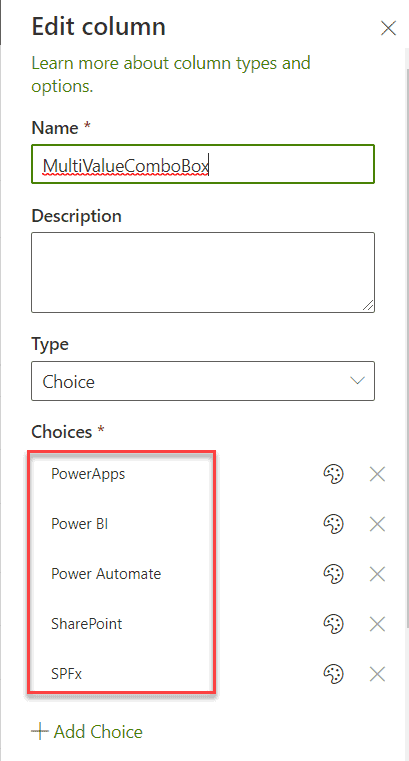
Now, let us create the SPFx solution and the example.
Before going further, make sure to set up your development environment for SharePoint Framework.
office-ui-fabric-react combobox example
Open Node.js command prompt in administrator mode. You can also use windows command prompt, PowerShell command prompts. The run the below command:
md FabricComboDemo
cd FabricComboDemo
Then in the same directory run the below command:
yo @microsoft/sharepoint
Now, it will ask you a few details like below:
- What is your solution name? Click enter to take the default value.
- Which baseline packages do you want to target for your component(s). You can choose from the below options
- SharePoint Online Only (latest)
- SharePoint 2016 onwards, including 2019 and SharePoint Online
- SharePoint 2019 onwards, including SharePoint Online
- Press Enter to choose the default one (SharePoint Online Only (latest).
- Where do you want to place the files?
- Use the current folder
- Create a subfolder with solution name, press enter to use the first option.
- Do you want to allow the tenant admin the choice of being able to deploy the solution to all sites immediately without running any feature deployment or adding apps in sites? Type N.
- Will the components in the solution require permissions to access web APIs that are unique and not shared with other components in the tenant. Type N.
- Which type of client-side component to create?
- WebPart
- Extension
- Library
- Here choose WebPart.
Next, it will ask you about the web part details.
- What is your web part name? FabricComboWP Click Enter
- What is your Web part description? FabricComboWP description Click Enter
- Which framework would you like to use? Select React from the below options:
- No JavaScript framework
- React
- Knockout
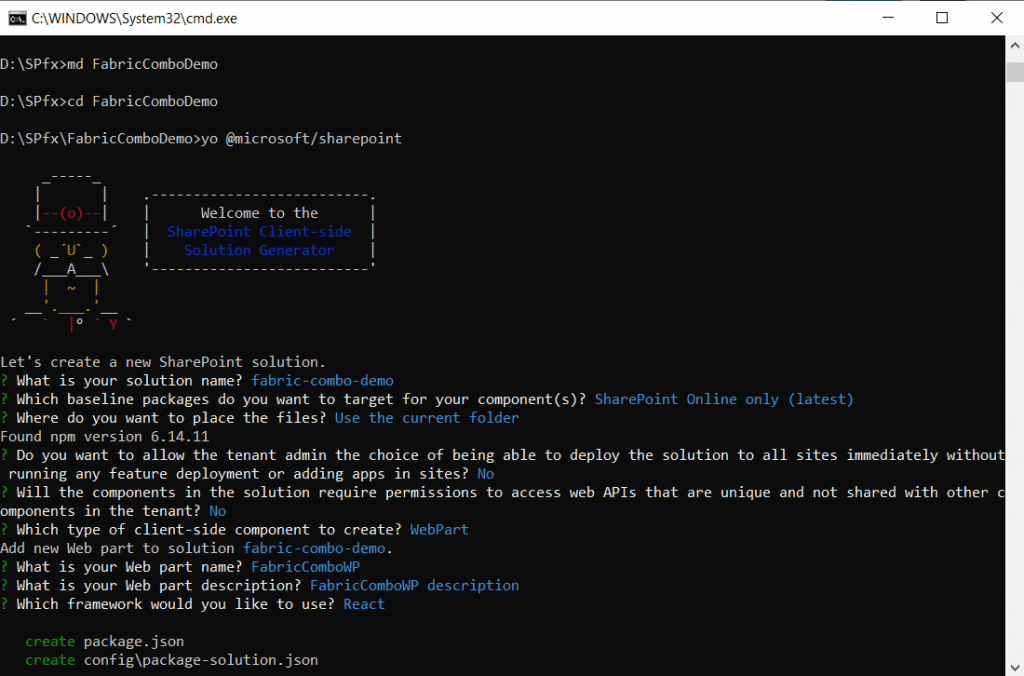
Then it will take sometime and create the solution, you can see the successful message like below:
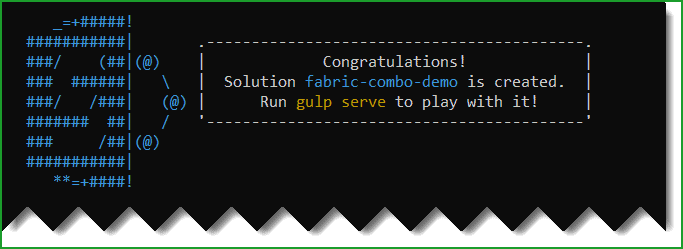
Here in this spfx combobox example, we will use PnP js to insert items to the list. For this, we need to install the pnp js library. For this run the below cmdlets.
npm i @pnp/sp

