In this spfx tutorial, we will discuss how to display SharePoint list items using SPFx (SharePoint Framework). We will see two examples, one is how to get SharePoint list items using spfx react and another example, we will see how to display sharepoint list items in a spfx web part using no javascript framework and typescript.
SharePoint Framework is the new development model and it is a page and Web part model which provides full support for client-side SharePoint development, easy integration with SharePoint data, and support for open-source tooling.
With SharePoint Framework, you can use modern Web technologies and the tools in your preferred development environment to build productive experiences and apps in SharePoint.
Retrieve SharePoint list items using SharePoint framework
If you have not set up the development environment, check out an article on How to Set up SharePoint Framework development environment.
Follow the below steps to spfx webpart to display list items using SharePoint Rest Interface and Typescript.
Create SPFx Solution
Step 1: Create a new Solution for example “GetSPListItems”
- Open the command prompt. Create a directory for SPFx solution.
- md GetSPListItems
- Navigate to the above-created directory
- cd GetSPListItems
Step 2: Run Yeoman SharePoint Generator to create the solution.
yo @microsoft/sharepoint
Yeoman generator will present you with the wizard by asking questions about the solution to be created.
- Solution Name: Hit Enter to have a default name (GetSPListItems in this case) or type in any other name for your solution.
- Target for component: Here, we can select the target environment where we are planning to deploy the client web part; i.e., SharePoint Online or SharePoint OnPremise (SharePoint 2016 onwards).Selected choice – SharePoint Online only (latest).
- Place of files: We may choose to use the same folder or create a subfolder for our solution.Selected choice – Same folder.
- Deployment option: Selecting Y will allow the app to be deployed instantly to all sites and will be accessible everywhere. Select choice – N (install on each site explicitly)
- Type of client-side component to create: We can choose to create client-side web part or an extension. Choose the web part option. Select option – WebPart.
- Web part name: Hit enter to select the default name or type in any other name. Type name – getSPListItems
- Web part description: Hit enter to select the default description or type in any other value.
- Framework to use: Select any JavaScript framework to develop the component. Available choices are No JavaScript Framework, React, and Knockout. Select option – No JavaScript Framework
Step 3: In the command prompt type the below command to open the solution in vs code editor-> ” code .“
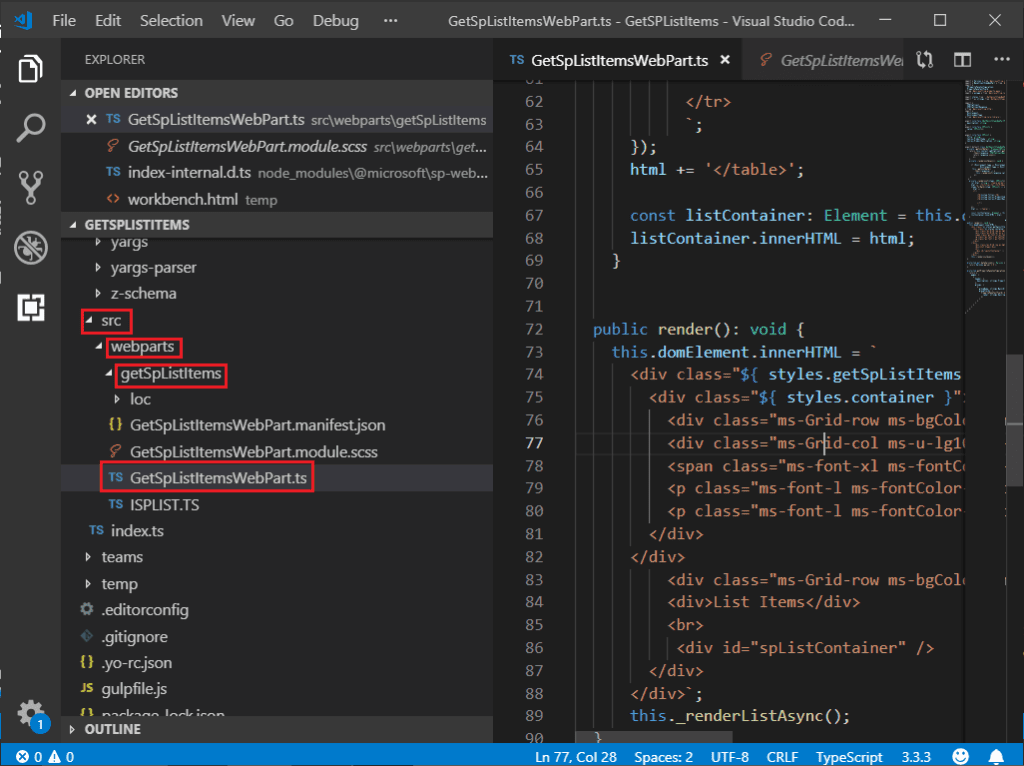
Step 4: go to the “GetSPListItems.ts” file, copy and paste the below code.
