In this Microsoft Teams tutorial, we will see how to connect to Microsoft Teams using PowerShell. We will also see a few examples of Microsoft Teams with PowerShell.
Connect to Microsoft teams PowerShell
Are you wondering, How do I connect to a Microsoft team using PowerShell? It is simple and easy to install and connect to the Microsoft teams PowerShell module. We can install Microsoft Teams PowerShell module as it is supported in all Windows platforms.
Prerequisites:
You need to have PowerShell 5.1 or higher version and .NET Framework 4.7.2. Download latest version of PowerShell.
Install Teams PowerShell Module:
Run the below PowerShell cmdlet to install Microsoft Teams PowerShell module. I am using here PowerShell ISE.
Install-Module -Name MicrosoftTeams -Force
It will take sometime and install like below:
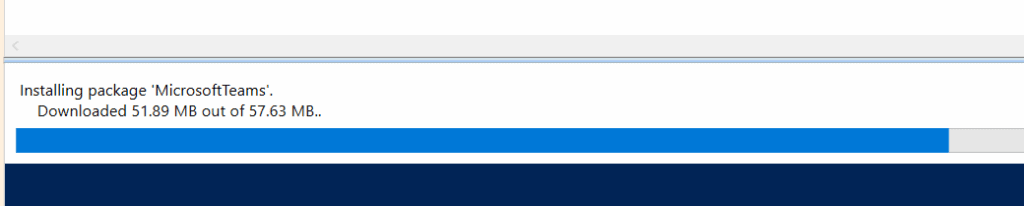
If you install all a particular version, then run the following command.
Install-Module -Name MicrosoftTeams -RequiredVersion 2.3.1
Connect to Microsoft teams PowerShell
Once the installation is successful, we will see how to connect to microsoft teams using PowerShell.
For this Microsoft provides Connect-MicrosoftTeams cmdlets.
$credential = Get-Credential
Connect-MicrosoftTeams -Credential $credential
When you run the above commands, it will ask you to enter the credentials. Make sure to put the admin credentials for Microsoft Teams.
Then it will connect.
This is how to connect to a Microsoft team using PowerShell using the Connect-MicrosoftTeams PowerShell cmdlets.
Update PowerShell Teams Module
If you want to update Teams PowerShell module run the below PowerShell cmdlets.
Update-Module MicrosoftTeams
Uninstall PowerShell Teams Module
If you uninstall Microsoft Teams PowerShell module, then run the following PowerShell cmdlets.
Uninstall-Module MicrosoftTeams
If you want to uninstall Teams PowerShell module then run the following cmdlets.
Uninstall-Module MicrosoftTeams -Allversions
Disconnect Microsoft teams PowerShell
After executing the PowerShell Teams commands, we should disconnect the connection.
If you want to disconnect Microsoft teams in PowerShell, then run the below command:
Disconnect-MicrosoftTeams
This is how to install, uninstall and update Microsoft Teams PowerShell module.
Read: How to create a team in Microsoft Teams
Teams PowerShell commands examples
Let us see a few examples of teams PowerShell commands and examples.
1. Create teams in Microsoft teams using PowerShell
Let us see a Microsoft teams PowerShell example.
Below is the PowerShell command to create a team in Microsoft Teams using PowerShell. We can use the New-Team PowerShell cmdlets.
New-Team -DisplayName "Weekend Trip" -Description "This is team for the trip" -Visibility Public
This will create a public team, where anyone from your organization can join. If you want to create a private team, set the Visibility to Private.
The below PowerShell command will create a new team in Microsoft teams and then will add a user to the team and then it will also create a channel in the team.