Once it is installed, open the solution using Visual studio code by running the below command.
code .
Bind spfx office-ui-fabric-react combobox items from SharePoint List Choice Column
Let us see how to bind office-ui-fabric-react combobox items from SharePoint List Choice Column in SharePoint Framework.
Open the IFabricUiComboBoxProps.ts file and add the below three properties.
webURL:string;
singleValueChoices:any;
multiValueChoices:any;
The interface looks like below:
export interface IFabricUiComboBoxProps {
description: string;
webURL:string;
singleValueChoices:any;
multiValueChoices:any;
}
Then open the FabricUiComboBoxWebPart.ts file and add the code like below:
As you can see here I have created a method as getChoiceFields where we are adding the choice options from the SharePoint list columns. Here SingleValueComboBox and MultiValueComboBox are my column names.
public async render(): Promise<void> {
const element: React.ReactElement<IFabricUiComboBoxProps> = React.createElement(
FabricUiComboBox,
{
description: this.properties.description,
webURL:this.context.pageContext.web.absoluteUrl,
singleValueChoices: await getChoiceFields(this.context.pageContext.web.absoluteUrl,'SingleValueComboBox'),
multiValueChoices: await getChoiceFields(this.context.pageContext.web.absoluteUrl,'MultiValueComboBox')
}
);
ReactDom.render(element, this.domElement);
}
export const getChoiceFields = async (webURL,field) => {
let resultarr = [];
await fetch(`${webURL}/_api/web/lists/GetByTitle('ComboBoxExample')/fields?$filter=EntityPropertyName eq '${field}'`, {
method: 'GET',
mode: 'cors',
credentials: 'same-origin',
headers: new Headers({
'Content-Type': 'application/json',
'Accept': 'application/json',
'Access-Control-Allow-Origin': '*',
'Cache-Control': 'no-cache',
'pragma': 'no-cache',
}),
}).then(async (response) => await response.json())
.then(async (data) => {
for (var i = 0; i < data.value[0].Choices.length; i++) {
await resultarr.push({
key:data.value[0].Choices[i],
text:data.value[0].Choices[i]
});
}
});
return await resultarr;
};
Then open the FabricUiComboBox.tsx file which is the main component file. First, we need to write the import statements.
