Do you want to check type in Typescript? In this Typescript tutorial, I have explained different methods to check the type of variables in TypeScript. Check out the complete tutorial on Typescript check type with examples.
To check type in Typescript, you can use the typeof operator, instanceof operator, and custom type guards methods. By using these methods, you can verify whether a variable is a string, number, boolean, object, function, symbol, array, or any other type. For example, you can use (typeof city) to find out city is of string data type.
Check type in Typescript
When you write code in TypeScript, you can specify the types of your variables and function parameters, which enables TypeScript to perform static type checking. This means that TypeScript will check your code for type errors before it is compiled into JavaScript. If any type of errors are found, the compilation process will fail, and the errors will be reported to you, helping you catch mistakes early in the development process.
TypeScript type checking is important because it helps to ensure that your code is reliable and behaves as expected. By catching type errors before your code is compiled and run, you can avoid many common programming mistakes and runtime errors. This can help to reduce the number of bugs in your code, making it easier to maintain and debug.
Now, let us see how to check type in typescript using different methods with examples.
1. Check type typescript using the typeof Operator
The typeof operator is a straightforward way to determine the data type of a given variable in typescript. In TypeScript, as in JavaScript, typeof returns a string indicating the type of the operand. The possible return values are “string,” “number,” “bigint,” “boolean,” “symbol,” “undefined,” “function,” and “object.” Here’s an example of how to use typeof in TypeScript to check type in typescript.
let city: string = "Los Angeles";
console.log(typeof city); // Output: string
Here, you can see I ran the above code in Visual Studio code, and it returns the type as string. Check the screenshot below:
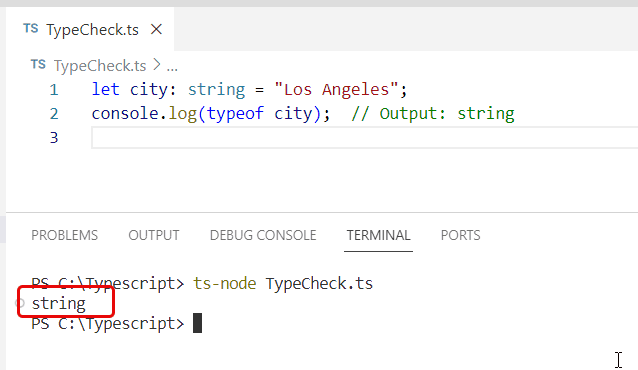
2. Check type in Typescript using instanceof Operator
Let us see how to check type in typescript using instanceof Operator.
The instanceof operator is used to test whether an object is an instance of a specific class or constructor function in Typescript. It returns a boolean value: true if the object is an instance of the specified class or constructor function and false otherwise.
Here’s an example of how to use instanceof in TypeScript:
class Car {
constructor(public make: string, public model: string) {}
}
let myCar = new Car("Ford", "F-150");
console.log(myCar instanceof Car); // Output: true
You can check the screenshot below, I ran the code using Visual Studio Code, and it return true.
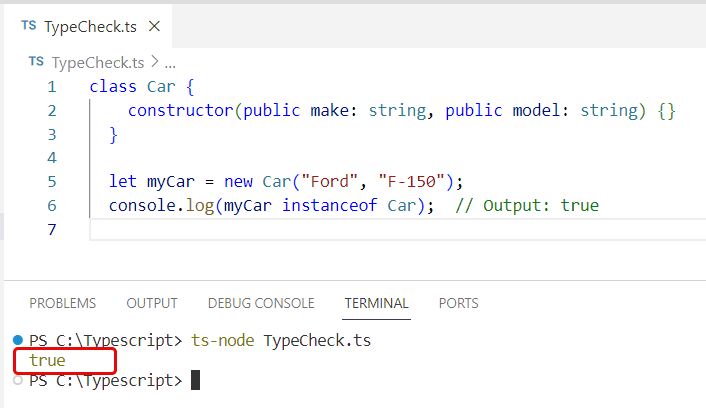
3. Typescript type check using Custom Type Guards
Now, let us check how to check typeof typescript using Custom Type Guards with an example.
