To become an SPFx developer, you should understand SPFx field customizers. I will explain here what a field customizer in the SharePoint framework is and how to create and deploy a spfx field customizer into SharePoint Online.
What is a Field Customizer Extension in the SharePoint Framework (SPFx)?
A Field Customizer Extension in SharePoint Framework (SPFx) lets you customize how columns appear and behave in SharePoint lists or libraries. You can adjust how data appears or add interactive features to improve the user experience without changing the actual data.
Here, I have a SharePoint Online list with a column named “PerformanceScore.” I have added an SPFx field customizer to change the way the field looks below.
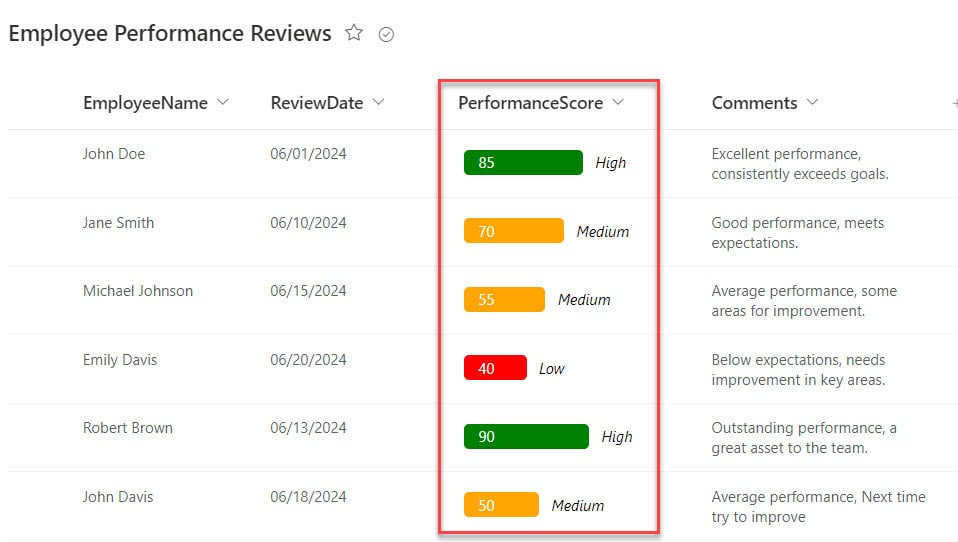
Now, let us do an example of a field customizer in SPFx.
Create SPFx Field Customizer Extension
Now, we will see how to create a field customizer extension in SPFx.
Check out this step-by-step tutorial if you have not yet set up the development environment.
Here, we see how we can achieve the above example.
Before starting the SPFx field customizer extension, you must have the same SharePoint list as the one shown below. If you have a different list, you must make some small changes to the code.
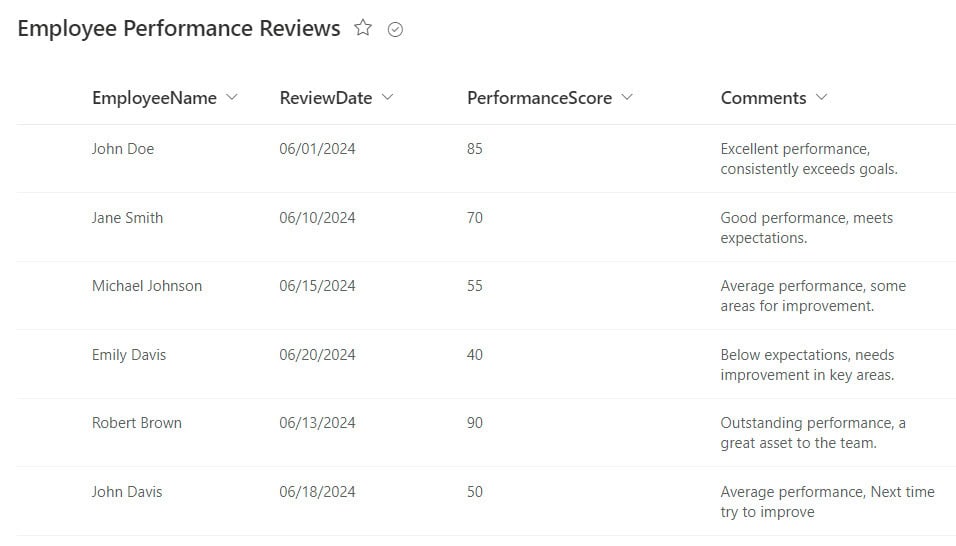
Open the Node.js command prompt and create a directory using the following command:
md PerformanceScoreCustomizer
Navigate to that directory using the following command:
cd PerformanceScoreCustomizer

To create an Extensions, we need to run Yeoman SharePoint Generator using the following command:
yo @microsoft/sharepoint
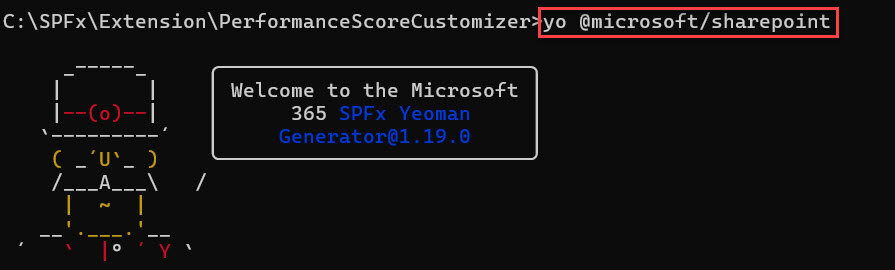
The Yeoman generator will guide you through a series of questions about the solution you want to create. Like below:
- What is the name of your solution? You can enter your solution name here. For example, I am naming mine ‘PerformanceScoreCustomizer.’