import { Version } from '@microsoft/sp-core-library';
import { BaseClientSideWebPart } from '@microsoft/sp-webpart-base';
import {
IPropertyPaneConfiguration,
PropertyPaneTextField
} from '@microsoft/sp-property-pane';
import { escape } from '@microsoft/sp-lodash-subset';
import styles from './GetSpListItemsWebPart.module.scss';
import * as strings from 'GetSpListItemsWebPartStrings';
import {
SPHttpClient,
SPHttpClientResponse
} from '@microsoft/sp-http';
import {
Environment,
EnvironmentType
} from '@microsoft/sp-core-library';
export interface IGetSpListItemsWebPartProps {
description: string;
}
export interface ISPLists {
value: ISPList[];
}
export interface ISPList {
Title: string;
Conros_ProductCode : string;
Conros_ProductDescription :string;
}
export default class GetSpListItemsWebPart extends BaseClientSideWebPart<IGetSpListItemsWebPartProps> {
private _getListData(): Promise<ISPLists> {
return this.context.spHttpClient.get(this.context.pageContext.web.absoluteUrl + "/_api/web/lists/GetByTitle('Conros Products')/Items",SPHttpClient.configurations.v1)
.then((response: SPHttpClientResponse) => {
return response.json();
});
}
private _renderListAsync(): void {
if (Environment.type == EnvironmentType.SharePoint ||
Environment.type == EnvironmentType.ClassicSharePoint) {
this._getListData()
.then((response) => {
this._renderList(response.value);
});
}
}
private _renderList(items: ISPList[]): void {
let html: string = '<table border=1 width=100% style="border-collapse: collapse;">';
html += '<th>Title</th> <th>Product Code</th><th>Product Description</th>';
items.forEach((item: ISPList) => {
html += `
<tr>
<td>${item.Title}</td>
<td>${item.Conros_ProductCode}</td>
<td>${item.Conros_ProductDescription}</td>
</tr>
`;
});
html += '</table>';
const listContainer: Element = this.domElement.querySelector('#spListContainer');
listContainer.innerHTML = html;
}
public render(): void {
this.domElement.innerHTML = `
<div class="${ styles.getSpListItems }">
<div class="${ styles.container }">
<div class="ms-Grid-row ms-bgColor-themeDark ms-fontColor-white ${ styles.row }">
<div class="ms-Grid-col ms-u-lg10 ms-u-xl8 ms-u-xlPush2 ms-u-lgPush1">
<span class="ms-font-xl ms-fontColor-white">Welcome to SharePoint Modern Developmennt</span>
<p class="ms-font-l ms-fontColor-white">Loading from ${this.context.pageContext.web.title}</p>
<p class="ms-font-l ms-fontColor-white">Retrive Data from SharePoint List</p>
</div>
</div>
<div class="ms-Grid-row ms-bgColor-themeDark ms-fontColor-white ${styles.row}">
<div>List Items</div>
<br>
<div id="spListContainer" />
</div>
</div>`;
this._renderListAsync();
}
protected get dataVersion(): Version {
return Version.parse('1.0');
}
protected getPropertyPaneConfiguration(): IPropertyPaneConfiguration {
return {
pages: [
{
header: {
description: strings.PropertyPaneDescription
},
groups: [
{
groupName: strings.BasicGroupName,
groupFields: [
PropertyPaneTextField('description', {
label: strings.DescriptionFieldLabel
})
]
}
]
}
]
};
}
}
Step5: In the command prompt, type “gulp serve”.
