In this typescript tutorial, we will see how to check if the string contains only numbers in typescript.
To check if string contains only numbers in typescript by using different methods:
- Using the
isNaN()
function in typescript - Using a regular expression in typescript
- Using the
match()
function in typescript.
Using the isNaN()
function in typescript
Here we will see how to check if string contains only numbers by using the isNaN()
function in typescript in typescript.
The isNaN() function in TypeScript is a built-in function that determines whether or not a value is NaN (Not a Number). The isNaN() function accepts one input of any value. If the value is NaN, it returns true; otherwise, it returns false.
The parseInt() method is used to convert text to an integer. If the string solely contains numeric characters, parseInt() will return a legitimate integer; otherwise, it will return NaN.
So here we will take a string, we will use parseInt() to convert the string to an integer, then by using isNaN(), we will check whether it is a number or not. If it is NaN, it signifies that the string does not include just numeric characters, thus we use the ! operator to negate the result.
Open the code editor, create a file named as ‘containsNum.ts’, and write the below code:
const str = "23444";
const isNum = !isNaN(parseInt(str));
console.log(parseInt(str))//23444
console.log(isNum); // true
To compile the code, run the below command and you can see the result in the console:
ts-node containsNum.ts
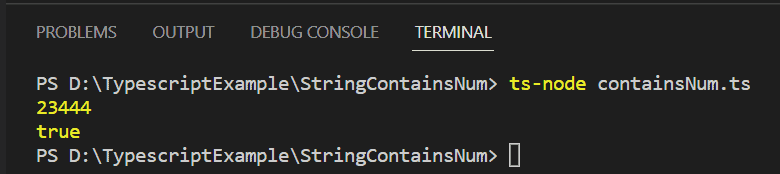
This is an example of how we can check if a string contains only numbers by using the isNaN()
function in typescript in typescript.
Using a regular expression in typescript
Here we will see how to check if a string contains only numbers by Using a regular expression in typescript.
The test() function is a TypeScript method available on a regular expression object. It is used to determine if a string matches a regular expression pattern or not. The test() method produces a boolean value: true if the string fits the regular expression pattern, false otherwise.
For example, we will define the regular expression ‘/[0-9]+$/ ‘ matches any text that exclusively contains numbers (0 to 9). The regular expression object’s test function is used to determine whether the string matches the pattern and returns a boolean result indicating whether the string includes only numeric characters or not.
In the containsNum.ts file, write the below code
const str = "23444";
const isNum = /^[0-9]+$/.test(str);
console.log(isNum); // true
To compile the code, run the below command and you can see the result in the console:
ts-node containsNum.ts
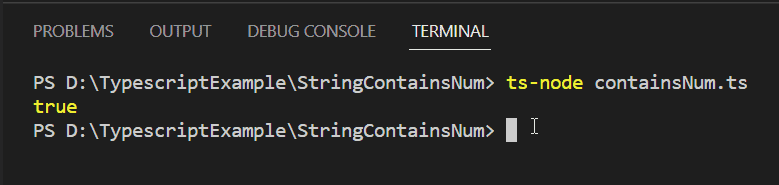
This is an example to check if string contains only numbers in typescript by using regular expression.
Using the match()
function in typescript
Here we will see how to check if string contains only numbers by Using the match()
function in typescript
For example, we will use the match() to match the string against a regular expression pattern that only allows numeric characters (0-9) in typescript.
The match() method produces an array of matches, or null if no matches were found. Here the string is then checked to determine if it includes just numeric characters if the result is not null.
In the containsNum.ts file, write the below code
const str = "23444";
const isNum = str.match(/^\d+$/) !== null;
console.log(isNum); // true
To compile the code, run the below command and you can see the result in the console:
ts-node containsNum.ts
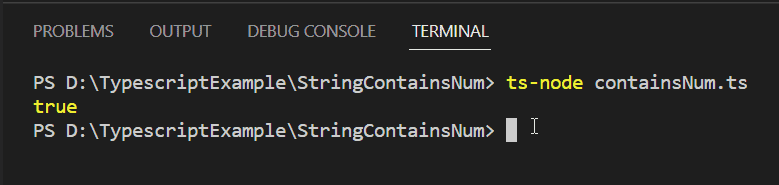
This is an example of how we can check if a string contains only numbers by Using the match()
function in typescript.
Conclusion
In this typescript tutorial, we saw how we can check if string contains only numbers or by using different methods. Here are the methods we used:
- Using the
isNaN()
function in typescript - Using a regular expression in typescript
- Using the
match()
function in typescript.
You may like the following typescript tutorials:
- Typescript convert string to keyof
- Typescript string capitalize first letter
- How to get string between 2 characters in Typescript
- Typescript string union vs enum
- Typescript split string by dot
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com