- Which type of client-side component to create? Choose Extension.
- Which type of client-side extension to create? Choose Field Customizer.
- What is your Application Customizer name? You can enter your Application name. For example, I am naming mine ‘PerformanceScore.’
- Which template would you like to use? Choose No framework

After creating the project, it will take some time to complete. Once finished, you should see a success message like this:
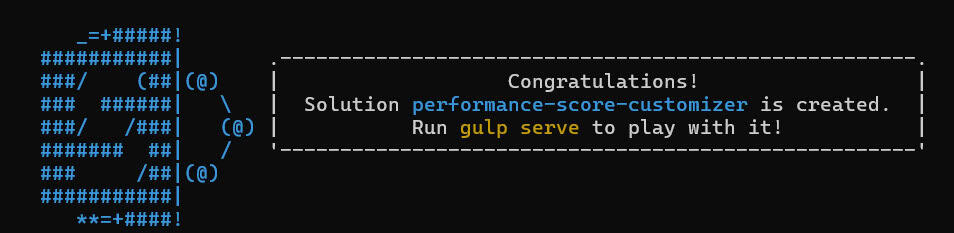
Next, you need to open this folder in VS code using the below command:
Code .
Then, the VS Code automatically opens with these files.
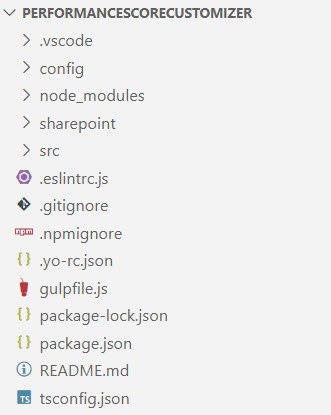
Now, in the Visual Studio Code, go to the src/extensions/PerformanceScoreFieldCustomizer.ts file. Here, we need to write our code.
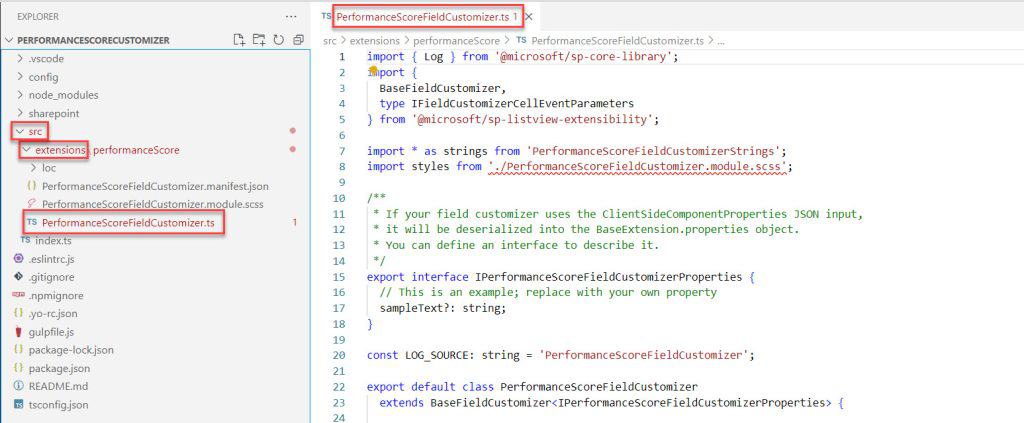
Now follow the below steps:
1. First, we import the necessary modules and classes for building SharePoint Framework (SPFx) extensions that are not automatically included by the Yeoman generator when creating a Field Customizer Extension.