Custom type guards are a powerful feature in TypeScript that allows you to create your own type-checking functions. Here’s an example:
function isString(value: any): value is string {
return typeof value === "string";
}
let state: any = "California";
console.log(isString(state)); // Output: true
Check the screenshot below, where I execute the custom function using Visual Studio Code and execute the program using the below command.
ts-node TypeCheck.ts
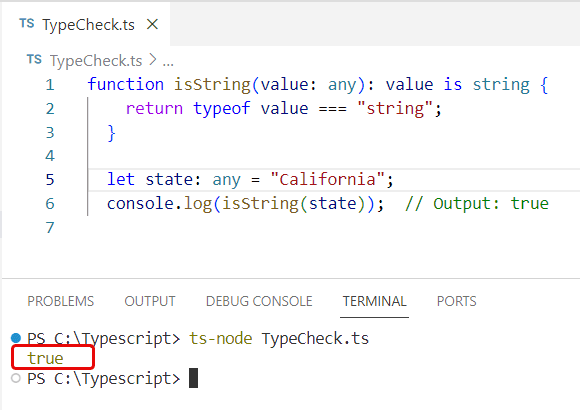
This is how to check type of variable in typescript using Custom Type Guards.
Typescript check type of variable: Examples
Here are a few examples of typescript type check.
Example-1: Check the type of a number in Typescript
Here is an example to check the type of a number in Typescript.
let population: number = 327000000;
console.log(typeof population); // Output: number
In this example, the typeof operator is used to check the type of the variable population. The output will be a number, confirming that the population is of type number.
Example-2: Check the type of a boolean in Typescript
Here is an example to check the type of boolean variable in Typescript. In this example, the typeof operator is used to check the type of the variable isRaining. The output will be boolean, confirming that isRaining is of type boolean.
let isRaining: boolean = false;
console.log(typeof isRaining); // Output: boolean
Example-3: Typescript Check the Type of an Array
Here is an example to check the type of an array variable in Typescript.
The Array.isArray method is used to check if the variable states is an array. The output will be true, confirming that states is of type array.
let states: string[] = ["California", "Texas", "Florida"];
console.log(Array.isArray(states)); // Output: true
Example-4: Check the Type of a Function in Typescript
Here is an example of a Typescript check type of a function.
The typeof operator in Typescript is used to check the type of the variable add. The output will be a function, confirming that add is of type function.
function add(a: number, b: number): number {
return a + b;
}
console.log(typeof add); // Output: function
Example-5: Check type typescript for an Object
Here is another example to check the type of an object in Typescript. The typeof operator is used to check the type of the variable person. The output will be an object, confirming that the person is of type object.
let person = {
name: "John Doe",
age: 30
};
console.log(typeof person); // Output: object
Example-6: Check type in Typescript for a Symbol
In this example, the Typescript typeof operator is used to check the type of the variable sym. The output will be a symbol, confirming that the sym is of a type symbol.
let sym = Symbol("unique");
console.log(typeof sym); // Output: symbol
Example-7: Check If a Value Is NaN in TypeScript
The isNaN() function can be used in TypeScript to check if a value is NaN (Not-a-Number). Here’s an example:
let temperature: any = "seventy degrees";
console.log(isNaN(temperature)); // Output: true
In this example, the variable temperature is assigned the value “seventy degrees”, which is not a valid number. The isNaN() function is then used to check if the temperature is NaN. The output will be true, confirming that temperature is not a number.
It’s important to note that isNaN() will first try to convert the value to a number before checking if it is NaN. If the value can be converted to a number, isNaN() will return false.
Conclusion
In this Typescript tutorial, I’ve shown you three different methods to check the type of variables in TypeScript: using the typeof operator, the instanceof operator, and custom type guards. I’ve also provided examples for checking the type of various variable types, including string, number, boolean, array, object, function, etc.
You may also like:
- How to Sort Array Alphabetically in Typescript?
- How to compare strings for sorting in typescript
- How to map an array of objects to another array of objects in Typescript
- Convert Typescript Array to String With Separator
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com