$group = New-Team -MailNickname "HRTeam" -displayname "HR Team" -Visibility Public
Add-TeamUser -GroupId $group.GroupId -User "user1@tsinfotechnologies.onmicrosoft.com"
New-TeamChannel -GroupId $group.GroupId -DisplayName "HR USA"
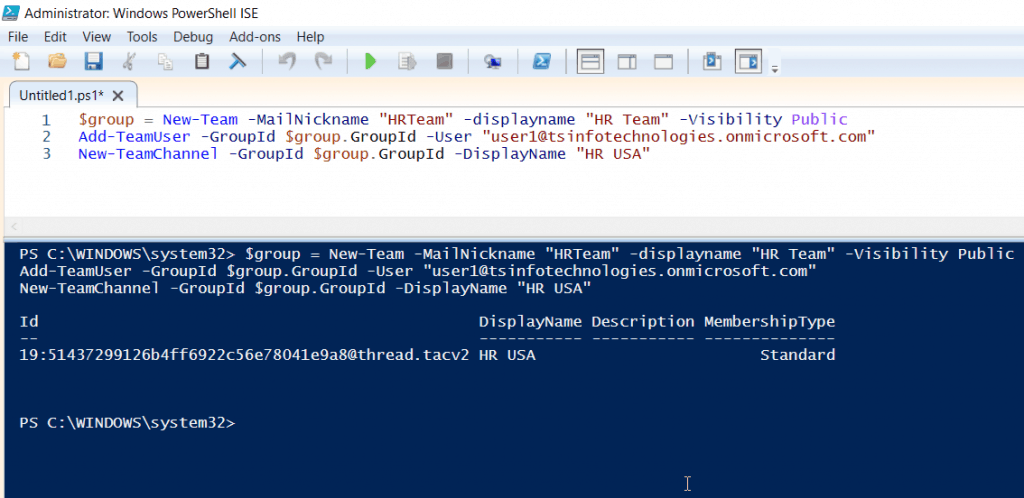
Once the Teams PowerShell command executed successfully, you will see the team got created with the member and channel in Microsoft Teams.
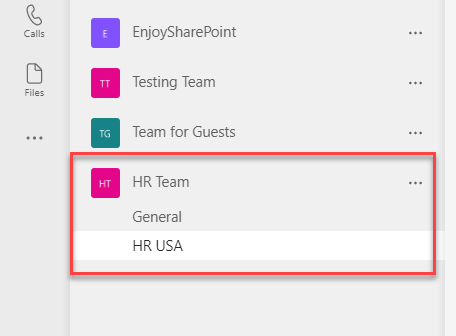
2. Get GroupId of Team in Microsoft Teams using PowerShell
To do any PowerShell operation in a Team in Microsoft Teams, we need to have the GroupId. Below is the PowerShell command we can use to get group id from Teams Display name.
The below command will return then Group id, Display name, visibility, Description etc.
Get-Team -DisplayName "TestingTeam"
We can also run the below command.
$team=Get-Team -DisplayName "TestingTeam"
$team.GroupId
3. PowerShell add user to team
Now, let us see, how to add user to a team using PowerShell in Microsoft Teams.
Microsoft provides Add-TeamUser PowerShell command that we can use to add an owner or a member to a Microsoft team.
Add-TeamUser -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -User user1@tsinfotechnologies.onmicrosoft.com
Once you execute the command, you can see the user will be added to the Team.
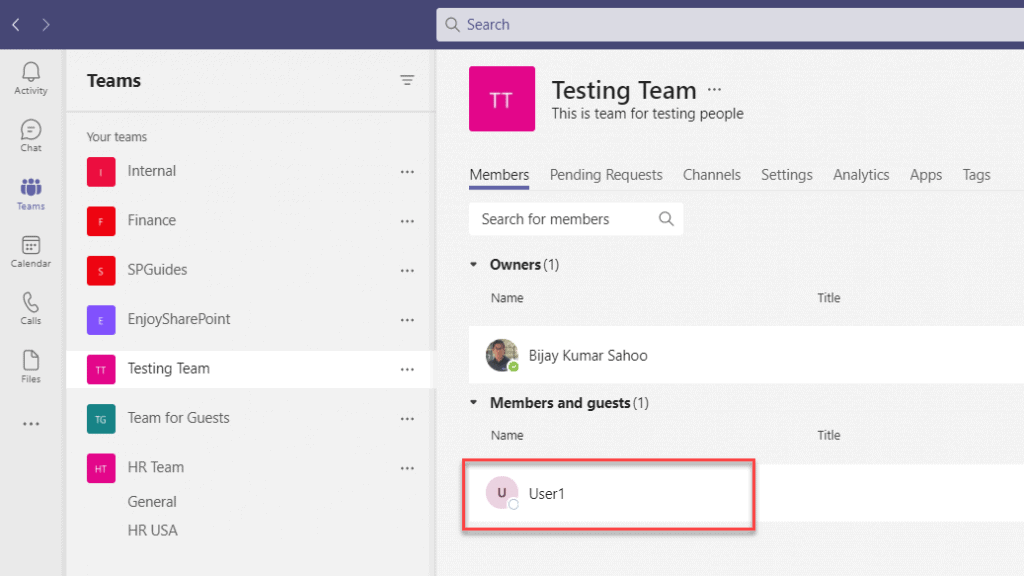
If you want to add a user as the owner of the team in Microsoft Teams using PowerShell, then add the -Role parameter and the value should be Owner. Below is the example.