import { Log } from '@microsoft/sp-core-library';
import {
BaseFieldCustomizer,
type IFieldCustomizerCellEventParameters
} from '@microsoft/sp-listview-extensibility';
import * as strings from 'PerformanceScoreFieldCustomizerStrings';
import styles from './PerformanceScoreFieldCustomizer.module.scss';
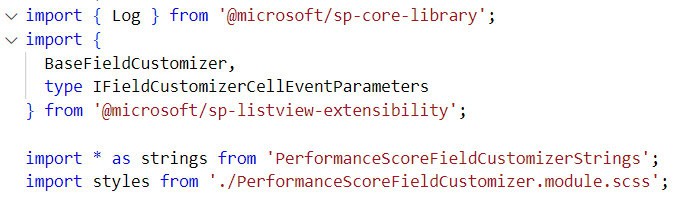
2. Then, we need to change some code in the onRenderCell method to customize the rendering of each cell in the list view. It calculates the performance score from event.fieldValue, determines the color and review text based on the score, applies styles using CSS modules (styles), and injects HTML into the DOM element (event.domElement).
Like the below code:
public onRenderCell(event: IFieldCustomizerCellEventParameters): void {
const performanceScore: number = parseInt(event.fieldValue);
let colorClass: string = '';
let reviewText: string = '';
if (performanceScore >= 80) {
colorClass = styles.highPerformance;
reviewText = 'High';
} else if (performanceScore >= 50) {
colorClass = styles.mediumPerformance;
reviewText = 'Medium';
} else {
colorClass = styles.lowPerformance;
reviewText = 'Low';
}
event.domElement.classList.add(styles.cell);
event.domElement.innerHTML = `
<div class='${styles.helloWorld}'>
<div class='${styles.full}'>
<div style='width: ${performanceScore}px;' class='${colorClass}'>
${performanceScore}
</div>
<div class='${styles.review}'>
${reviewText}
</div>
</div>
</div>`;
}
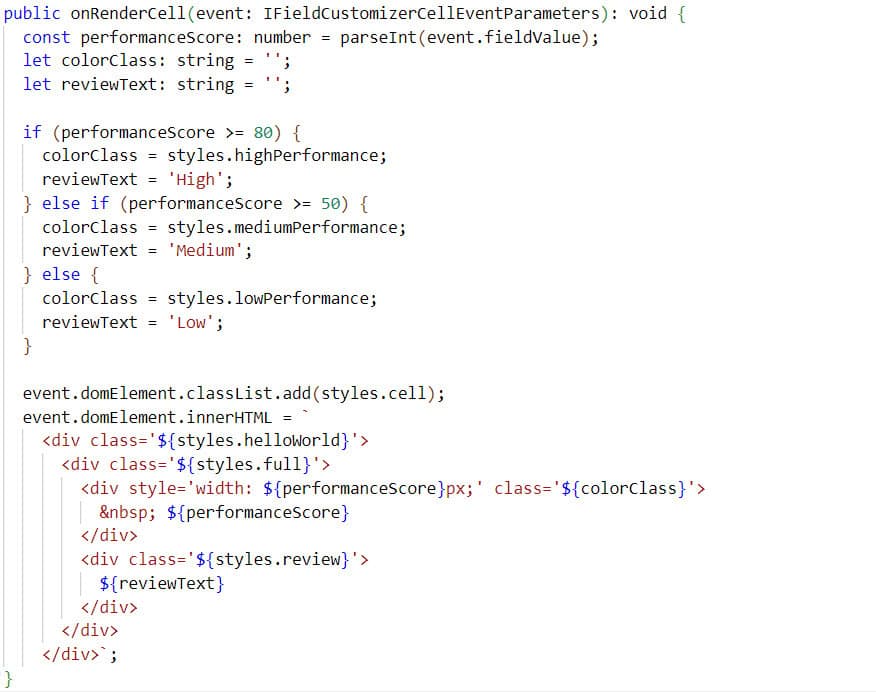
3. After that, I add CSS properties to the PerformanceScoreFieldCustomizer.module.scss file like below:
.cell {
padding: 5px;
}
.helloWorld {
display: flex;
align-items: center;
justify-content: space-between;
}
.full {
display: flex;
align-items: center;
width: 100%;
}
.highPerformance {
background-color: green;
color: white;
padding: 2px 5px;
border-radius: 4px;
}
.mediumPerformance {
background-color: orange;
color: white;
padding: 2px 5px;
border-radius: 4px;
}
.lowPerformance {
background-color: red;
color: white;
padding: 2px 5px;
border-radius: 4px;
}
.review {
margin-left: 10px;
font-style: italic;
color: rgb(0, 0, 0);
}
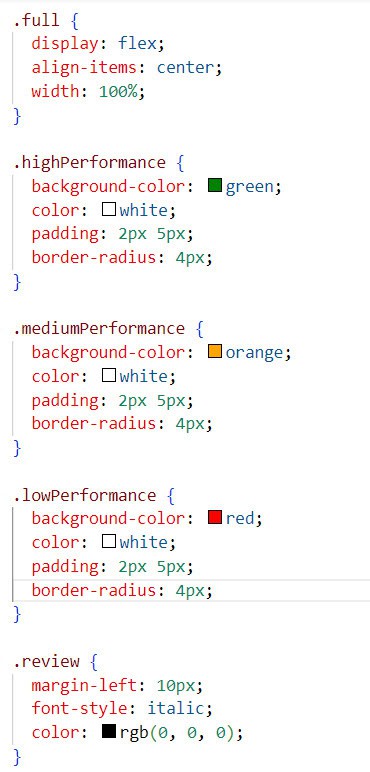
4. We cannot use the local workbench to test SharePoint framework extensions such as Field Customizers. Instead, we need to test directly on the SharePoint Online list.