Step 6: Go to sharepoint workbench URL is
https://domain-name/sites/site collection name/_layouts/15/workbench.aspx
https://tsinfotechnologies.sharepoint.com/sites/SPFxForBlog/_layouts/15/workbench.aspx
Step 7: In the SharePoint local workbench page, add the web part. SharePoint list items will be displayed in the web part.
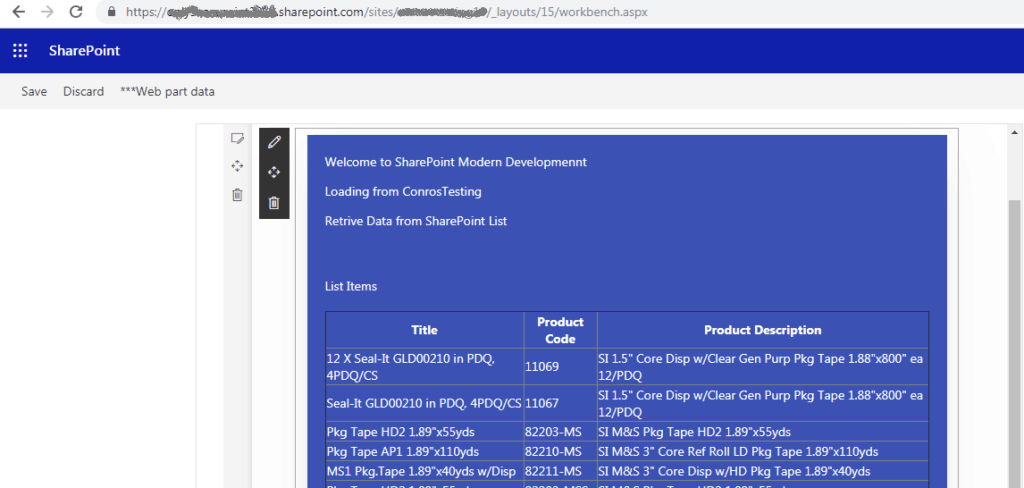
This is how to get sharepoint list items using typescript in spfx and display list items using spfx in an HTML table in SharePoint Online.
Let us check another example of how to display list items using SPFx react. We will create an spfx webpart to display list items in SharePoint Online modern site.
Read SPFx Send Email using PnP
Get sharepoint list items using reactjs spfx
Let us see, how to retrieve sharepoint list items using spfx react and then display sharepoint list items in a spfx web part using DetailsList, Microsoft fluent UI control.
Here I have a custom SharePoint list which has the below columns:
- CustomerName
- ProductName
- OrderDate
- ProductDescription
- ProductImage
It also has a few items added and the list looks like below:
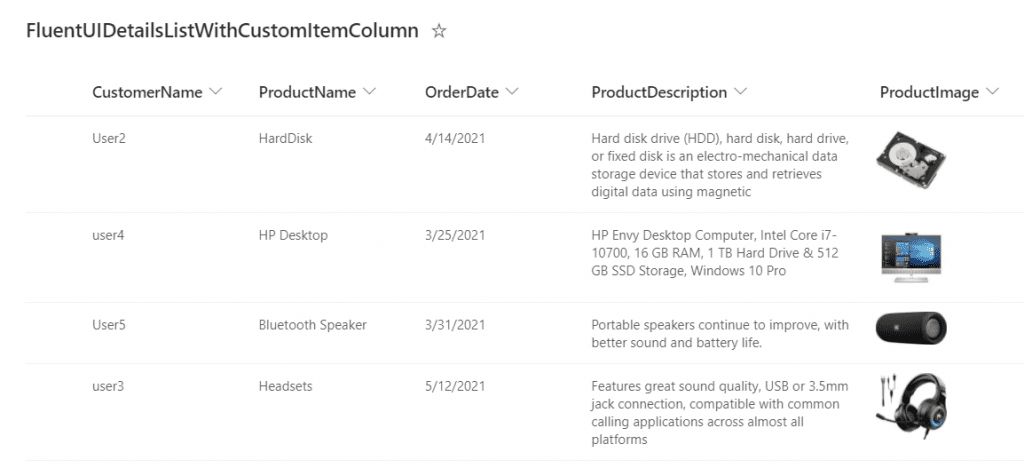
We will use this list to display sharepoint list items in a spfx web part.