Add-TeamUser -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -User Bhawana@tsinfotechnologies.onmicrosoft.com -Role Owner
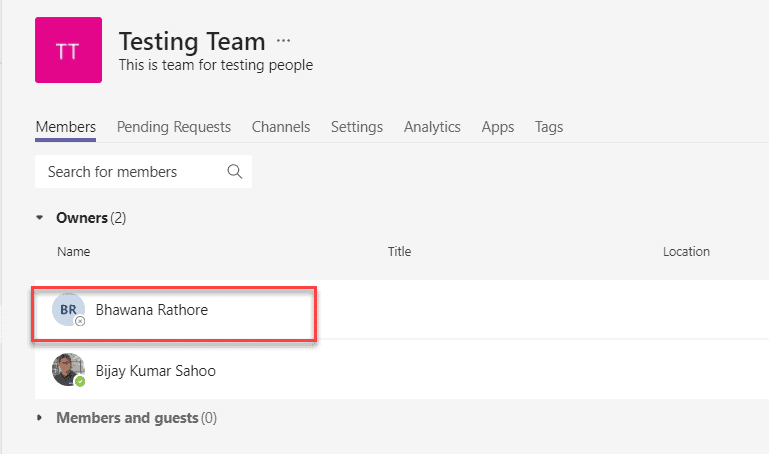
4. PowerShell get-team
Let us see how to get a list of all Microsoft Teams PowerShell.
Below is the PowerShell cmdlets that will gives us all the teams from Microsoft Teams.
Get-Team
It will return the below properties:
- GroupId
- DisplayName
- Visibility
- Archived
- MailNickName
- Description
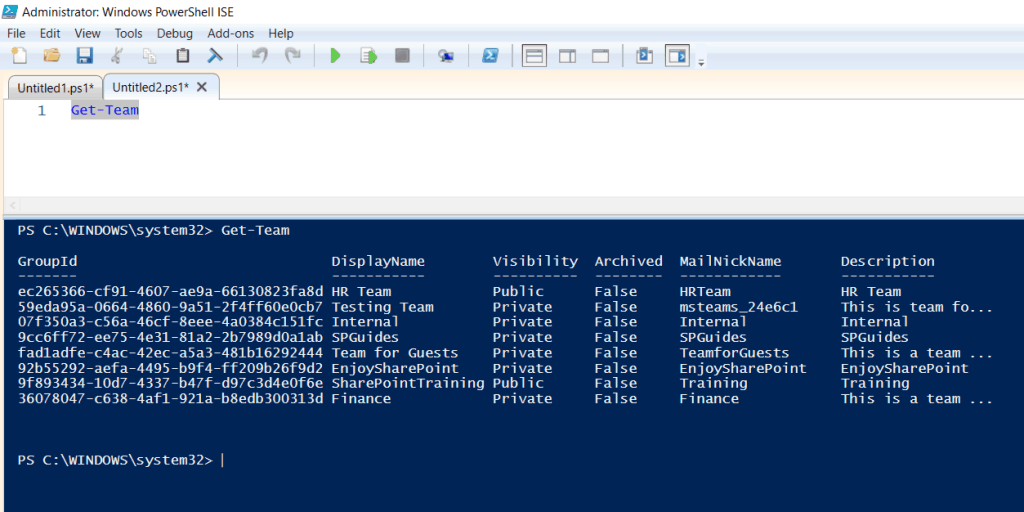
Similarly, if you want to get team details based on the Display Name then run the below command:
Get-Team -DisplayName "Testing Team"
If you want to display the team details based on the MailNickName then run the below PowerShell commands.
Get-Team -MailNickName "TestingTeam"
5. Get all teams a user is a member of
Let us see, how to get all teams a user is a member of using PowerShell. Below is the PowerShell cmdlet:
Get-Team -User user1@tsinfotechnologies.onmicrosoft.com
This will give all the teams the user belongs to in Microsoft Teams using PowerShell.
6. Create channel in teams PowerShell
Let us see another Microsoft teams PowerShell example, how to create a channel in Microsoft Teams using PowerShell.
Microsoft provides New-TeamChannel PowerShell cmdlets to create a channel for a particular team in Microsoft Teams.