To do this, open the serve.json file in the config folder. In the page URL field, enter your SharePoint online list URL.
Also, we need to provide the column’s internal name. I am using the PerformanceScore column here, as shown in the screenshot below.
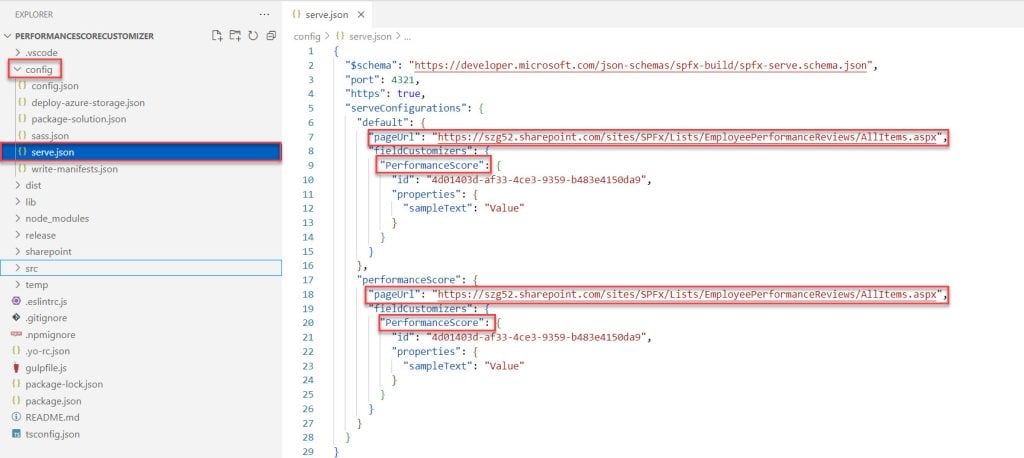
5. You can also modify the SharePoint/ assets/elements.xml file to create the column as a site column.
Here are two things to remember: DisplayName and Group. These will help you when you try to add a site column.
<?xml version="1.0" encoding="utf-8"?>
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<Field ID="{968aedcf-342e-4bad-a1f9-25243598fbe7}"
Name="SPFxPercentage"
DisplayName="PerformanceScore"
Type="Number"
Min="0"
Required="FALSE"
Group="SPFx Columns"
ClientSideComponentId="4d01403d-af33-4ce3-9359-b483e4150da9">
</Field>
</Elements>
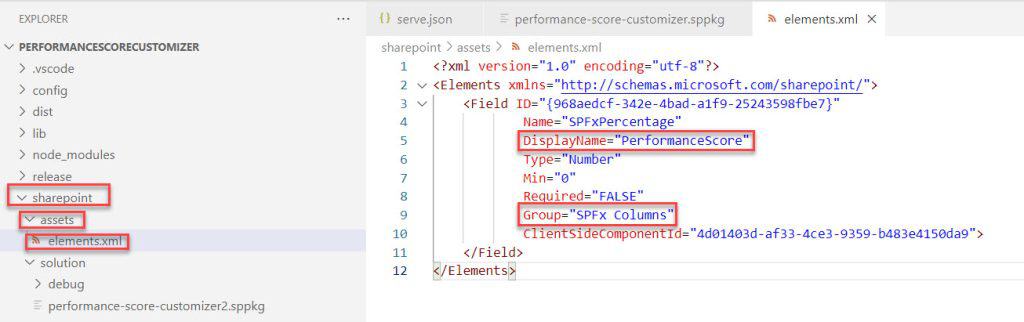
6. Then, in the VS Code, open a new terminal and run the below command:
gulp serve
7. It will automatically open the SharePoint page directly in the browser. That page shows a warning message here; you click on Load debug scripts like the below:
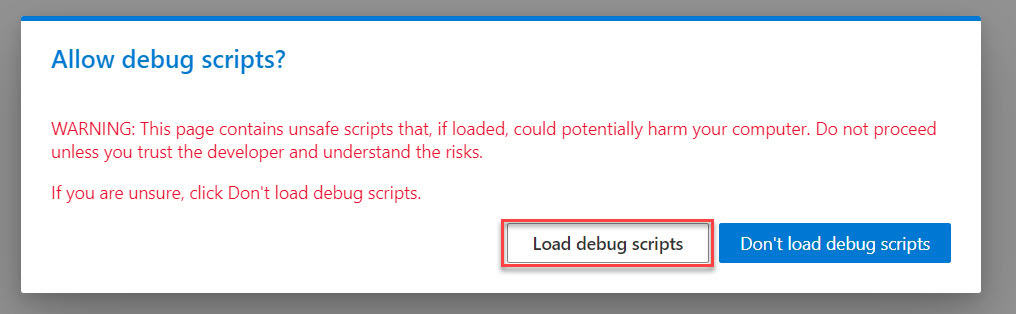
8. Now, it will display our SharePoint list column, like the screenshot below.
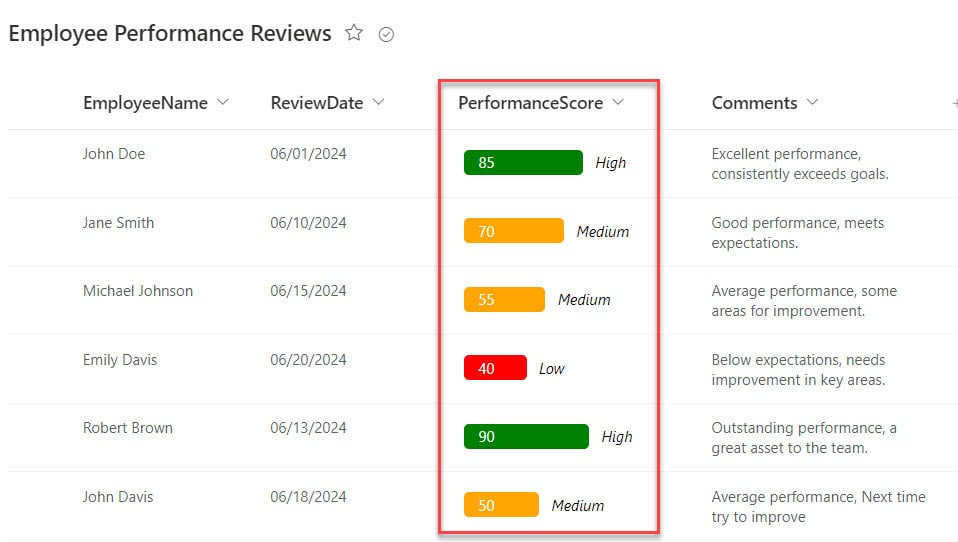
Deploy the SPFx Field Customizer Extension to an Existing SharePoint List
Now, we will see how to deploy the SPFx field customizer to SharePoint Online.
Now, we need to bundle our solution to do this in the VS code terminal and run the following command:
gulp bundle
Then, enter the below command to package the extension that we created :
gulp package-solution
This command creates a package where we need to put our extension store in the SharePoint site app catalog.
To see this file, go to sharepoint/solution/performance-score-customizer.sppkg in the VS Code.
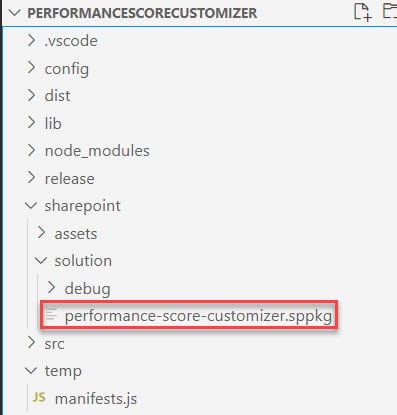
Now, I want to deploy the field customizer extension in the Site Collection App Catalog, not the Tenant App Catalog.
Note: You can upload the SPFx web part to the organizational app catalog site if you want to deploy it there.
1. To enable the Site Collection App Catalog, use the following PowerShell script:
Connect-SPOService -Url https://<tenant>-admin.sharepoint.com/ -Credential MiriamG@<tenant>.onmicrosoft.com
$site = Get-SPOSite https://<tenant>.sharepoint.com/sites/SPFx
Add-SPOSiteCollectionAppCatalog -Site $site

If you want to disable the Site Collection App Catalog, use the script below:
Remove-SPOSiteCollectionAppCatalog -Site $site
2. When you navigate to the Site contents where you enabled the Site Collection App Catalog, you’ll see a new list called “Apps for SharePoint.” Here, you need to deploy your web part package.
