In this typescript tutorial, we will see how to create a multiline string in typescript or how to define a multiline string in Typescript.
In Typescript, there are multiple ways to define a multiline string. These different ways are listed below.
- Using template literals
- Using escape sequence (\n)
- Using plus operator
- Using backslash
- Using an array and join()
- Using escape sequence (\r\n)
Typescript Multiline String using template literals
Here we will see how to define multiline strings using template literals in typescript
In typescript, we will define multiline strings using template literals. A template literal is nothing but a string that is defined with backticks (“), instead of double and single quotes.
Here you can see the example to define multiline string using templates literals. Write the below code in the multilineString.ts file.
// Defining a multipine string
let info: string = `New York commonly known as New York City.
Also refered as NYC.
It is the most populous city in the United States.`
// Printing result
console.log(info)
To compile the code and run the below command and you can see the result in the console.
ts-node multilineString.ts
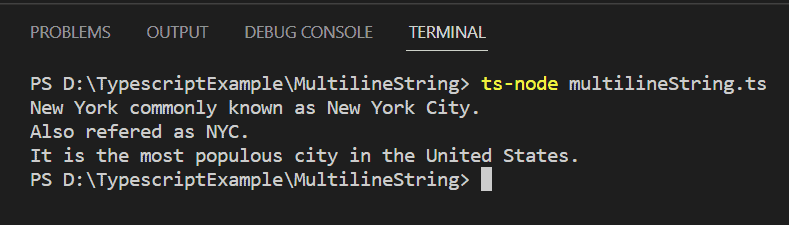
This is an example of a Typescript multiline string using template literals.
Typescript Multiline String using \n
Here we will see how we can define a multiline string in typescript using escape sequence(\n).
The \n is represented as the escape sequence which is used for a line break. Let’s see how we can implement the \n in our program to define a multiline string in the typescript.
Open the code editor, and create a file named multilineString.ts file, write the below code:
// Defining a multipine string
let info: string = "New York commonly known as New York City.\nAlso refered as NYC.\nIt is the most populous city in the United States."
// Printing result
console.log(info)
To compile the code and run the below command and you can see the result in the console.
ts-node multilineString.ts
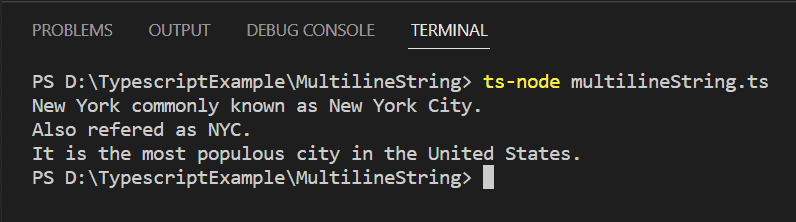
Note:
The best way to define multipleline string is by using template literals in typescript.
This is an example to define multiple line string in typescript, using \n.
Check out, How to check if string contains only numbers in typescript
Typescript Multiline String using the plus operator
Here we will how we can define multiline strings using the plus operator in typescript.
We will use the plus operator ‘+’ to combine the string and, the escape sequence to define a multiline string in typescript.
In the multilineString.ts file write the below code:
// Defining a multipine string
let info: string = "New York commonly known as New York City."
+ "\nAlso refered as NYC.\n"
+ "It is the most populous city in the United States."
// Printing result
console.log(info)
To compile the code and run the below command and you can see the result in the console:
ts-node multilineString.ts
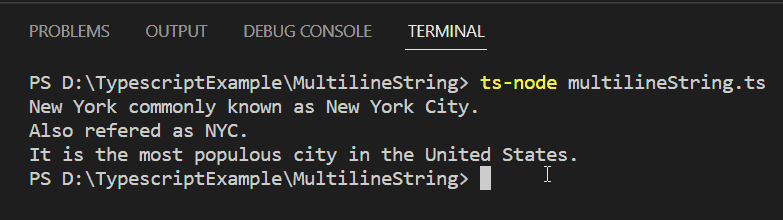
This is an example of a typescript Multiline String using the plus operator.
Typescript Multiline String using backslash
Here we will see how we can use multiline string using a backslash in typescript.
We will use the backslash(\) in the string to define the multiline string in typescript. Here backslash at the end of each line represents that the string continues to the next line.