Here we need to get the groupid. Follow the above example of get the groupid of a particular team.
New-TeamChannel -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -DisplayName "USA Testing Team"
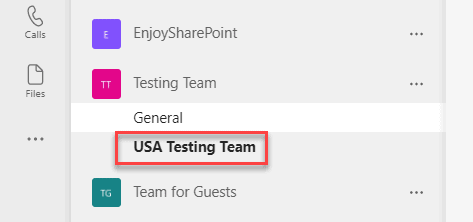
If you want to create a private channel, then pass parameter as, -MembershipType Private, it looks like below:
New-TeamChannel -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -DisplayName "USA Testing Team" -MembershipType Private
This is how to add a channel to a team using PowerShell.
7. PowerShell get all teams users
Let us see, how to get all teams users in PowerShell.
Microsoft provides Get-TeamUser PowerShell cmdlets to get users of a particular team in Microsoft Teams.
Below is the PowerShell command that will give all the users of a particular Microsoft team. It includes both owners and members.
Get-TeamUser -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7
If you want to retrieve only the owners, then run the below command (Pass the -Role Owner as a parameter):
Get-TeamUser -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -Role Owner
If you want to retrieve only the members, then run the below command (Pass the -Role Member as a parameter):
Get-TeamUser -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -Role Member
If you want to retrieve only the Guests, then run the below command (Pass the -Role Guest as a parameter):
Get-TeamUser -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -Role Guest
You can see the output.
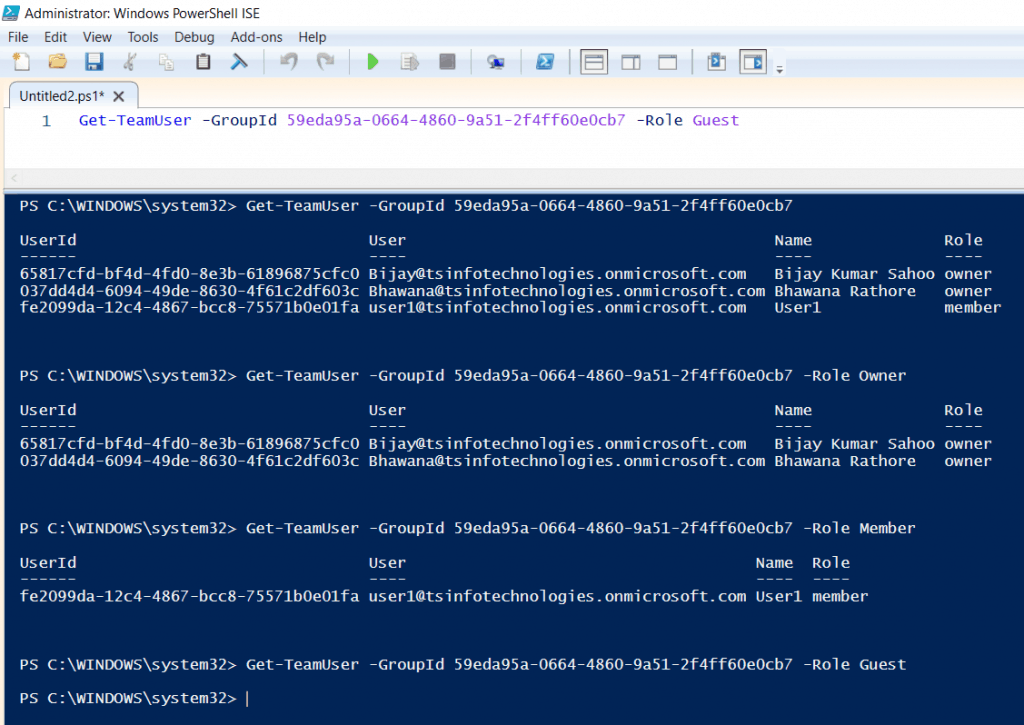
8. PowerShell get all teams and owners
Here is another interesting example on Microsoft teams PowerShell. Here we will se, how to get all teams and corresponding owners using PowerShell in Microsoft Teams.
A team can have more than one owners, so run the below PowerShell script that will give you all the teams and corresponding team owners in proper format.
$allTeams=Get-Team
# Loop through the teams
foreach($team in $allTeams)
{
$allOwners= Get-TeamUser -GroupId $team.GroupId -Role Owner
"`n" + "Team Name: " + $team.DisplayName
foreach($owner in $allOwners)
{
Write-Host "User: " $owner.User " Name: " $owner.Name
}
}
You can see the output.