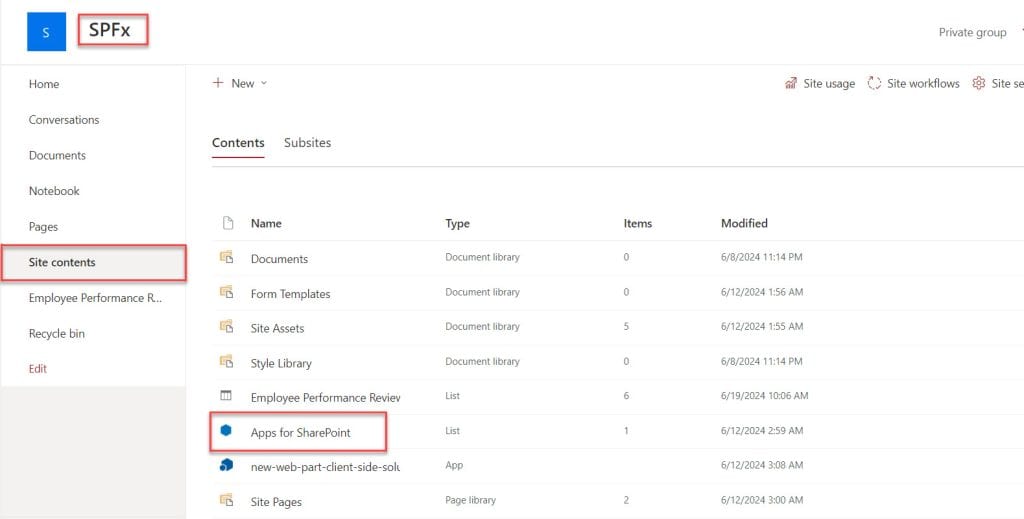
3. Open the Apps for SharePoint list and upload or drag and drop the .sppkg file.
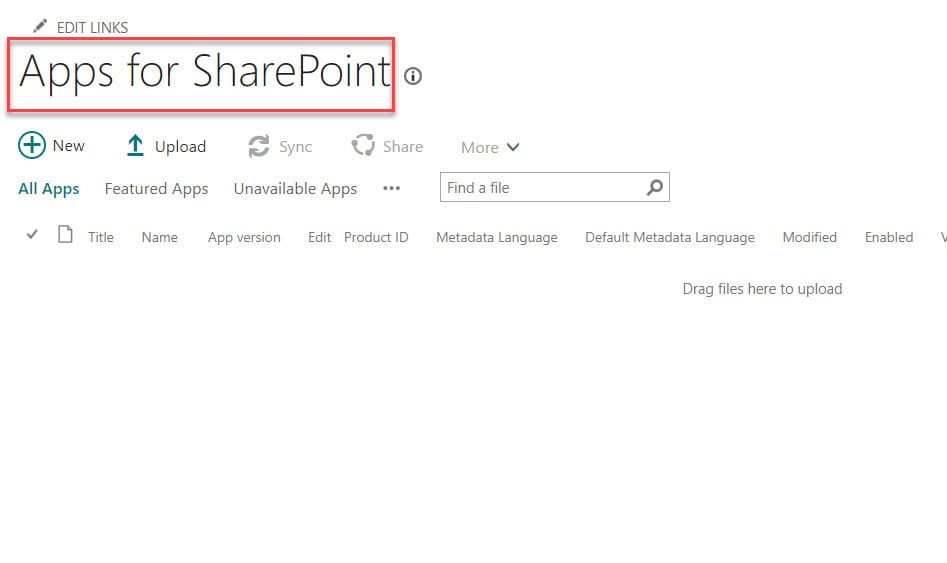
4. Then, a popup window will open, where you need to click “Deploy.” You can check the checkbox to show the extension to all sites in the organization.