import { ComboBox, IComboBoxOption, IComboBox, PrimaryButton } from 'office-ui-fabric-react/lib/index';
import { Web } from "@pnp/sp/presets/all";
import "@pnp/sp/lists";
import "@pnp/sp/items";
import { getGUID } from "@pnp/common";
Then write the below interface:
export interface IStates {
SingleSelect: any;
MultiSelect: any;
}
The render() method where we have added the controls looks like below:
public render(): React.ReactElement<IFabricUiComboBoxProps> {
return (
<div>
<h1>ComboBox Examples</h1>
<ComboBox
placeholder="Single Select ComboBox..."
selectedKey={this.state.SingleSelect}
label="Single Select ComboBox"
autoComplete="on"
options={this.props.singleValueChoices}
onChange={this.onComboBoxChange}
/>
<br />
<ComboBox
placeholder="Multi Select ComboBox..."
selectedKey={this.state.MultiSelect}
label="Multi Select ComboBox"
autoComplete="on"
multiSelect
options={this.props.multiValueChoices}
onChange={this.onComboBoxMultiChange}
/>
<div>
<br />
<br />
<PrimaryButton onClick={() => this.Save()}>Submit</PrimaryButton>
</div>
</div>
);
}
public onComboBoxChange = (ev: React.FormEvent<IComboBox>, option?: IComboBoxOption): void => {
this.setState({ SingleSelect: option.key });
}
public onComboBoxMultiChange = async (event: React.FormEvent<IComboBox>, option: IComboBoxOption): Promise<void> => {
if (option.selected) {
await arr.push(option.key);
}
else {
await arr.indexOf(option.key) !== -1 && arr.splice(arr.indexOf(option.key), 1);
}
await this.setState({ MultiSelect: arr });
}
The constructor looks like below:
constructor(props) {
super(props);
this.state = {
SingleSelect: "",
MultiSelect: []
};
}
The Save button code looks like below:
private async Save() {
let web = Web(this.props.webURL);
await web.lists.getByTitle("ComboBoxExample").items.add({
Title: getGUID(),
SingleValueComboBox: this.state.SingleSelect,
MultiValueComboBox: { results: this.state.MultiSelect }
}).then(i => {
console.log(i);
});
alert("Submitted Successfully");
}
Below is the complete code for FabricUiComboBox.tsx
import * as React from 'react';
import { IFabricUiComboBoxProps } from './IFabricUiComboBoxProps';
import { ComboBox, IComboBoxOption, IComboBox, PrimaryButton } from 'office-ui-fabric-react/lib/index';
import { Web } from "@pnp/sp/presets/all";
import "@pnp/sp/lists";
import "@pnp/sp/items";
import { getGUID } from "@pnp/common";
var arr = [];
export interface IStates {
SingleSelect: any;
MultiSelect: any;
}
export default class FabricUiComboBox extends React.Component<IFabricUiComboBoxProps, IStates> {
constructor(props) {
super(props);
this.state = {
SingleSelect: "",
MultiSelect: []
};
}
private async Save() {
let web = Web(this.props.webURL);
await web.lists.getByTitle("ComboBoxExample").items.add({
Title: getGUID(),
SingleValueComboBox: this.state.SingleSelect,
MultiValueComboBox: { results: this.state.MultiSelect }
}).then(i => {
console.log(i);
});
alert("Submitted Successfully");
}
public onComboBoxChange = (ev: React.FormEvent<IComboBox>, option?: IComboBoxOption): void => {
this.setState({ SingleSelect: option.key });
}
public onComboBoxMultiChange = async (event: React.FormEvent<IComboBox>, option: IComboBoxOption): Promise<void> => {
if (option.selected) {
await arr.push(option.key);
}
else {
await arr.indexOf(option.key) !== -1 && arr.splice(arr.indexOf(option.key), 1);
}
await this.setState({ MultiSelect: arr });
}
public render(): React.ReactElement<IFabricUiComboBoxProps> {
return (
<div>
<h1>ComboBox Examples</h1>
<ComboBox
placeholder="Single Select ComboBox..."