In the multilineString.ts file, write the below code:
// Defining a multipine string
let info: string = 'New York commonly known as New York City. \
Also refered as NYC. \
It is the most populous city in the United States.'
// Printing result
console.log(info) // 👉️New York commonly known as New York City. Also refered as NYC. It is the most populous city in the United States.
To compile the code, run the below command and you can see the result in the console.
ts-node multilineString.ts
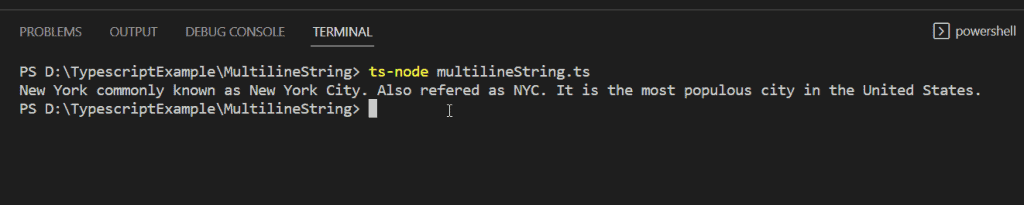
This is an example of a Typescript Multiline String using a backslash.
Typescript Multiline String using array & join()
Here we will see an example of a typescript multiline string using array and join().
For example, strings are used to represent the individual lines of the multiline string. The join()
method is used to concatenate the elements of the array into a single string, with the \n
escape sequence used as the separator. This approach can be useful if you need to dynamically build a string from multiple separate parts.
In the multilineString.ts file, write the below code:
// Defining a multipine string
let stringArray: string[] = [
'New York commonly known as New York City.',
'Also refered as NYC.',
'It is the most populous city in the United States.'
]
// Uisng join() to join array elements
let info: string = stringArray.join("\n")
// Printing result
console.log(info)
To compile the code and run the below command and you can see the result in the console:
ts-node multilineString.ts
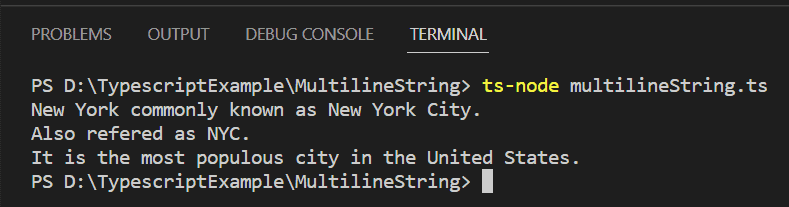
This is an example of a Typescript Multiline String using array & join().
Typescript Multiline String using \r\n
Here we will see an example of a typescript multiline string using \r\n.
For example, we will use the line break sequence character (\r\n) to define a multiline string in typescript.
In the multilineString.ts file, write the below code:
// Defining a multipine string
let info: string = "New York commonly known as New York City.\r\nAlso refered as NYC.\r\nIt is the most populous city in the United States."
// Printing result
console.log(info)
To compile the code and run the below command and you can see the result in the console.
ts-node multilineString.ts
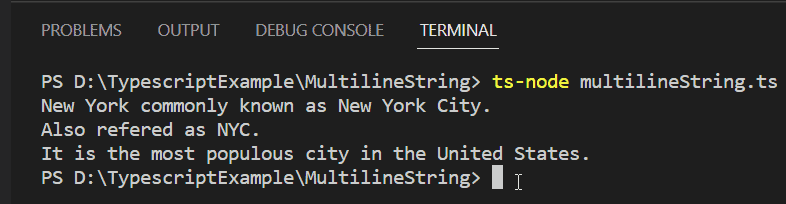
This is an example of a Typescript Multiline String using a line break sequence characters.
Conclusion
In this typescript tutorial, we saw how to define a multiline string in typescript by using different methods.
- Using plus operator
- Using template literals
- Using backslash
- Using an array and join()
- Using escape sequence (\n)
- Using escape sequence (\r\n)
You may like the following typescript tutorials:
- How to split a string with multiple separators in TypeScript?
- Typescript check if a string contains a substring
- Compare two string dates in typescript
- How to compare two strings in typescript
- Typescript string replaces all occurrences
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com