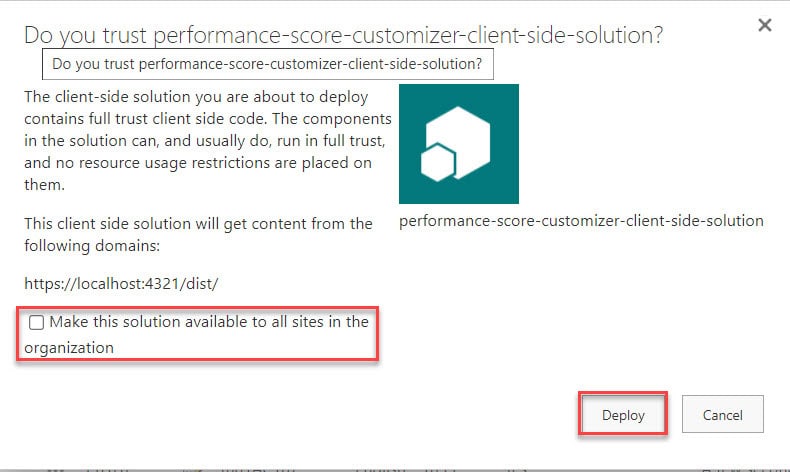
5. Go to the Home page, click +New, then select App.
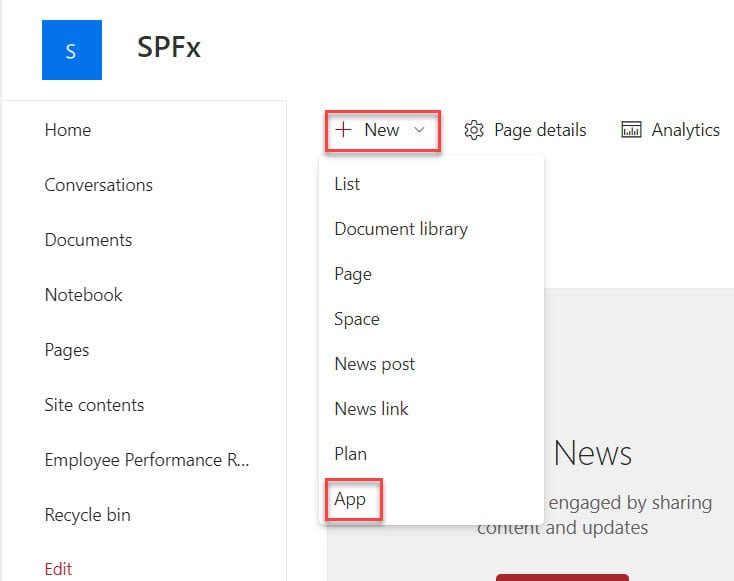
6. To add the SharePoint Framework field customizer extension to the SharePoint site, click the “Add” button.
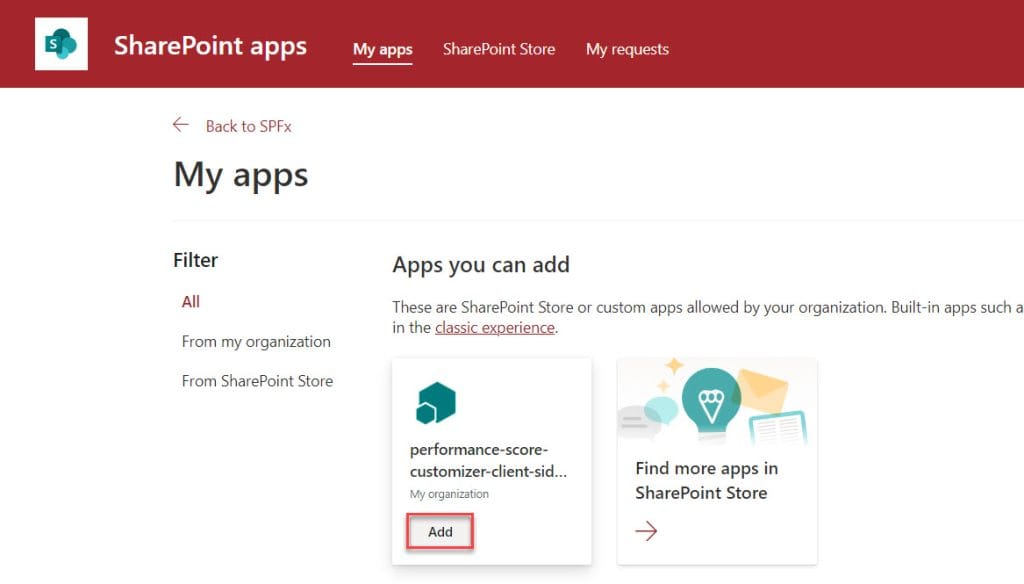
7. When you revisit the Site Contents, you’ll find the extension app listed there.
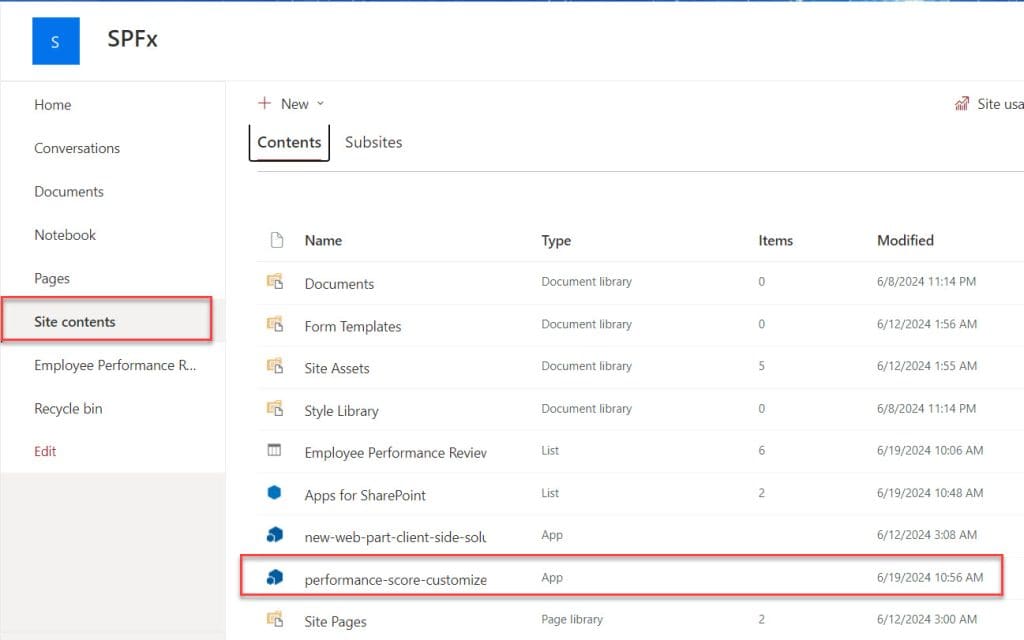
8. Now, create a SharePoint list and go to the list settings. Then click ‘Add from existing site columns.’ Select site columns from ‘SPFx Columns‘ and add the ‘PerformanceScore‘ column. Remember the two things I mentioned earlier: DisplayName and Group, which you’ll find in the elements.xml file.