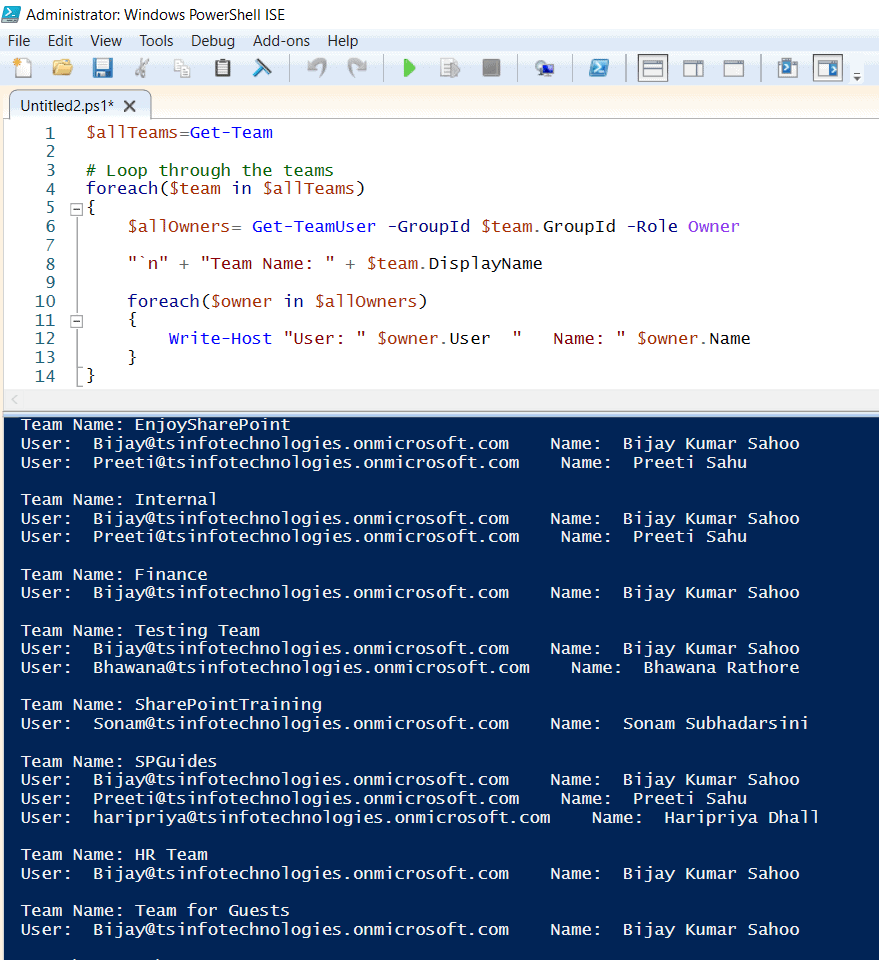
This is how to get all the teams and owners in Microsoft Teams using PowerShell.
9. Get all teams and channels using PowerShell
How to get all teams and channels using PowerShell in teams? To get all the teams and corresponding channels using PowerShell in Microsoft Teams we can use the Get-TeamChannel PowerShell cmdlets.
A team can have one or more than one channel in Microsoft Teams. In the below code, we first retrieve all the teams and then apply foreach loop to get all the channels for a particular Team using PowerShell.