Follow the above steps to create a client-side web part using SPFx and make sure to choose react framework.
Once you created the SPFx solution, the project structure looks like below:
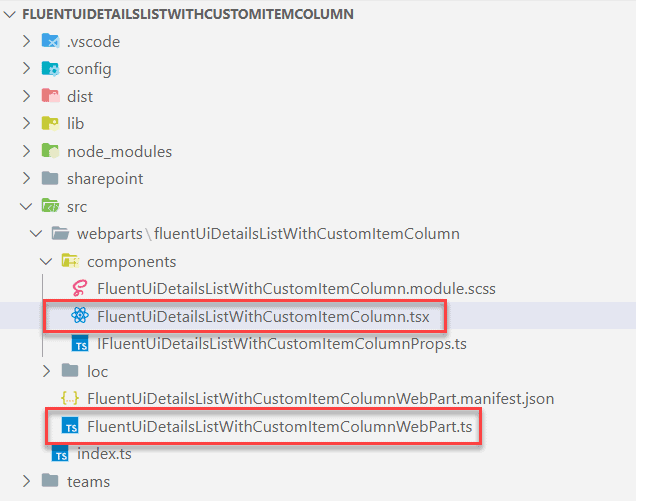
Here we will modify a few lines of code like below:
In the IFluentUiDetailsListWithCustomItemColumnProps.ts file, add one more property like below:
export interface IFluentUiDetailsListWithCustomItemColumnProps {
description: string;
webURL:string;
}
In the FluentUiDetailsListWithCustomItemColumnWebPart.ts file, modify the render() method like below:
public render(): void {
const element: React.ReactElement<IFluentUiDetailsListWithCustomItemColumnProps> = React.createElement(
FluentUiDetailsListWithCustomItemColumn,
{
description: this.properties.description,
webURL:this.context.pageContext.web.absoluteUrl,
}
);
ReactDom.render(element, this.domElement);
}
Here we need the absoluteUrl to communicate with the SharePoint site.
Here our main file is the .tsx file and below is the code.
FluentUiDetailsListWithCustomItemColumn.tsx
Here, we will use the DetailsList fluent UI control.