After that, click ok.
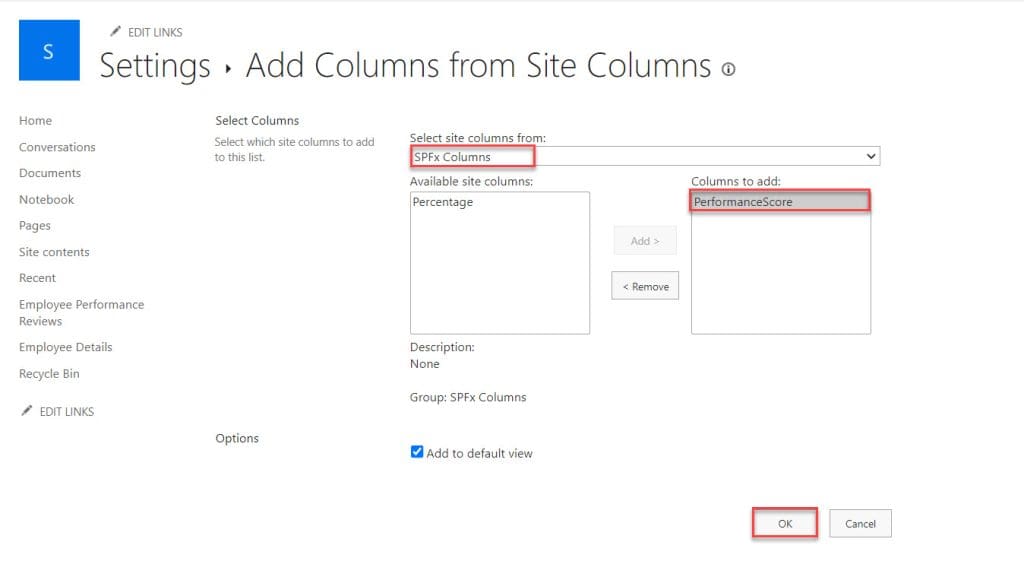
9. You can see the changes when you go to that SharePoint list and add some value in the PerformanceScore column.
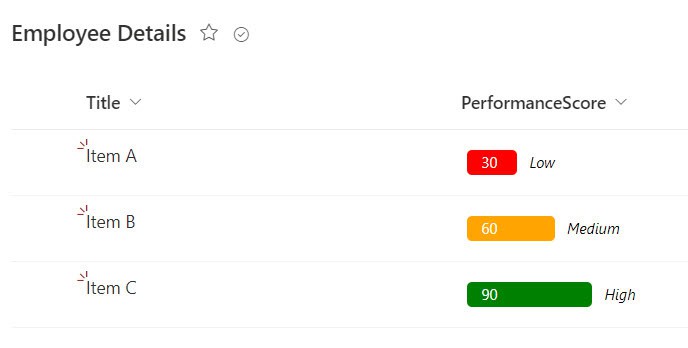
I hope now you can work with the SPFx field customizers in SharePoint Online. Here, I have explained how to create a field customizer using SPFx and deploy it to a SharePoint site collection.
You may also like:
- SPFx Property Pane Controls
- SPFx fluent UI react dropdown example
- SharePoint Framework CRUD Operations using React
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com
Very good. Thank you teacher.