$allTeams=Get-Team
foreach($team in $allTeams)
{
$allChannel = Get-TeamChannel -groupid $team.groupid
"`n" + "Team Name: " + $team.DisplayName
foreach($channel in $allChannel)
{
Write-Host "Channel Name: " $channel.DisplayName
}
}
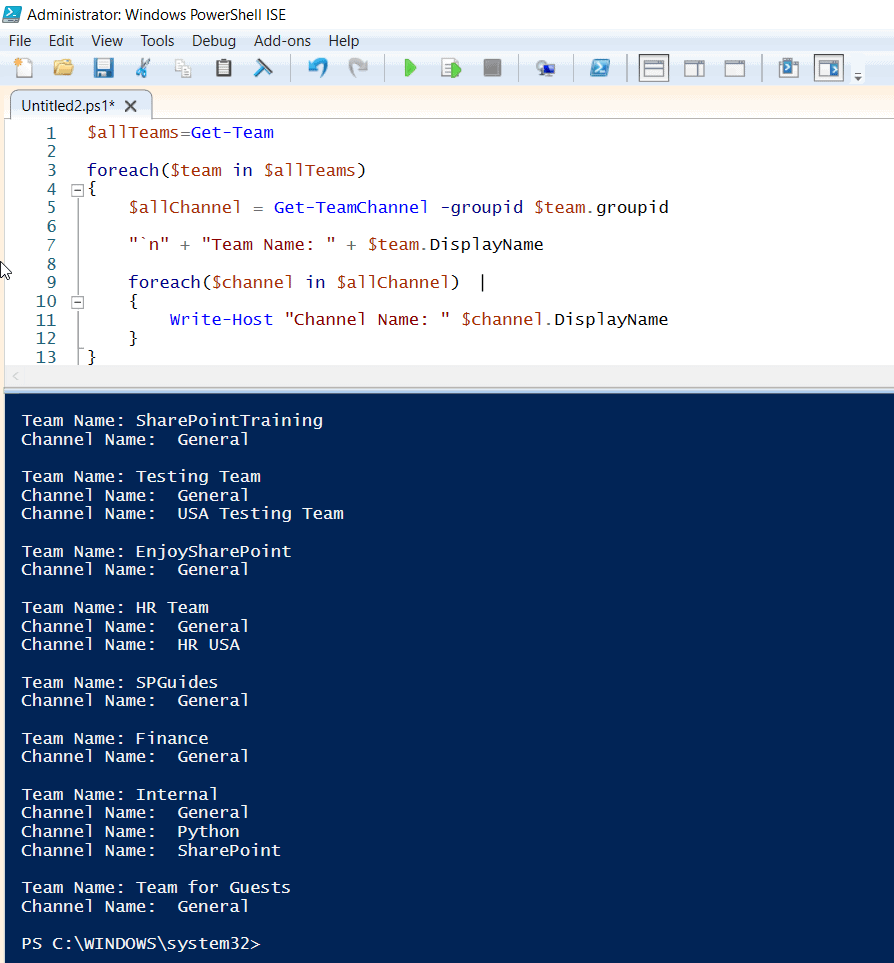
If you want to get the channels from a particular team, then run the following PowerShell command.
Get-TeamChannel -groupid 59eda95a-0664-4860-9a51-2f4ff60e0cb7
The above PowerShell cmdlet will return all the channels for a particular team in Microsoft Teams.
10. PowerShell add channel to team from CSV file
Now, let us see how to add channels to a team from a CSV file using PowerShell in Microsoft Teams. Here I have a CSV file that has one column as Name and contains a few records.
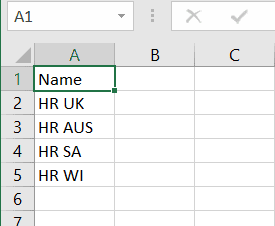
Below is the PowerShell cmdlet to add bulk channels to a Microsoft Team using PowerShell.
Import-csv D:\Bijay\Channels.csv | foreach{New-TeamChannel -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -DisplayName $_.Name}
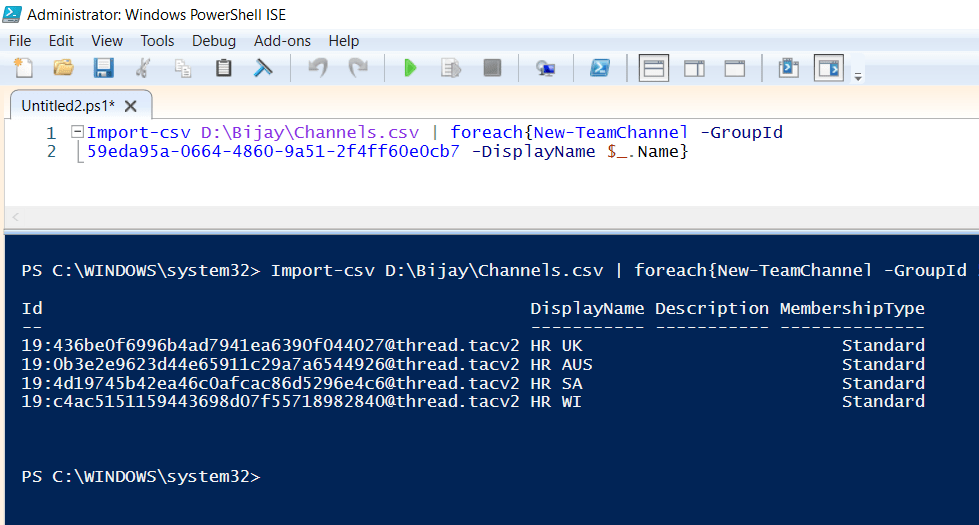
This way, we can add bulk channel to a Microsoft Team from a CSV file using PowerShell.