import * as React from 'react';
import { IFluentUiDetailsListWithCustomItemColumnProps } from './IFluentUiDetailsListWithCustomItemColumnProps';
import { Web } from "@pnp/sp/presets/all";
import {IColumn, DetailsList, SelectionMode, DetailsListLayoutMode, mergeStyles, Link,Image,ImageFit} from '@fluentui/react';
import "@pnp/sp/lists";
import "@pnp/sp/items";
export interface ICustomColumnDetailsListStates {
Items: IDocument[];
columns: any;
isColumnReorderEnabled: boolean;
}
export interface IDocument {
CustomerName: string;
CustomerEmail: string;
ProductName: string;
OrderDate: any;
ProductDescription: any;
ProductImage: string;
}
export default class FluentUiDetailsListWithCustomItemColumn extends React.Component<IFluentUiDetailsListWithCustomItemColumnProps, ICustomColumnDetailsListStates> {
constructor(props) {
super(props);
const columns: IColumn[] = [
{
key: "ProductImage",
name: "",
fieldName: "ProductImage",
minWidth: 70,
maxWidth: 90,
isResizable: true,
data: "string",
isPadded: true,
},
{
key: "ProductName",
name: "Product Name",
fieldName: "ProductName",
minWidth: 70,
maxWidth: 90,
isResizable: true,
data: "string",
isPadded: true,
},
{
key: "CustomerName",
name: "Customer Name",
fieldName: "CustomerName",
minWidth: 70,
maxWidth: 90,
isRowHeader: true,
isResizable: true,
data: "string",
isPadded: true
},
{
key: "CustomerEmail",
name: "Customer Email",
fieldName: "CustomerEmail",
minWidth: 70,
maxWidth: 90,
isResizable: true,
data: "number",
isPadded: true
},
{
key: "OrderDate",
name: "Order Date",
fieldName: "OrderDate",
minWidth: 70,
maxWidth: 90,
isResizable: true,
data: "string",
},
{
key: "ProductDescription",
name: "Product Description",
fieldName: "ProductDescription",
minWidth: 210,
maxWidth: 350,
isResizable: true,
data: "string",
}
];
this.state = {
Items: [],
columns: columns,
isColumnReorderEnabled: true,
};
}
public async componentWillMount() {
await this.getData();
}
public async getData() {
const data: IDocument[] = [];
let web = Web(this.props.webURL);
const items: any[] = await web.lists.getByTitle("FluentUIDetailsListWithCustomItemColumn").items.select("*", "CustomerName/EMail", "CustomerName/Title").expand("CustomerName/ID").get();
console.log(items);
await items.forEach(async item => {
await data.push({
CustomerName: item.CustomerName.Title,
CustomerEmail: item.CustomerName.EMail,
ProductName: item.ProductName,
OrderDate: FormatDate(item.OrderDate),
ProductDescription: item.ProductDescription,
ProductImage: window.location.origin + item.ProductImage.match('"serverRelativeUrl":(.*),"id"')[1].replace(/['"]+/g, '')
});
});
console.log(data);
await this.setState({ Items: data });
console.log(this.state.Items);
}
public _onRenderItemColumn = (item: IDocument, index: number, column: IColumn): JSX.Element | string => {
const src = item.ProductImage;
switch (column.key) {
case 'ProductImage':
return(
<a href={item.ProductImage} target="_blank">
<Image src={src} width={50} height={50} imageFit={ImageFit.cover} />
</a>) ;
case 'ProductName':
return (
<span
data-selection-disabled={true}
style={{whiteSpace:'normal'}}
>
{item.ProductName}
</span>
);
case 'CustomerName':
return <Link style={{whiteSpace:'normal'}} href="#">{item.CustomerName}</Link>;
case 'CustomerEmail':
return <span style={{whiteSpace:'normal'}}>{item.CustomerEmail}</span>;
case 'OrderDate':
return (
<span
data-selection-disabled={true}
className={mergeStyles({ height: '100%', display: 'block' })}
>
{item.OrderDate}
</span>
);
case 'ProductDescription':
return (
<span
data-selection-disabled={true}
style={{whiteSpace:'normal'}}
>
{item.ProductDescription}
</span>
);
default:
return <span>{item.CustomerName}</span>;
}
}
public render(): React.ReactElement<IFluentUiDetailsListWithCustomItemColumnProps> {
return (
<div>
<h1>Display SharePoint list data using spfx</h1>
<DetailsList
items={this.state.Items}
columns={this.state.columns}
setKey="set"
layoutMode={DetailsListLayoutMode.justified}
isHeaderVisible={true}
onRenderItemColumn={this._onRenderItemColumn}
selectionMode={SelectionMode.none}
/>
<h3>Note : Image is clickable.</h3>
</div>
);
}
}
export const FormatDate = (date): string => {
var date1 = new Date(date);
var year = date1.getFullYear();
var month = (1 + date1.getMonth()).toString();
month = month.length > 1 ? month : '0' + month;
var day = date1.getDate().toString();
day = day.length > 1 ? day : '0' + day;
return month + '/' + day + '/' + year;
};
Once the code is ready, run the below command to open the local workbench.