selectedKey={this.state.SingleSelect}
label="Single Select ComboBox"
autoComplete="on"
options={this.props.singleValueChoices}
onChange={this.onComboBoxChange}
/>
<br />
<ComboBox
placeholder="Multi Select ComboBox..."
selectedKey={this.state.MultiSelect}
label="Multi Select ComboBox"
autoComplete="on"
multiSelect
options={this.props.multiValueChoices}
onChange={this.onComboBoxMultiChange}
/>
<div>
<br />
<br />
<PrimaryButton onClick={() => this.Save()}>Submit</PrimaryButton>
</div>
</div>
);
}
}
Once the code is ready, you can run the below cmdlet that will open the local workbench.
gulp serve
While the local workbench is running, you can run the SharePoint workbench and add the web part.
https://tsinfo.sharepoint.com/sites/SPFxBlog/_layouts/15/workbench.aspx
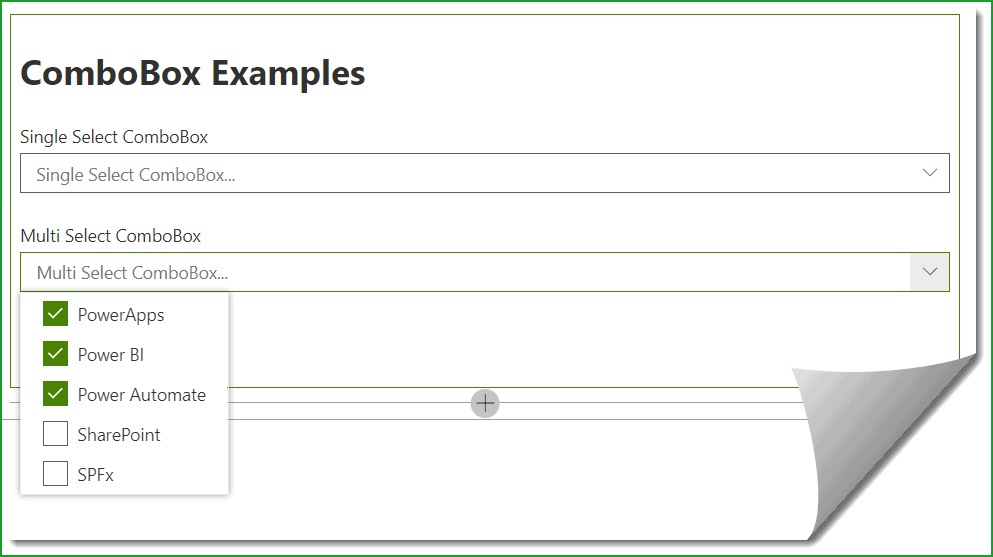
If you want to deploy the spfx client side web part to the SharePoint app catalog site, then run the below commands to create the .sppkg file.
gulp bundle --ship
gulp package-solution --ship
Download Solution office-ui-fabric-react combobox
You can download the complete SPFx solution from the below link:
Once you download the solution, unzip it and run the below command before running gulp serve.
npm i
npm i @pnp/sp
Video tutorial on office-ui-fabric-react combobox example
I have recorded a complete video tutorial on office-ui-fabric-react combobox example and uploaded to the YouTube Channel.
You may like the following SPFx tutorials:
- SharePoint framework client web part example
- SharePoint client-side web part configurable properties in Property Pane using spfx
- How to Create Site Columns, Content Type and Custom List using SharePoint Framework
- Retrieve SharePoint list items using SharePoint framework (SPFx)
- SharePoint Framework CRUD Operations using React
- SharePoint framework crud operations no javascript framework
- What is Microsoft Fluent UI
- How to get user details using Microsoft Graph API in SPFx
- SPFx – Bind dropdown list from SharePoint list using PnP
In this tutorial, we learned office-ui-fabric-react combobox example. We saw how we can create a SPFx web part and also how to save the combobox selection options to a SharePoint Online list. Here, we will see, how to select multiple items from the office-ui-fabric-react combobox.
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com