Also, check out, Microsoft Teams Tips and Tricks
11. Create teams PowerShell csv
Let us see now how to create teams using PowerShell from CSV file. The below PowerShell script will help you to create teams in bulk.
Here I have a .csv file having some Team names.
Import-csv D:\Bijay\Teams.csv | foreach{New-Team -DisplayName $_.Name}
You can see it created like below:
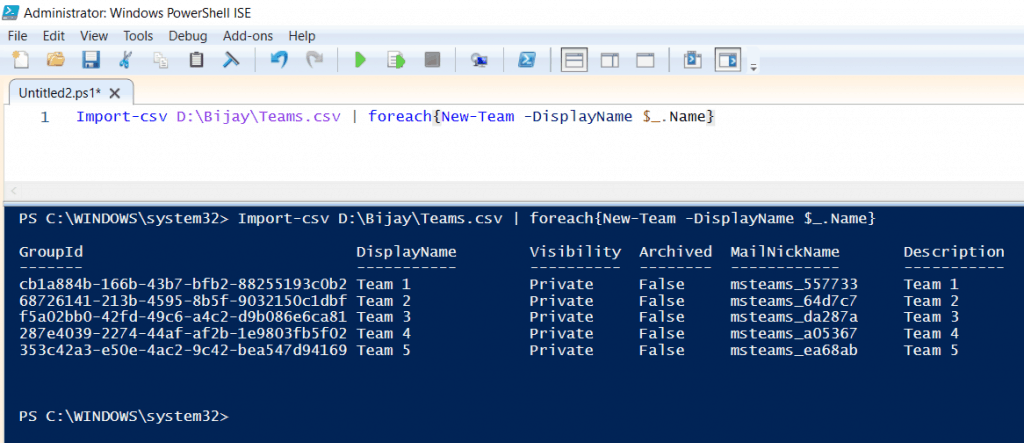
If you open Microsoft Teams desktop app, you will be able to see all the teams created successfully from the .CSV file.
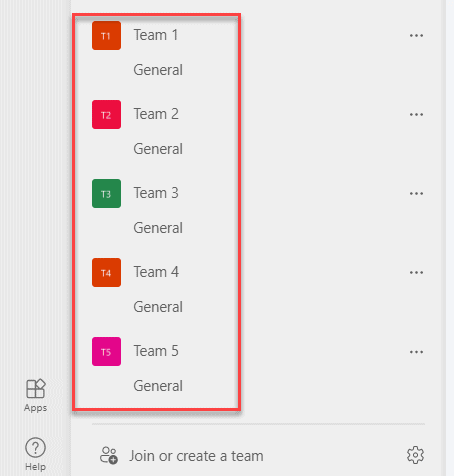
This is how to create teams in bulk from a csv file using PowerShell.
12. Delete Teams using PowerShell
Now, let us see how to delete a team using PowerShell.
Microsoft Teams provides Remove-Team PowerShell cmdlets to delete a particular team using PowerShell.
Remove-Team -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7
If you want to remove teams in bulk using PowerShell, then follow the below PowerShell script. Here I have used the same .csv file.
Import-csv D:\Bijay\Teams.csv | foreach{
$team=Get-Team -DisplayName $_.Name
Remove-Team -GroupId $team.GroupId
}
It will delete all teams that are presented in the .csv file.
13. PowerShell add guest user to teams
Now, let us see how to add guest users to teams using PowerShell. Run the below PowerShell command to add a guest user to a team in Microsoft Teams.
Add-TeamUser -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -User yourguestuseremailid@gmail.com -Role Member
Here if the guest user is not added to the Azure active directory then you will get an error like below:
Add-TeamUser : Error occurred while executing
Code: Request_ResourceNotFound
Message: Resource ‘fewlines4biju@gmail.com’ does not exist or one of its queried reference-property objects are not present.