gulp serve
While the local workbench is running, open the SharePoint workbench on the same site, where your list is there.
https://tsinfotechnologies.sharepoint.com/sites/SPFxForBlog/_layouts/15/workbench.aspx
Here add the web part and you can see the output lie below:
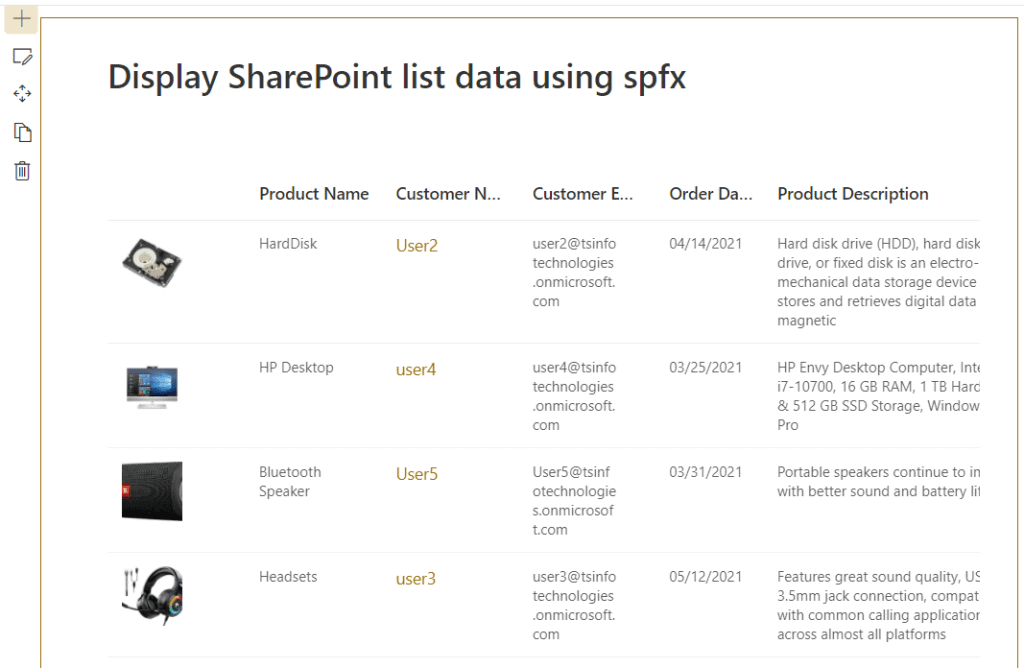
Once it is ready, we can deploy the SPFx web part to the SharePoint online app catalog site.
Run the below commands:
gulp bundle --ship
gulp package-solution --ship
This will create the package file (.sppkg) which we need to deploy to the SharePoint Online app catalog site.
This is how to retrieve sharepoint list items using spfx react.
Download SPFx Solution
You can download the complete solution from the below URL and then run the below command.
npm i
You may also like the following SPFx tutorials:
- SPFx Fluent UI Basic List Example
- office-ui-fabric-react combobox example in SharePoint Framework (SPFx)
- SPFx fluent UI react dropdown example
- How to add new webpart in spfx solution
- SharePoint Framework CRUD Operations using React
- SharePoint framework crud operations no javascript framework
- cannot find module jquery spfx
- error TS1192: Module has no default export
In this SPFx tutorial, we learned, how to get sharepoint list items using reactjs spfx and how to display sharepoint list items in an spfx web part.
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com
Thank you so much
Please share the code in GitHub ..
const items: any[] = await web.lists.getByTitle(“FluentUIDetailsListWithCustomItemColumn”).items.select(“*”, “CustomerName/EMail”, “CustomerName/Title”).expand(“CustomerName/ID”).get();
Not Working
Thats a great tutorial. Thanks
If I wanted to get just one item, say where title = hello, how can this be achieved?