InnerError:
RequestId: 36aed36d-d7de-43c5-b0ff-aee9111e7a24
DateTimeStamp: 2021-06-23T12:55:58
HttpStatusCode: Request_ResourceNotFound
At line:1 char:1
- Add-TeamUser -GroupId 59eda95a-0664-4860-9a51-2f4ff60e0cb7 -User fewl …
~~~~~~~~~~~~~~~~~- CategoryInfo : NotSpecified: (:) [Add-TeamUser], ApiException
- FullyQualifiedErrorId : Microsoft.TeamsCmdlets.PowerShell.Custom.ErrorHandling.ApiException,Microsoft.TeamsCmdlets.PowerShell.Custom.AddT
eamUser
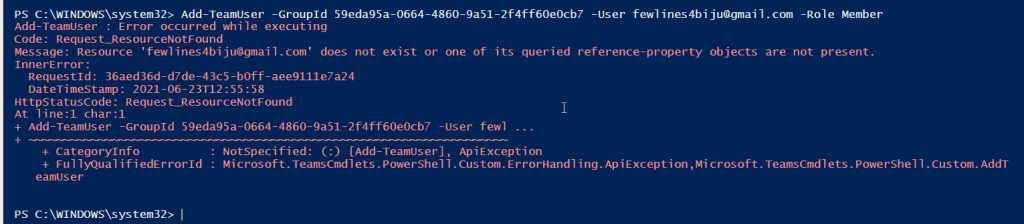
To fix this issue, check if the user is there in Guest users list in Microsoft 365 admin center.
Open Microsoft 365 admin center, Expand Users -> Guest users and the user should be there in the Guest users list.
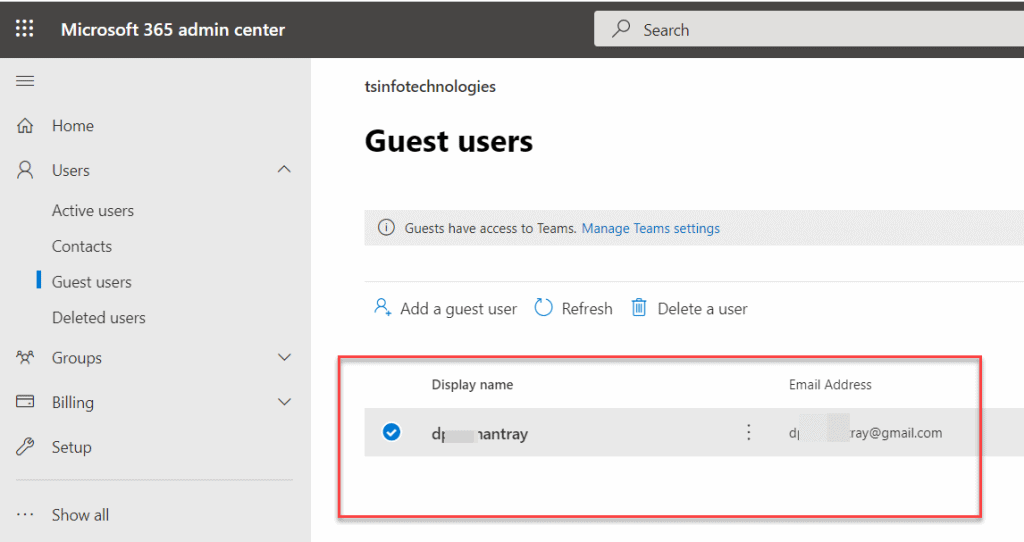
If the user is not presented here, then the error will come. And if the user is there then error will not come and the guest user will be added to teams.
If you want to add bulk guest users in Azure AD then follow this article.
This is how to add guest user to teams using PowerShell.
You may like the following tutorials:
- How to add PowerApps app to Microsoft Teams – Step by Step
- How to invite guest or external users to Microsoft Teams Meetings
- Microsoft teams settings
- How to create a group in Microsoft teams (ms teams Group Call and Group Chat)
- How to leave an organization as a guest user in Microsoft Teams
In this tutorial, we learned how to connect to Microsoft Teams using PowerShell, how to update, uninstall PowerShell teams module, and the below examples:
- Create teams in Microsoft teams using PowerShell
- Get GroupId of Team in Microsoft Teams using PowerShell
- PowerShell add user to team
- PowerShell get-team
- Get all teams a user is a member of
- Create channel in teams PowerShell
- PowerShell get all teams users
- PowerShell get all teams and owners
- Get all teams and channels using PowerShell
- PowerShell add channel to team from CSV file
- Create teams PowerShell csv
- Delete Teams using PowerShell
- PowerShell add guest user to teams
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com